Summary:
There's a crash-inducing bug with `Image.blurRadius` on Android.
`blurRadius` is specified in JavaScript as a `float`, but it's cast to `int` before being passed to the `IterativeBoxBlurPostProcessor`. However, in `IterativeBoxBlurPostProcessor`, there is an argument precondition requiring the integer `blurRadius` to be non-zero.
Because the `== 0` condition is evaluated on the `float`, it's possible for a `blurRadius` in the range of `(0, 1)` (non-inclusive) to pass the conditional, and then be truncated to `0` and passed as an argument to `IterativeBoxBlurPostProcessor`, which will fail its precondition and crash the app.
This change works in our app, which was previously crashing.
[ANDROID] [BUGFIX] [Image] Fixed crash when specifying an Image.blurRadius between (0, 1)
Closes https://github.com/facebook/react-native/pull/16845
Differential Revision: D6387416
Pulled By: shergin
fbshipit-source-id: d5191aa97e949ffd41e6d68c96b3c7bcbc82a52e
Summary:
`Canvas.clipPath` isn't supported with hardware acceleration in APIs below 18. The rounded border rendering logic for Android relies on this method. Therefore, rounded borders do not render correctly on such devices.
**Screenshot of Nexus 5 running API 17 (Before these changes):**
https://pxl.cl/9rsf
**The fix**: If the API version is less than 18 and we're rendering rounded borders, I disable hardware acceleration. Otherwise, I enable it. I'm going to check to see if this has perf regressions by running a CT-Scan.
With this change, rounded borders render correctly on Android devices running versions of Android between Honeycomb to JellyBean MR2.
**Screenshot of Nexus 5 running API 17 (After these changes):**
https://pxl.cl/9rrk
Reviewed By: xiphirx
Differential Revision: D6153087
fbshipit-source-id: 16e35be096051ac817c8b8bcdd132ecff3b4b167
Summary:
React Native 0.43 added additional functionality to setSelectionColor that also tints the cursor drawable of the View. However, some views may not have a cursor drawable set in which case, the code will crash when attempting to load a drawable with resource id 0.
We encountered this in our RN 0.45 upgrade in the Airbnb app.
lelandrichardson
Closes https://github.com/facebook/react-native/pull/14789
Differential Revision: D6386076
Pulled By: shergin
fbshipit-source-id: faa5a1edb3be8d08988f46205c0f22d17b63b5bc
Summary:
Previously, only form-data request bodies emitted upload progress updates. Now,
other request body types will also emit updates.
Addresses issues:
https://github.com/facebook/react-native/issues/15724https://github.com/facebook/react-native/issues/11853
This is a bug fix for functionality that's missing on Android. These events are already working correctly on iOS.
Run the following code on Android, and ensure that events are being sent:
```
const fileUri = 'file:///my_file.dat';
const url = 'http://my_post_url.com/';
const xhr = new XMLHttpRequest();
xhr.upload.onprogress = (event) => {
console.log('progress: ' + event.loaded + ' / ' + event.total);
}
xhr.onreadystatechange = () => {if (xhr.readyState === 4) console.log('done');}
console.log('start');
xhr.open('POST', url);
xhr.send({ uri: fileUri }); // sending a file (wasn't sending progress)
xhr.send("some big string"); // sending a string (wasn't sending progress)
const formData = new FormData(); formData.set('test', 'data');
xhr.send(formData); // sending form data (was already working)
```
[ANDROID] [BUGFIX] [XMLHttpRequest] - Added progress updates for all XMLHttpRequest upload types
Previously, only form-data request bodies emitted upload progress updates. Now,
other request body types will also emit updates.
Addresses issues:
https://github.com/facebook/react-native/issues/15724https://github.com/facebook/react-native/issues/11853
Closes https://github.com/facebook/react-native/pull/16541
Differential Revision: D6325252
Pulled By: hramos
fbshipit-source-id: 4fe617216293e6f451e2a1af4fa872e8f56d4f93
Summary:
We've seen cases (based on logs) where NetInfo is reporting no connectivity, but network requests still work. This will keep status up to date after app foreground <-> backgrounds, since we don't listen to broadcasts when backgrounded.
This is rather difficult to test given we haven't nailed an internal repro (evidence is solely based on device/app logs). Testing has been done to ensure that there are no behavioural changes on devices that were previously working (no regressions).
Closes https://github.com/facebook/react-native/pull/15558
Differential Revision: D6264708
Pulled By: hramos
fbshipit-source-id: 1648cadb59949103d0a595614b38024ec9236719
Summary:
update() is called from the choreographer, so it can be
invoked asynchronously relative to RN. If it's called while the node
tree is incomplete, this can be called with no parent. Don't treat an
unparented node as an invariant failure, just skip over it.
Reviewed By: AaaChiuuu
Differential Revision: D6249038
fbshipit-source-id: d22807dff1659bf29a81893ab97d0fe7c19de512
Summary:
We noticed that on Android the lineHeight behaviour is different from iOS for built in fonts and custom fonts. The problem becomes visible when the lineHeight approaches the fontSize, showing a cut-off on the bottom of the TextView. This issue has been raised before in #10712. There is a mention of a PR with a fix in that issue, which has not been merged yet. This implementation is a less intrusive fix leaving the current lineHeight approach in place and fixing the discrepancy only.
This proposed change prioritises ascent over descent for reduction, making the lineHeight functionality behave identical to iOS.
There is no existing test covering the lineHeight property and its behaviour in the CustomLineHeightSpan. This PR contains new unit tests that covers the various scenario's for the lineHeight calculations.
The original behaviour, before the change can against these unit tests. The case that fails is `shouldReduceAscentThird`, which can be made to succeed on the old code by changing the asserts to:
```
assertThat(fm.top).isEqualTo(-5);
assertThat(fm.ascent).isEqualTo(-5);
assertThat(fm.descent).isEqualTo(-4);
assertThat(fm.bottom).isEqualTo(-4);
```
The unit test succeeds for the current implementation, which has the values for ascent and descent inverted.
Below screenshots show before, after and iOS:
BEFORE
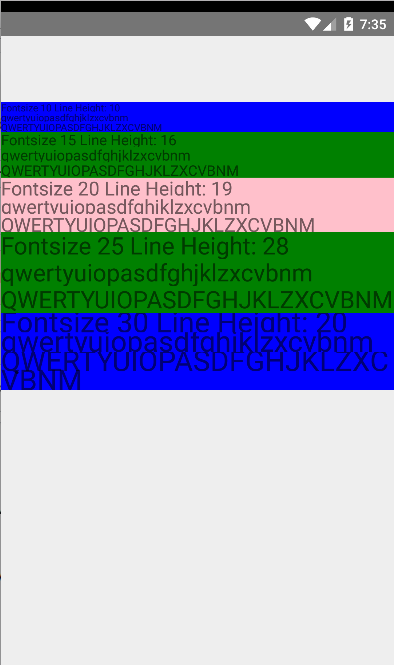
AFTER
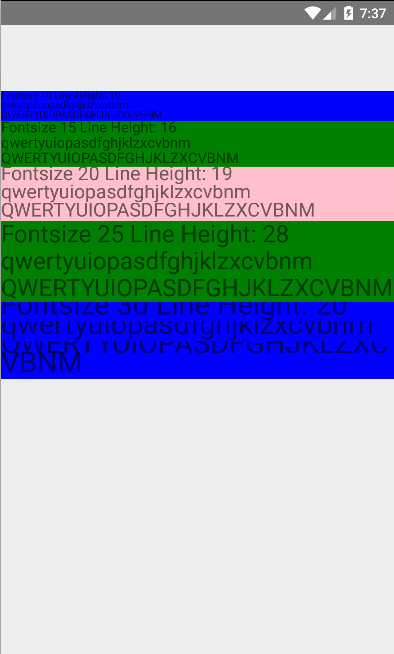
iOS
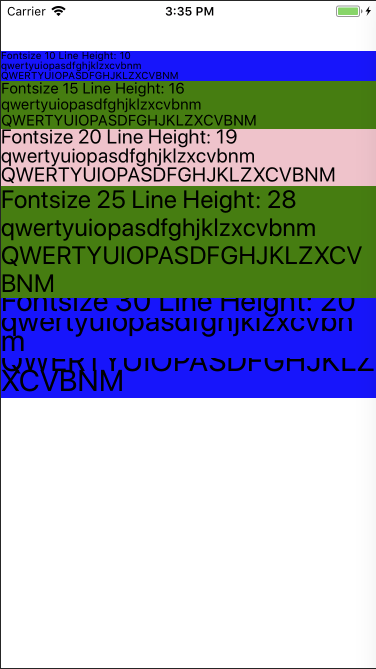
[ANDROID] [BUGFIX] [Text] - Fix the lineHeight behaviour on Android to match iOS
Closes https://github.com/facebook/react-native/pull/16448
Differential Revision: D6221854
Pulled By: andreicoman11
fbshipit-source-id: 7292f0f05f212d79678ac9d73e8a46bf93f1a7c6
Summary:
Reported in Issue #15928
Fixing bug when permission showing dialog and user go to home and re-open minimized app.
