mirror of
https://github.com/status-im/react-native.git
synced 2025-01-27 09:45:04 +00:00
Add iOS 10 textContentType for TextInput
Summary: Setting `textContentType` will provide the keyboard and system with semantic meaning for inputs. Should enable password/username autofill in apps running on iOS 11+ as demonstrated here: https://developer.apple.com/videos/play/wwdc2017/206/ Also gives you the ability to disable autofill by setting `textContentType="none"`: https://stackoverflow.com/questions/48489479/react-native-disable-password-autofill-option-on-ios-keyboard Adding `textContentType` equal to `username` or `password` should give you an autofill-bar over the keyboard which will let you fill in values from the device Keychain: 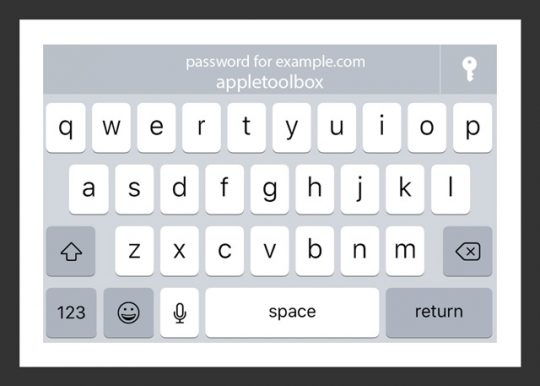 Setting the appropriate `textContentType` will fill in the correct value in the `TextInput`. I have only been able to get this to work on device, and not simulator. Usage: ```jsx <TextInput value={this.state.username} onChangeText={this.setUserName} textContentType="username" /> ``` ```jsx <TextInput value={this.state.password} onChangeText={this.setPassword} secureTextEntry={true} textContentType="password" /> ``` To disable: ```jsx <TextInput value={this.state.password} onChangeText={this.setPassword} secureTextEntry={true} textContentType="none" /> ``` This will set `textContentType` to an empty string: https://stackoverflow.com/a/46474180/5703116 <!-- Does this PR require a documentation change? Create a PR at https://github.com/facebook/react-native-website and add a link to it here. --> Docs PR coming up. [IOS] [MINOR] [TextInput] - Added `textContentType` prop for iOS 10+. Will enable password autofill for iOS 11+. Closes https://github.com/facebook/react-native/pull/18526 Differential Revision: D7469630 Pulled By: hramos fbshipit-source-id: 852a9749be98d477ecd82154c0a65a7c084521c1
This commit is contained in:
parent
8a99241f81
commit
d4fb87b0b8
@ -605,6 +605,39 @@ const TextInput = createReactClass({
|
||||
* @platform ios
|
||||
*/
|
||||
inputAccessoryViewID: PropTypes.string,
|
||||
/**
|
||||
* Give the keyboard and the system information about the
|
||||
* expected semantic meaning for the content that users enter.
|
||||
* @platform ios
|
||||
*/
|
||||
textContentType: PropTypes.oneOf([
|
||||
'none',
|
||||
'URL',
|
||||
'addressCity',
|
||||
'addressCityAndState',
|
||||
'addressState',
|
||||
'countryName',
|
||||
'creditCardNumber',
|
||||
'emailAddress',
|
||||
'familyName',
|
||||
'fullStreetAddress',
|
||||
'givenName',
|
||||
'jobTitle',
|
||||
'location',
|
||||
'middleName',
|
||||
'name',
|
||||
'namePrefix',
|
||||
'nameSuffix',
|
||||
'nickname',
|
||||
'organizationName',
|
||||
'postalCode',
|
||||
'streetAddressLine1',
|
||||
'streetAddressLine2',
|
||||
'sublocality',
|
||||
'telephoneNumber',
|
||||
'username',
|
||||
'password',
|
||||
]),
|
||||
},
|
||||
getDefaultProps(): Object {
|
||||
return {
|
||||
|
@ -167,6 +167,17 @@ RCT_NOT_IMPLEMENTED(- (instancetype)initWithFrame:(CGRect)frame)
|
||||
}
|
||||
}
|
||||
|
||||
- (void)setTextContentType:(NSString *)type
|
||||
{
|
||||
#if defined(__IPHONE_OS_VERSION_MAX_ALLOWED) && __IPHONE_OS_VERSION_MAX_ALLOWED >= __IPHONE_10_0
|
||||
if (@available(iOS 10.0, *)) {
|
||||
// Setting textContentType to an empty string will disable any
|
||||
// default behaviour, like the autofill bar for password inputs
|
||||
self.backedTextInputView.textContentType = [type isEqualToString:@"none"] ? @"" : type;
|
||||
}
|
||||
#endif
|
||||
}
|
||||
|
||||
#pragma mark - RCTBackedTextInputDelegate
|
||||
|
||||
- (BOOL)textInputShouldBeginEditing
|
||||
|
@ -55,6 +55,7 @@ RCT_EXPORT_VIEW_PROPERTY(maxLength, NSNumber)
|
||||
RCT_EXPORT_VIEW_PROPERTY(selectTextOnFocus, BOOL)
|
||||
RCT_EXPORT_VIEW_PROPERTY(selection, RCTTextSelection)
|
||||
RCT_EXPORT_VIEW_PROPERTY(inputAccessoryViewID, NSString)
|
||||
RCT_EXPORT_VIEW_PROPERTY(textContentType, NSString)
|
||||
|
||||
RCT_EXPORT_VIEW_PROPERTY(onChange, RCTBubblingEventBlock)
|
||||
RCT_EXPORT_VIEW_PROPERTY(onSelectionChange, RCTDirectEventBlock)
|
||||
|
Loading…
x
Reference in New Issue
Block a user