1. Ask for permission
2. Minimize app (Press HomeButton)
3. Open app again via shortcut
[ANDROID] [BUGFIX] [PermissionsModule.java] - Fixed bug when asked for permission Cause: java.lang.ArrayIndexOutOfBoundsException · length=0; index=0
Closes https://github.com/facebook/react-native/pull/16507
Differential Revision: D6133708
Pulled By: shergin
fbshipit-source-id: 9c05d1d5d16fedf718ad5113df69a4df3af62013
Summary:
Rebased version of #12842 that was reverted because of failing fb internal tests.
Closes https://github.com/facebook/react-native/pull/15919
Differential Revision: D5823956
Pulled By: hramos
fbshipit-source-id: 4ece19a403f5ebbe4829c4c26696ea0575ab1d0e
Summary:
Trivial.
That's okay that sometimes shadowNodes and views hierarchies have lack of synchonization.
Reviewed By: sahrens
Differential Revision: D6040022
fbshipit-source-id: 6b49a82317b620b66a87441719fddcafb1f27934
Summary:
`visible-password` represents a very basic keyboard, typically only
letters and numbers. Backed by InputType.TYPE_TEXT_VARIATION_VISIBLE_PASSWORD,
it is useful for things like password and code entry fields. It can also be more
effective than autoCorrect={false} for disabling autocompletion on some keyboards
(like Gboard).
Note `secureTextEntry` also affects `TYPE_TEXT_VARIATION_*` flags internally, so there
may be some undefined behavior when combining `secureTextEntry` with
`keyboardType="visible-password"`
Also, while here, improve the documentation on TextInput to explicitly enumerate
which keyboardType applies to Android vs. iOS (since this is the first android-specific)
Reviewed By: shergin
Differential Revision: D6005353
fbshipit-source-id: 13af90c96353f714c0e106dd0fde90184a476533
Summary:
FIX#11142
This fixes#11142 and supersedes #11155 as I was unsure how to add/change commits in that PR.
Wrote up a bunch of unit tests for the ImageStore module. The added tests showed that there was indeed a problem with the flags used for the Base64OutputStream, and they also show that that has been fixed now.
Closes https://github.com/facebook/react-native/pull/13856
Differential Revision: D6017764
Pulled By: shergin
fbshipit-source-id: adf667dc722ddfe31449afd8cd20a0a192eacff6
Summary:
CI is currently failing because of a lint issue, this fixes it and a bunch of other warnings that are auto-fixable.
**Test plan**
Quick manual test, cosmetic changes only.
Closes https://github.com/facebook/react-native/pull/16229
Differential Revision: D6009748
Pulled By: TheSavior
fbshipit-source-id: cabd44fed99dd90bd0b35626492719c139c89f34
Summary:
Motivation:
(SUDDENLY) There is a thing on Android called SpanWather, and their purpose is to notify "the watcher" about span-related changes in SpannableString. The idea is: some special kind of span can have some logic to prevent or tweak interleaving with some another kind of spans. To do so, it has to implement SpanWather interface.
So, EditText uses this to control internal spannable object (!) and SUDDENLY (#><) calls internal "layout" method as a reaction to adding new spans. So, when we are cloning SpannableString, we are (re)applying same span objects to a new spannable instance, and it causes notifying other spans in the string, and they notify EditText, and the EditText does relayout and... BOOM!
So, the solution is, easy, we should use SpannableStringBuilder instead of SpannableString because it does not notify SpanWather during cloning.
See:
https://android.googlesource.com/platform/frameworks/base/+/master/core/java/android/text/SpannableStringBuilder.java#101
(the first argument is `false`).
https://android.googlesource.com/platform/frameworks/base/+/master/core/java/android/text/SpannableStringBuilder.java#678
Compare with:
https://android.googlesource.com/platform/frameworks/base/+/master/core/java/android/text/SpannableStringInternal.java#43
Why? I believe because SpannableStringBuilder objects are "unfinished" by design, and documentation said: "it is the caller's responsibility to restore invariants [among spans]". As we do an exact clone of the string, that's perfectly okay to assume that all invariants were already satisfied for original string.
Reviewed By: achen1
Differential Revision: D5970940
fbshipit-source-id: 590ca0e3aede4470b809c7db527c5d55ddf5edb4
Summary:
After this diff the intrinsic content size of <TextInput> reflects the size of text inside EditText,
it means that if there is no additional style constraints, <TextInput> will grow with containing text.
If you want to constraint minimum or maximum height, just do it via Yoga styling.
Reviewed By: achen1
Differential Revision: D5828366
fbshipit-source-id: eccd0cb4ccf724c7096c947332a64a0a1e402673
Summary:
In some cases we need a way to provide some peice of data to shadow node
to improve layout (or do something similar), `setLocalData` allows to do this.
Reviewed By: AaaChiuuu
Differential Revision: D5828368
fbshipit-source-id: bf6a04f460dc7695a16269426d365b78909bc8eb
Summary:
Basic implementation of the proposal in #15271
Note that this should not affect facebook internally since they are not using OSS releases.
Points to consider:
- How strict should the version match be, right now I just match exact versions.
- Wasn't able to use haste for ReactNativeVersion because I was getting duplicate module provider caused by the template file in scripts/versiontemplates. I tried adding the scripts folder to modulePathIgnorePatterns in package.json but that didn't help.
- Redscreen vs. warning, I think warning is useless because if the app crashes you won't have time to see the warning.
- Should the check and native modules be __DEV__ only?
**Test plan**
Tested that it works when version match and that it redscreens when versions don't before getting other errors on Android and iOS.
Closes https://github.com/facebook/react-native/pull/15518
Differential Revision: D5813551
Pulled By: hramos
fbshipit-source-id: 901757e25724b0f22bf39de172b56309d0dd5a95
Summary:
The Android ImageEditingManager is inefficient and slow when cropping images. It loads the full resolution image into memory and then crops it. This leads to slow performance and occasional OutOfMemory Exceptions.
[BitmapRegionDecoder](https://developer.android.com/reference/android/graphics/BitmapRegionDecoder.html) can be used to crop without needing to load the full resolution image into memory. Using it is much more efficient and much faster.
Relevant issue: https://github.com/facebook/react-native/issues/10470
Attempt to crop a very large image (2000x2000) on Android. With this change, the crop should happen almost instantly. On the master branch, it should take 2-3 seconds (and might run out of memory).
Please let me know if there's anything else I can provide.
Closes https://github.com/facebook/react-native/pull/15439
Differential Revision: D5628223
Pulled By: shergin
fbshipit-source-id: bf314e76134cd015380968ec4533225e724c4b26
Summary:
Instead of preventing events from working when not on the UI Thread we can just dispatch to it instead.
**Test plan**
Tested manually that animated events still work in RNTester
Closes https://github.com/facebook/react-native/pull/15953
Differential Revision: D5909816
Pulled By: shergin
fbshipit-source-id: 48d02b6aa9f2bc3bcb638e8852fccaac3f205276
Summary:
This problem tries to solve issue #14244
Implemented a fetch request to a TLSv1 server. It now successfully resolves TLS handshake.
Tested same fetch to TLSv1.2 server and still successfully resolves TLS handshake.
Closes https://github.com/facebook/react-native/pull/14245
Differential Revision: D5898689
Pulled By: shergin
fbshipit-source-id: 8766ebe6909443367651ab868aa5ff62747cd906
Summary:
Following the migration guide. Let's see what happens here.
Closes https://github.com/facebook/react-native/pull/14955
Differential Revision: D5877682
Pulled By: hramos
fbshipit-source-id: 2a40560120b5d8d28bc6c52cc5e5916fd1bba336
Summary:
As I was working on mimicking iOS animations for my ongoing work with `react-navigation`, one task I had was to match the "push from right" animation that is common in UINavigationController.
I was able to grab the exact animation values for this animation with some LLDB magic, and found that the screen is animated using a `CASpringAnimation` with the parameters:
- stiffness: 1000
- damping: 500
- mass: 3
After spending a considerable amount of time attempting to replicate the spring created with these values by CASpringAnimation by specifying values for tension and friction in the current `Animated.spring` implementation, I was unable to come up with mathematically equivalent values that could replicate the spring _exactly_.
After doing some research, I ended up disassembling the QuartzCore framework, reading the assembly, and determined that Apple's implementation of `CASpringAnimation` does not use an integrated, numerical animation model as we do in Animated.spring, but instead solved for the closed form of the equations that govern damped harmonic oscillation (the differential equations themselves are [here](https://en.wikipedia.org/wiki/Harmonic_oscillator#Damped_harmonic_oscillator), and a paper describing the math to arrive at the closed-form solution to the second-order ODE that describes the DHO is [here](http://planetmath.org/sites/default/files/texpdf/39745.pdf)).
Though we can get the currently implemented RK4 integration close by tweaking some values, it is, the current model is at it's core, an approximation. It seemed that if I wanted to implement the `CASpringAnimation` behavior _exactly_, I needed to implement the analytical model (as is implemented in `CASpringAnimation`) in `Animated`.
We add three new optional parameters to `Animated.spring` (to both the JS and native implementations):
- `stiffness`, a value describing the spring's stiffness coefficient
- `damping`, a value defining how the spring's motion should be damped due to the forces of friction (technically called the _viscous damping coefficient_).
- `mass`, a value describing the mass of the object attached to the end of the simulated spring
Just like if a developer were to specify `bounciness`/`speed` and `tension`/`friction` in the same config, specifying any of these new parameters while also specifying the aforementioned config values will cause an error to be thrown.
~Defaults for `Animated.spring` across all three implementations (JS/iOS/Android) stay the same, so this is intended to be *a non-breaking change*.~
~If `stiffness`, `damping`, or `mass` are provided in the config, we switch to animating the spring with the new damped harmonic oscillator model (`DHO` as described in the code).~
We replace the old RK4 integration implementation with our new analytic implementation. Tension/friction nicely correspond directly to stiffness/damping with the mass of the spring locked at 1. This is intended to be *a non-breaking change*, but there may be very slight differences in people's springs (maybe not even noticeable to the naked eye), given the fact that this implementation is more accurate.
The DHO animation algorithm will calculate the _position_ of the spring at time _t_ explicitly and in an analytical fashion, and use this calculation to update the animation's value. It will also analytically calculate the velocity at time _t_, so as to allow animated value tracking to continue to work as expected.
Also, docs have been updated to cover the new configuration options (and also I added docs for Animated configuration options that were missing, such as `restDisplacementThreshold`, etc).
Run tests. Run "Animated Gratuitous App" and "NativeAnimation" example in RNTester.
Closes https://github.com/facebook/react-native/pull/15322
Differential Revision: D5794791
Pulled By: hramos
fbshipit-source-id: 58ed9e134a097e321c85c417a142576f6a8952f8
Summary:
Fixes https://github.com/facebook/react-native/issues/15863 on master. Behavior of `onSubmitEditing` is now consistent between iOS and Android. Tapping the submit button in a TextInput dispatches the event precisely when doing so does not make a newline (when blurOnSubmit is true or multiline is false).
1. Run this app on iOS and Android:
```
// flow
import React, { Component } from 'react';
import {
StyleSheet,
TextInput,
View
} from 'react-native';
type State = {
toggled: boolean
};
type Props = {
blurOnSubmit: boolean,
multiline: boolean
};
class ToggleColorInput extends Component<Props, State> {
state: State = {
toggled: false
};
props: Props;
toggle = () => {
this.setState({
toggled: !this.state.toggled
});
}
render() {
return (
<TextInput
blurOnSubmit={this.props.blurOnSubmit}
multiline={this.props.multiline}
onSubmitEditing={this.toggle}
style={[styles.textInput, {backgroundColor: this.state.toggled ? 'blue' : 'azure'}]}
underlineColorAndroid='transparent'
/>
)
}
}
export default class App extends Component<{}> {
render() {
return (
<View>
<ToggleColorInput blurOnSubmit={true} multiline={true} />
<ToggleColorInput blurOnSubmit={true} multiline={false} />
<ToggleColorInput blurOnSubmit={false} multiline={true} />
<ToggleColorInput blurOnSubmit={false} multiline={false} />
</View>
);
}
}
const styles = StyleSheet.create({
textInput: {
height: 75,
borderWidth: 1,
borderColor: 'black'
}
});
```
2. You see four TextInputs, with each combination of the `blurOnSubmit` and `multiline` properties. For each TextInput, type some text and tap the submit button.
3. The TextInputs in this test will toggle background color when they emit an `onSubmitEditing` event. Verify the following behavior on each platform:
* blurOnSubmit && isMultiline => Submit event emitted, blurred, no newline inserted
* blurOnSubmit && !isMultiline => Submit event emitted, blurred
* !blurOnSubmit && isMultiline => Submit event emitted, newline inserted
* !blurOnSubmit && !isMultiline => Submit event emitted
Closes https://github.com/facebook/react-native/pull/16040
Differential Revision: D5877401
Pulled By: shergin
fbshipit-source-id: 741bcc06d8b69d7025f2cb42dd0bee4fa01cd88e
Summary:
<!--
Thank you for sending the PR! We appreciate you spending the time to work on these changes.
Help us understand your motivation by explaining why you decided to make this change.
You can learn more about contributing to React Native here: http://facebook.github.io/react-native/docs/contributing.html
Happy contributing!
-->
Headless tasks are required to run in the main thread, however due to the nature of the React context creation flow, the handler may be returned outside of the main thread, causing the HeadlessJsTaskContext to throw an exception.
Swipe out the app. send push notification from a server that starts a HeadlessJsTaskService
Closes https://github.com/facebook/react-native/pull/15940
Differential Revision: D5852448
Pulled By: foghina
fbshipit-source-id: 54c58a1eb7434dd5de5c39c28f6e068ed15ead9d
Summary:
When we convert nested <Text> components to Spannable object we must enforce the order of spans somehow,
otherwise we will have Spannable object with unpredictable order of spans, which will produce unpredictalbe text layout.
We can do it only using `Spannable.SPAN_PRIORITY` feature because Spannable objects do not maintain the order of spans internally.
We also have to fix this to implement autoexpandable <TextInput>.
Reviewed By: achen1
Differential Revision: D5811172
fbshipit-source-id: 5bc68b869e58aba27d6986581af9fe3343d116a7
Summary:
**Problem:**
It was observed that in [this code path](https://github.com/facebook/react-native/blob/master/ReactAndroid/src/main/java/com/facebook/react/views/scroll/ReactHorizontalScrollView.java#L292) (i.e. horizontal, paging-enabled scroll view) if you tried to programmatically call the scrollTo method within ~1s of the onMomentumScrollEnd event (which should only be called after all scrolling has ended), the scrollView would scroll to the new location, and then scroll BACK to the original location.
For example, assume you had released the scrollView at location B, and the nearest page boundary is A. Then, 1000ms later, you call scrollTo position C. The order of operations would be:
1. Begin scrolling to A from position B (as it is the nearest page boundary)
2. Reach position A
3. Fire onMomentumScrollEnd
4. 1000ms later call scrollTo C
5. scrollView scrolls to C
6. scrollView scrolls BACK to position A (for no apparent reason).
**Reason:**
I suspect this is because the [smoothlyScrollTo](https://github.com/facebook/react-native/blob/master/ReactAndroid/src/main/java/com/facebook/react/views/scroll/ReactHorizontalScrollView.java#L292) will continue to animate towards A, but the [scrollEvents will not fire](f954f3d9b6/ReactAndroid/src/main/java/com/facebook/react/views/scroll/OnScrollDispatchHelper.java (L45)) as they are too close to each other. So the true order of events is:
1. Begin scrolling to A from position B (as it is the nearest page boundary)
[begin smoothlyScrollTo]
[scroll towards position A]
[mActivelyScrolling is true]
2. Reach position A
[mActivelyScrolling is true]
[scroll towards position A]
[mActivelyScrolling is false, as there is another scrollEvent, but because it is close enough to the same location it is ignored]
3. Fire onMomentumScrollEnd
4. 1000ms later call scrollTo C
[scroll towards position C]
5. scrollView scrolls to C
[scroll towards position A as the original smoothlyScrollTo animation was never completed]
6. scrollView scrolls BACK to position A.
This is an untested hypothesis, but seems to explain the behavior, and the solution is more semantically correct anyway. If there is an easy way to rebuild the android binaries happy to test it myself! Just let me know!
**Solution:**
Move the mActivelyAnimating outside the mOnScrollDispatchHelper.onScrollChanged helper, because the HorizontalScrollView event should be considered to be animating so long as onScrollChanged events are being fired.
Closes https://github.com/facebook/react-native/pull/15146
Reviewed By: AaaChiuuu
Differential Revision: D5792026
Pulled By: tomasreimers
fbshipit-source-id: 9654fda038d4a687cc32f4c32dc312baa34627ed
Summary:
Abstract class `ReactBaseTextShadowNode` was decoupled from `ReactTextShadowNode` to separate two goals/roles:
* `ReactBaseTextShadowNode` represents spanned `<Text>` nodes, which can bear text attributes (both `RCTText` and `RCTVirtualText`);
* `ReactTextShadowNode` represents anchor `<Text>` view in Yoga terms, which can bear layout attributes (`RCTText` and `RCTTextInput`).
`ReactVirtualTextShadowNode` now inherits `ReactBaseTextShadowNode`.
The same architectural changes was applited to view managers.
Why?
* This is just a better architecture which represents the nature of this objects.
* Bunch of "negative" logic which turn off excessive features for some suclasses was removed.
* Memory efficiency.
* Now we can improve `<TextInput>` component using right inheritance. Yay!
Reviewed By: achen1
Differential Revision: D5715830
fbshipit-source-id: ecc0764a03b5b7586fe77ad31f149cd840f4da41
Summary:
ReactRawTextShadowNode represents "raw" text node (aka textContent), so it cannot have layout or styling, it is just a line of text, a pure string.
So, we must not interit it from HUGE ReactTextShadowNode (which represents <Text> node with ReactRawTextShadowNode instance inside).
We need this change to make future fancy (and valuable from product perspective) refactoring possible. Stay tuned!
Reviewed By: achen1
Differential Revision: D5712961
fbshipit-source-id: 009e48046bdf34ddfd40b93175934969af64b98b
Summary:
We have to have a way to explicitly enforce the fact that some nodes cannot have Yoga child nodes.
Previously we relied on `isMeasureDefined()`, which is actually special case (so it does not cover all possible cases).
Reviewed By: AaaChiuuu
Differential Revision: D5647855
fbshipit-source-id: 59591be61ef62c61eb98748d44bb28b878f713fc
Summary:
This code related to velocity would cause some scroll events to be skipped and caused some jitter for sticky headers. Not sure if there is a better fix but removing this does fix missing events and the velocity calculation still seems to be working.
**Test plan**
Tested that sticky headers now work properly on Android, tested that velocity calculation still seem to work.
Closes https://github.com/facebook/react-native/pull/15761
Differential Revision: D5760820
Pulled By: sahrens
fbshipit-source-id: 562b5f606bdc5452ca3d85efb5e0e3e7db224891
Summary:
…ndroid
Android overrides the original set ime option when the EditText is a multiline one.
This change makes sure to set it back to the original one when blurOnSubmit is true,
which causes the button icon to be conforming to the set returnKeyType as well as
changing the behaviour of the button, such that it will blurOnSubmit correctly.
The reason not do it with blurOnSubmit being false is
because it then would not create new lines when pressing the submit button,
which would be inconsistent with IOS behaviour.
**Note** this change relies on this one #11006 because the app would crash if we don't expllicitly remove the focus (`editText.clearFocus();`)
Fixes#8778
**Test plan (required)**
1. Create view with TextInput with multiline and blurOnSubmit set to true
```javascript
<View>
<TextInput
returnKeyType='search'
blurOnSubmit={true}
multiline={true}
onSubmitEditing={event => console.log('submit search')}></TextInput>
</View>
```
2. Input some text and click submit button in soft keyboard
3. See submit event fired and focus cleared / keyboard removed
Closes https://github.com/facebook/react-native/pull/11125
Differential Revision: D5718755
Pulled By: hramos
fbshipit-source-id: c403d61a8a879c04c3defb40ad0b6689a2329ce1
Summary:
Fixes#12591
The Android JSTouchDispatcher was using `mTargetCoordinates` when creating the TouchEvent for a move. However, these are final values which are set when the touch down is received. When the user's finger moves, it's important to be able to track the coordinates of the touch as it moves. Thus, we need to update the x,y coordinates by calling `TouchTargetHelper` on each move event.
Closes https://github.com/facebook/react-native/pull/15123
Reviewed By: achen1
Differential Revision: D5476663
Pulled By: shergin
fbshipit-source-id: ce79e96490f3657a13f9114fcc93e80d5fdbebaf
Summary:
<!--
Thank you for sending the PR! We appreciate you spending the time to work on these changes.
Help us understand your motivation by explaining why you decided to make this change.
You can learn more about contributing to React Native here: http://facebook.github.io/react-native/docs/contributing.html
Happy contributing!
-->
I was trying to typeset mathematical equations in react native. When typesetting fractions, baseline of the fraction must be aligned with the baseline of others. The baseline of fraction will vary based on size of numerator and denominator.
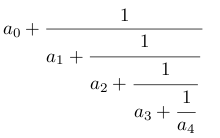
In yoga, we can set custom baseline function using `YogaNode.setBaselineFunction` method. If this method is exposed in `ReactShadowNode` class, it will be easy to create custom native UI modules which requires setting custom baseline.
Closes https://github.com/facebook/react-native/pull/15605
Differential Revision: D5686876
Pulled By: shergin
fbshipit-source-id: 34d797a7ea27d5c1b9f6b9c36e469cdca3883aec
Summary:
Currently, `onLoadStart` fires for a couple of cases:
1. toplevel page loads (e.g. initial page load, clicking links)
2. loading of pages within an iframe
The fact that `onLoadStart` fires for case (2) causes some problems. For example, it makes it difficult for the code that uses the WebView to know what URL the WebView is currently rendering. This is because the listener can't distinguish between the toplevel URL and the URL of an iframe. Additionally, this behavior is inconsistent with the behavior on iOS. On iOS, `onLoadStart` only fires for toplevel page loads.
To fix these issues, this change deletes the `doUpdateVisitedHistory` handler so that `onLoadStart` only fires for case (1).
**Test Plan**
Created a test page that has an iframe and loaded it in the WebView. Verified that `onLoadStart` only fires for toplevel page loads.
Adam Comella
Microsoft Corp.
Closes https://github.com/facebook/react-native/pull/15554
Differential Revision: D5665979
Pulled By: hramos
fbshipit-source-id: a52e473bc5691a6e180f45f0728e4ad89a7d354f
Summary:
Overview -
This PR resolves the issue described in #14606. This PR makes Text components take into account the includeFontPadding property when calculating their size.
Background -
Currently, on Android, when includeFontPadding is set to false, the React Text component does not adjust its height. This makes it difficult to lay out other components at a precise spacing relative to a Text component.
iOS calculates the height of a UILabel based on the font's descent + ascent.
Android lets you choose whether to calculate the height of a TextView based on the font's top + bottom (includeFontPadding=true) or ascent + descent (includeFontPadding=false).
In order for a text component to be the same size on iOS and Android (relative to the rest of the layout in points and dips), one should set includeFontPadding=false on Android - but the React Text component needs to take this property into account when sizing itself for this to work.
Please see this stack overflow post for a visual explanation of the difference between a font's ascent/descent and top/bottom - https://stackoverflow.com/questions/27631736/meaning-of-top-ascent-baseline-descent-bottom-and-leading-in-androids-font
Testing -
Please see the attached screenshots to see the height difference of a Text component with this PR when includeFontPadding is true vs false.
The font I am using has an ascent + descent = em-size so that the height of the Text component will be equal to the font-size for a single line of text. This is to clearly show the additional height that includeFontPadding=true adds to the Text component.
For Text components that are styled in the same way,
When includeFontPadding=true, height = ~29.714 dips
When includeFontPadding=false, height= 24 dips
<img width="342" alt="includefontpaddingtrue" src="https://user-images.githubusercontent.com/1437344/27299391-3eec9de0-54fa-11e7-81d5-d0aeb40e8e27.png">
<img width="346" alt="includefontpaddingfalse" src="https://user-images.githubusercontent.com/1437344/27299401-45c95248-54fa-11e7-98d7-17dd152d3cb8.png">
Closes https://github.com/facebook/react-native/pull/14609
Reviewed By: AaaChiuuu
Differential Revision: D5587602
Pulled By: achen1
fbshipit-source-id: 6d2f12ba72ec7462676645519cd27820279278eb
Summary:
This is a workaround for missing PDF url support in Android WebView, which is a general known issue: when tapping a PDF url within WebView, instead of doing nothing, we just let android default intent handle it (e.g. it will open Chrome to load it).
This is basically to trick `shouldOverrideUrlLoading()` to return true for the specific url. The drawback is that product code needs to provide the whitelist.
The proper fix would be to use PdfRenderer in that method, but it seems like it's only for API >= 21...
Differential Revision: D5619383
fbshipit-source-id: f86b930f970dab9a5f57999df69ce94b9508edc9
Summary:
Since the `hot` parameter is not used anymore in metro-bundler (we are always applying the HMR transforms for bundlers requested through the HTTP server), we can remove it from the client request URL.
This allows us to reduce the metro-bundler memory by half when switching between HMR and not-HMR, since metro caches the bundles based on the requested URL path.
Reviewed By: davidaurelio
Differential Revision: D5630051
fbshipit-source-id: fb5dce4c31bbb38b1c0c93c97a525a992b2f6d8d
Summary:
We ran into a couple of problems with the implementation of `getCurrentPosition` on Android:
- It sometimes returns an inaccurate location
- It times out when `enableHighAccuracy` is `true` (#7495)
This change improves `getCurrentPosition` for both of the above problems. Instead of calling `requestSingleUpdate` it now calls `requestLocationUpdates` so it can receive multiple locations giving it an opportunity to pick a better one. Unlike `requestSingleUpdate`, this approach doesn't seem to timeout when `enableHighAccuracy` is `true`.
**Test plan (required)**
Verified in a test app that `getCurrentPosition` returns a good location and doesn't timeout when `enableHighAccuracy` is `true`. Also, my team has been using this change in our app in production.
Adam Comella
Microsoft Corp.
Closes https://github.com/facebook/react-native/pull/15094
Differential Revision: D5632100
Pulled By: hramos
fbshipit-source-id: 86e40b01d941a13820cb775bccad7e19dba3d692
Summary:
This is a simple groundwork PR to allow options to be passed to the `WebSocket` constructor. It represents a minor change to an undocumented part of the API, moving `headers` to within `options`.
This will be a BC for anyone manually specifying headers other than `origin` but a) that's not a common use case with WebSockets and b) it's not documented even in code and wouldn't currently pass a flow check.
NB: The third argument to the WebSocket constructor isn't part of the W3C spec, so I think this is a good place for RN-specific named parameters, better than adding a fourth argument. `protocols` needs to stay where it is, in line with the spec.
If this goes through I'd like to build on it by adding an additional connection option for SSL certificate pinning, as already supported by the underlying `okhttp` and `RCTSRWebSocket`. It could later be expanded for various other uses.
Currently, there's no way for a `WebSocket` user to specify any connection options other than url, protocol and headers. The fact that `WebSocket` connects in its constructor means any options have to go in there.
Connect to a websocket server using iOS and Android, observe the connection headers:
1. Without specifying `origin`, the default header should be set
2. Specifying it in the old way `new WebSocket(url, protocols, { origin: 'customorigin.com' })`
3. Specifying it in the new way `new WebSocket(url, protocols, { headers: { origin: 'customorigin.com' }})`.
I've tested myself using the test app with iOS and Android.
Closes https://github.com/facebook/react-native/pull/15334
Differential Revision: D5601675
Pulled By: javache
fbshipit-source-id: 5959d03a3e1d269b2c6775f3e0cf071ff08617bf
Summary:
LocalString doesn't have a check for null ptr which causes a crash if the null string passed.
Closes https://github.com/facebook/react-native/pull/15372
Differential Revision: D5601469
Pulled By: javache
fbshipit-source-id: a1b20efbae90009f0d465c077e6401a701d7515f
Summary:
If the z-index was updated after the initial mount, changes would not be reflected because we did not recalculate the z-index mapped child views and redraw the view. This adds code to do that and call it whenever we update z-index.
**Test plan**
Tested by reproducing the bug with 2 overlapping views that change z-index every second. Made sure it now works properly and z-index changes are reflected.
Closes https://github.com/facebook/react-native/pull/15203
Differential Revision: D5564832
Pulled By: achen1
fbshipit-source-id: 5b6c20147211ce0b7e8954d60f8614eafe128fb4
Summary:
My PR was pulled into RN 0.37 (d294e15c43). Since then an issue was discovered: ARTSurface skipped drawing the first render cycle if native TextureView takes too long. In case a static graphic is rendered in a single render cycle, it may be skipped resulting in an empty canvas being displayed.
A solution proposed in this PR: instead of skipping updates, make them pending and flush once the TextureView is ready.
This solution is released within our production app. It fixed ArtSurface initialisation issues cased by original PR to RN 0.37.
Closes https://github.com/facebook/react-native/pull/11539
Differential Revision: D4449255
Pulled By: shergin
fbshipit-source-id: a517909ca5c78c09a3ac8d9052664b92841b4e08
Summary:
This is the better fix for the same issue as mentioned in PR https://github.com/facebook/react-native/pull/14560
Certain rotateX, rotateY, scaleX and scaleY animations do not work correctly on some phones in Android 7.0.0, causing issues such as https://github.com/facebook/react-native/issues/14462 and https://github.com/facebook/react-native/issues/13522.
The issue can be fixed on JS side by setting an additional transform for perspective, eg. `{perspective: 1}` which triggers a `setCameraDistance` call in native code.
The fix in this PR always sets the camera distance on transforms, even when no perspective transform was specified. The default camera distance is set before the scale multiplication, to make sure that the value is appropriate for the phones density. The value calculates to an Android 'default' camera distance of 1280 * scale multiplier; https://developer.android.com/reference/android/view/View.html#setCameraDistance(float)
If a perspective transform is specified, this value will be used correctly still.
This fix was tested on the RNTester. Before the fix, on some devices, the FlatList example, with inverted turned on, will not display the list.
Devices that have been confirmed to have this issue:
FRD-AL10(honor 8) EMUI:5.0 android: 7.0
MHA-AL00(Mate9) EMUI:5.0 android:7.0
Huawei P10 VTR-L09, running Android 7.0
After the fix, the inverted FlatList displays correctly.
Closes https://github.com/facebook/react-native/pull/14646
Differential Revision: D5492009
Pulled By: shergin
fbshipit-source-id: d4da3b090a7e65df3b84e48ea32c964f4f8f7c88
Summary:
**Summary:**
There was a bug with RN.Dimensions returning incorrect window dimensions. In certain cases when device was in portrait, window dimensions reported landscape dimensions and vice versa.
This happened because in certain scenarios, after device orientation changed, dimensions update event from ReactRootView had incorrect dimensions.
Was able to reproduce this when device was rotated during app launch. After rotation global layout listener callback gets invoked. Inside the callback current and previous orientations are compared. When a change is detected, orientation and dimension change events are sent to JS. It is assumed, when orientation changes, new dimensions are available immediately. This is not the case for window dimensions as they are retrieved from resources object which gets updated asynchronously after orientation change. In cases when app is doing a lot of work on the main thread, like app startup, it takes more time to update the resources object. And when orientation change is detected in global layout, resources object is not updated with new dimensions yet. This causes dimensions update to be sent to JS with old window dimensions.
Global layout listener callback does get invoked a second time when resources object is finally updated with new dimensions, but since orientation no longer changes, no event is sent to JS.
Fixed this by separating dimensions update from orientation update. Now RN keeps track of previous window and screen dimension values. When a change is detected, an event is sent to JS with updated dimensions. This ensures that whenever dimensions change, JS gets the updated values.
This has a side effect of sending dimension update twice in some cases.
One example is the case above where window dimensions take time to update, but screen dimensions are updated immediately. This will cause two events to be sent to JS. One for window dimensions and one for screen dimensions update.
Other change is that initial value for both window and screen fields is empty. Which results in first change to trigger an event. Previously initial orientation value was 0 which meant when app started in normal portrait orientation, first layout did not trigger a dimension update event. Now even first layout sends the event. This should not be an issue as it is to make sure dimensions in JS side are correct.
**Testing:**
Verified with a sample app that correct dimensions are available when app launches.
Verified that after orientation dimensions are updated.
Verified that in the scenario described above where window dimensions are updated later, we get correct dimension values in JS.
We have incorporated this fix into our app and have been testing it internally.
Ats Jenk
Microsoft Corp.
<!--
Thank you for sending the PR!
If you changed any code, please provide us with clear instructions on how you verified your changes work. In other words, a test plan is *required*. Bonus points for screenshots and videos!
Please read the Contribution Guidelines at https://github.com/facebook/react-native/blob/master/CONTRIBUTING.md to learn more about contributing to React Native.
Happy contributing!
-->
Closes https://github.com/facebook/react-native/pull/15181
Differential Revision: D5552195
Pulled By: shergin
fbshipit-source-id: d1f190cb960090468886ff56cda58cac296745db
Summary:
Fixes the following crash:
```
Fatal Exception: java.lang.RuntimeException: Unable to destroy activity {com.example/com.example.MainActivity}: java.lang.NullPointerException: ImagePipelineFactory was not initialized!
at android.app.ActivityThread.performDestroyActivity(ActivityThread.java:3831)
at android.app.ActivityThread.handleDestroyActivity(ActivityThread.java:3849)
at android.app.ActivityThread.access$1500(ActivityThread.java:150)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1398)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Method.java)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:764)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:626)
Caused by java.lang.NullPointerException: ImagePipelineFactory was not initialized!
at com.facebook.common.internal.Preconditions.checkNotNull(Preconditions.java:226)
at com.facebook.imagepipeline.core.ImagePipelineFactory.getInstance(ImagePipelineFactory.java:74)
at com.facebook.drawee.backends.pipeline.Fresco.getImagePipelineFactory(Fresco.java:92)
at com.facebook.drawee.backends.pipeline.Fresco.getImagePipeline(Fresco.java:97)
at com.facebook.react.modules.fresco.FrescoModule.onHostDestroy(FrescoModule.java:186)
at com.facebook.react.bridge.ReactContext.onHostDestroy(ReactContext.java:240)
at com.facebook.react.ReactInstanceManager.moveToBeforeCreateLifecycleState(ReactInstanceManager.java:667)
at com.facebook.react.ReactInstanceManager.onHostDestroy(ReactInstanceManager.java:586)
at com.facebook.react.ReactInstanceManager.onHostDestroy(ReactInstanceManager.java:599)
at com.facebook.react.ReactActivityDelegate.onDestroy(ReactActivityDelegate.java:142)
at com.facebook.react.ReactActivity.onDestroy(ReactActivity.java:72)
at android.app.Activity.performDestroy(Activity.java:6456)
at android.app.Instrumentation.callActivityOnDestroy(Instrumentation.java:1143)
at android.app.ActivityThread.performDestroyActivity(ActivityThread.java:3818)
at android.app.ActivityThread.handleDestroyActivity(ActivityThread.java:3849)
at android.app.ActivityThread.access$1500(ActivityThread.java:150)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1398)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5417)
at java.lang.reflect.Method.invoke(Method.java)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:764)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:626)
```
This was introduced by d9ae27ba89
1. Create app with an image to be loaded
2. Background app before fresco has been initialised. (Very tight window to do this)
3. Should not crash
cc foghina
Closes https://github.com/facebook/react-native/pull/14359
Differential Revision: D5508505
Pulled By: hramos
fbshipit-source-id: 5a66d594625783f1c30180fe78c5baddb4f835aa
Summary:
This is the first PR from a series of PRs grabbou and me will make to add blob support to React Native. The next PR will include blob support for XMLHttpRequest.
I'd like to get this merged with minimal changes to preserve the attribution. My next PR can contain bigger changes.
Blobs are used to transfer binary data between server and client. Currently React Native lacks a way to deal with binary data. The only thing that comes close is uploading files through a URI.
Current workarounds to transfer binary data includes encoding and decoding them to base64 and and transferring them as string, which is not ideal, since it increases the payload size and the whole payload needs to be sent via the bridge every time changes are made.
The PR adds a way to deal with blobs via a new native module. The blob is constructed on the native side and the data never needs to pass through the bridge. Currently the only way to create a blob is to receive a blob from the server via websocket.
The PR is largely a direct port of https://github.com/silklabs/silk/tree/master/react-native-blobs by philikon into RN (with changes to integrate with RN), and attributed as such.
> **Note:** This is a breaking change for all people running iOS without CocoaPods. You will have to manually add `RCTBlob.xcodeproj` to your `Libraries` and then, add it to Build Phases. Just follow the process of manual linking. We'll also need to document this process in the release notes.
Related discussion - https://github.com/facebook/react-native/issues/11103
- `Image` can't show image when `URL.createObjectURL` is used with large images on Android
The websocket integration can be tested via a simple server,
```js
const fs = require('fs');
const http = require('http');
const WebSocketServer = require('ws').Server;
const wss = new WebSocketServer({
server: http.createServer().listen(7232),
});
wss.on('connection', (ws) => {
ws.on('message', (d) => {
console.log(d);
});
ws.send(fs.readFileSync('./some-file'));
});
```
Then on the client,
```js
var ws = new WebSocket('ws://localhost:7232');
ws.binaryType = 'blob';
ws.onerror = (error) => {
console.error(error);
};
ws.onmessage = (e) => {
console.log(e.data);
ws.send(e.data);
};
```
cc brentvatne ide
Closes https://github.com/facebook/react-native/pull/11417
Reviewed By: sahrens
Differential Revision: D5188484
Pulled By: javache
fbshipit-source-id: 6afcbc4d19aa7a27b0dc9d52701ba400e7d7e98f
Summary:
This should be functionally identical, but avoids unnecessary conditionals in the code.
<!--
Thank you for sending the PR!
If you changed any code, please provide us with clear instructions on how you verified your changes work. In other words, a test plan is *required*. Bonus points for screenshots and videos!
Please read the Contribution Guidelines at https://github.com/facebook/react-native/blob/master/CONTRIBUTING.md to learn more about contributing to React Native.
Happy contributing!
-->
Closes https://github.com/facebook/react-native/pull/15147
Differential Revision: D5497883
Pulled By: javache
fbshipit-source-id: a4b182084ffce87adac56013a178fbc5a7a5d1bb
Summary:
This change intends to fix 2 issues with the NetInfo API:
- The NetInfo API is currently platform-specific. It returns completely different values on iOS and Android.
- The NetInfo API currently doesn't expose a way to determine whether the connection is 2g, 3g, or 4g.
The NetInfo API currently just exposes a string-based enum representing the connectivity type. The string values are different between iOS and Andorid. Because of this design, it's not obvious how to achieve the goals of this change without making a breaking change. Consequently, this change deprecates the old NetInfo APIs and introduces new ones. Specifically, these are the API changes:
- The `fetch` method is deprecated in favor of `getConnection`
- The `change` event is deprecated in favor of the `connectionchange` event.
- `getConnection`/`connectionchange` use a new set of enum values compared to `fetch`/`change`. See the documentation for the new values.
- On iOS, `cell` is now known as `cellular`. It's worth pointing out this one in particular because the old and new names are so similar. The rest of the iOS values have remained the same.
- Some of the Android enum values have been removed without a replacement (e.g. `DUMMY`, `MOBILE_DUN`, `MOBILE_HIPRI`, `MOBILE_MMS`, `MOBILE_SUPL`, `VPN`). If desirable, we could find a way to expose these in the new API. For example, we could have a `platformValue` key that exposes the platform's enum values directly (like the old `fetch` API did).
`getConnection` and `connectionchange` each expose an object which has 2 keys conveying a `ConnectionType` (e.g. wifi, cellular) and an `EffectiveConnectionType` (e.g. 2g, 3g). These enums and their values are taken directly from the W3C's Network Information API spec (https://wicg.github.io/netinfo/). Copying the W3C's API will make it easy to expose a `navigation.connection` polyfill, if we want, in the future. Additionally, because the new APIs expose an object instead of a string, it's easier to extend the APIs in the future by adding keys to the object without causing a breaking change.
Note that the W3C's spec doesn't have an "unknown" value for `EffectiveConnectionType`. I chose to introduce this non-standard value because it's possible for the current implementation to not have an `effectiveConnectionType` and I figured it was worth representing this possibility explicitly with "unknown" instead of implicitly with `null`.
**Test Plan (required)**
Verified that the methods (`fetch` and `getConnection`) and the events (`change` and `connectionchange`) return the correct data on iOS and Android when connected to a wifi network and a 4G cellular network. Verified that switching networks causes the event to fire with the correct information. Verified that the old APIs (`fetch' and 'change') emit a deprecation warning when used. My team is using a similar patch in our app.
Adam Comella
Microsoft Corp.
Closes https://github.com/facebook/react-native/pull/14618
Differential Revision: D5459593
Pulled By: shergin
fbshipit-source-id: f1e6c5d572bb3e2669fbd4ba7d0fbb106525280e
Summary:
Fixes#11209
Updating action items in a `ToolbarAndroid`, or more specifically the native `ReactToolbar`, after the initial render presently will not always work as expected - typically manifesting itself as new action items not being displayed at all (under certain circumstances).
This seems to be happening because Fresco gets back to us asynchronously and updates the `MenuItem` in a listener. However when a keyboard is displayed the `Toolbar` is in a weird state where updating the `MenuItem` doesn't automatically trigger a layout.
The solution is to trigger one manually.
This is a bit wacky, so I created a sample project:
https://github.com/Benjamin-Dobell/DynamicToolbar
`master` demonstrates the problem. Run the project, the toolbar action item is scheduled to update every 2 seconds. It works fine _until_ you give the `TextInput` focus. Once you give it focus the action item disappears and it never recovers (despite on-going renders due to state changes).
You can then checkout the `fixed` branch, run `yarn` again, and see that problem is fixed. This branch is using a prebuilt version of React Native with this patch applied. Of course you could (and probably should) also modify `master` to use a version of RN built by the Facebook CI (assuming that's a thing you guys do).
Closes https://github.com/facebook/react-native/pull/13876
Differential Revision: D5476858
Pulled By: shergin
fbshipit-source-id: 6634d8cb3ee18fd99f7dc4e1eef348accc1c45ad
Summary:
<!--
Thank you for sending the PR!
If you changed any code, please provide us with clear instructions on how you verified your changes work. In other words, a test plan is *required*. Bonus points for screenshots and videos!
Please read the Contribution Guidelines at https://github.com/facebook/react-native/blob/master/CONTRIBUTING.md to learn more about contributing to React Native.
Happy contributing!
-->
Closes https://github.com/facebook/react-native/pull/15156
Differential Revision: D5479265
Pulled By: shergin
fbshipit-source-id: a2dfa3a4357e126838a17dac4797d1d845cd56ae
Summary:
Closes https://github.com/facebook/react-native/issues/7463.
This PR fixes the `HEAD` http requests in Android.
In Android all the HEAD http requests will fail, even if the request succeeds in the native layer due to an issue in the communication with the `OkHttp`.
Closes https://github.com/facebook/react-native/pull/14289
Differential Revision: D5166130
Pulled By: hramos
fbshipit-source-id: a7a0deee0fcb5f6a645c07d4e6f4386b5f550e31
Summary:
This diff changes the behaviour of natively driven animations in case the node that they are being run for has its value changed using `setValue` or as a result of an incoming event.
The reason for changing that is to match the JS implementation of `setValue` which behaves as described above (see relevant code here: 7cdd4d48c8/Libraries/Animated/src/AnimatedImplementation.js (L743))
**Test Plan:**
Use this sample app: https://snack.expo.io/B1V7RX9r-
Change: `USE_NATIVE_DRIVER` const between `true` and `false`.
See the animation stops regardless of the state of `USE_NATIVE_DRIVER` unlike before when it would stop only when `USE_NATIVE_DRIVER` was set to `false`
Closes https://github.com/facebook/react-native/pull/15054
Differential Revision: D5463750
Pulled By: hramos
fbshipit-source-id: e164c5299588ba8cac2937260c9ba9f6053b04e5
Summary:
On Android, using `ImageEditor.cropImage` with `displaySize` option may causes crash with exception below:
```
FATAL EXCEPTION: mqt_native_modules
Process: me.sohobloo.test, PID: 11308
com.facebook.react.bridge.UnexpectedNativeTypeException: TypeError: expected dynamic type `int64', but had type `double'
at com.facebook.react.bridge.ReadableNativeMap.getInt(Native Method)
at com.facebook.react.modules.camera.ImageEditingManager.cropImage(ImageEditingManager.java:196)
at java.lang.reflect.Method.invoke(Native Method)
at java.lang.reflect.Method.invoke(Method.java:372)
at com.facebook.react.bridge.BaseJavaModule$JavaMethod.invoke(BaseJavaModule.java:345)
at com.facebook.react.cxxbridge.JavaModuleWrapper.invoke(JavaModuleWrapper.java:141)
at com.facebook.react.bridge.queue.NativeRunnable.run(Native Method)
at android.os.Handler.handleCallback(Handler.java:815)
at android.os.Handler.dispatchMessage(Handler.java:104)
at com.facebook.react.bridge.queue.MessageQueueThreadHandler.dispatchMessage(MessageQueueThreadHandler.java:31)
at android.os.Looper.loop(Looper.java:194)
at com.facebook.react.bridge.queue.MessageQueueThreadImpl$3.run(MessageQueueThreadImpl.java:196)
at java.lang.Thread.run(Thread.java:818)
```
This is caused by getInt from `number` type of JS.
```javascript
ImageEditor.cropImage(
uri,
{
offset: {x: 0, y: 0},
size: {width: 320, height: 240},
displaySize: {width: 320.5, height: 240.5}
}
);
```
Closes https://github.com/facebook/react-native/pull/13312
Differential Revision: D5462709
Pulled By: shergin
fbshipit-source-id: 42cb853b533769b6969b8ac9ad50f3dd3c764055
Summary:
The support for `ReadableArray` in `toBundle` was never implemented, throwing an `UnsupportedOperationException` when trying to convert an array.
* Created `toList` -- A method that converts a `ReadableArray` to an `ArrayList`
* Modified `toBundle` to support arrays using `toList`
* Created `fromList` -- A method that converts a `List` to a `WritableArray`
* Modified `fromBundle` to also support lists using `fromList`
This PR allows `toBundle` and `fromBundle` (as well as `toList` and `fromList`) to work consistently without loosing information.
**Test Plan**
I've created three different arrays: one full of integers, one full of strings, and one mixed (with a integer, a boolean, a string, null, a map with a string and a boolean array), putting all of them inside a map.
After converting the map to a `Bundle` using `toBundle`, the integer array was retrieved through `Bundle.getIntegerArrayList`, the string array through `Bundle.getStringArrayList` and the mixed array through `Bundle.get` (casting it to an `ArrayList`)
After checking whether each value from the bundle was correct, I converted the bundle back to a map using `fromBundle`, and checked again every value.
The code and results from the test can be found in [this gist](https://gist.github.com/Guichaguri/5c7574b31f9584b6a9a0c182fd940a86).
Closes https://github.com/facebook/react-native/pull/15056
Differential Revision: D5460966
Pulled By: javache
fbshipit-source-id: a11b450eae4186e68bed7b8ce7dea8e5982e689a
Summary:
Convert to base64 not utf8
<!--
Thank you for sending the PR!
If you changed any code, please provide us with clear instructions on how you verified your changes work. In other words, a test plan is *required*. Bonus points for screenshots and videos!
Please read the Contribution Guidelines at https://github.com/facebook/react-native/blob/master/CONTRIBUTING.md to learn more about contributing to React Native.
Happy contributing!
-->
Closes https://github.com/facebook/react-native/pull/15046
Differential Revision: D5451398
Pulled By: javache
fbshipit-source-id: b8c6c7b0fb50ca9558e92d3f63a088e343791b7f
Summary:
This PR fixes an issue with rotation decomposition matrix on android.
The issue can be illustrated with this sample code https://snack.expo.io/r1SHEJpVb
It surfaces when we have non-zero rotation in Y or X axis and when rotation Z is greater than 90deg or less than -90deg. In that case the decomposition code doesn't give a valid output and as a result the view gets rotated by 180deg in Z axis.
You may want to run the code linked above on android and iOS to see the difference. Basically the example app renders first image rotated only by 89deg and the next one by 91deg. As a result you should see the second view being pivoted just slightly more than the first image. Apparently on android the second image is completely flipped:
iOS:
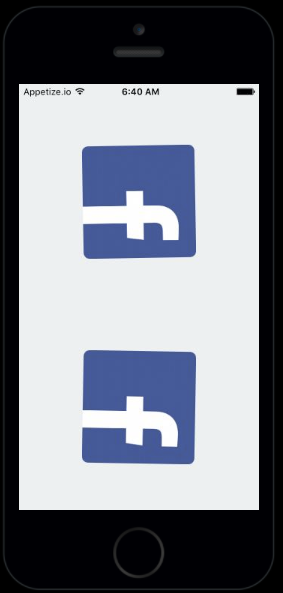
Android:
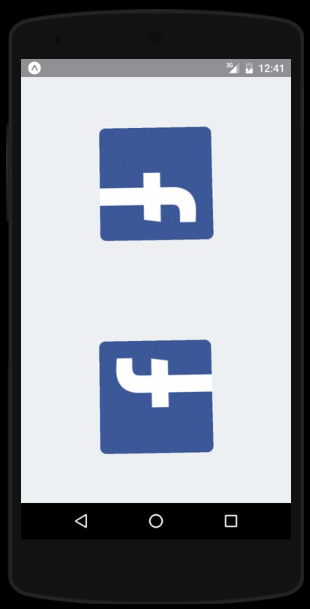
The bug seemed to be caused by the code that decomposes the matrix into axis angles. It seems like that whole code has been overly complicated and we've been converting matrix first into quaternion just to extract angles. Whereas it is sufficient to extract angles directly from rotation matrix as described here: http://nghiaho.com/?page_id=846
This formula produces way simpler code and also gives correct result in the aforementioned case, so I decided not to debug quaternion code any further.
sidenote: New formula's y angle output range is now -90 to 90deg hence changes in tests.
Closes https://github.com/facebook/react-native/pull/14888
Reviewed By: astreet
Differential Revision: D5414006
Pulled By: shergin
fbshipit-source-id: 2e0a68cf4b2a9e32f10f6bfff2d484867a337fa3
Summary:
This fixes support for the `backgroundColor` style prop on an `ART.Surface`.
`ARTSurfaceViewManager` inherits its `setBackgroundColor` implementation from `BaseViewManager`. This implementation broke in API 24 because API 24 removed support for `setBackgroundColor`on `TextureViews`. `ARTSurfaceView` inherits from `TextureView` so it also lost support for `setBackgroundColor`.
To fix this, the implementation of `ART.Surface's` `setBackgroundColor` was moved to the shadow node. The implementation now draws the background color on the canvas.
In a test app, verified that initializing and changing the background color on an `ART.Surface` works. Verified that the background renders as transparent when a background color isn't set. Also, this change is being used in my team's app.
Adam Comella
Microsoft Corp.
Closes https://github.com/facebook/react-native/pull/14117
Differential Revision: D5419574
Pulled By: hramos
fbshipit-source-id: 022bfed553e33e2d493f68b4bf5aa16dd304934d
Summary:
What existing problem does the pull request solve?
this fixes#13506 and #12717 where:
- there are some issues when pressing enter with some keyboard
- blurOnSubmit is not working with multiline
I think this should be in stable branch as this is pretty critical, isn't it?
- just create a TextInput with multiline and press enter with samsung keyboard
before, it won't create a new line, now it will.
- just create a TextInput with multiline and blurOnSubmit true and press enter
before, it won't blur, and wont create a new line (on some keyboard, it will create a new line), now it will blur only
Closes https://github.com/facebook/react-native/pull/13890
Reviewed By: achen1
Differential Revision: D5333464
Pulled By: shergin
fbshipit-source-id: a0597d1b1967d4de1486e728e03160e1bb15afeb