prevent scheduling unnecessary layoutanimation
Summary: when a hardware keyboard is connected, the virtual keyboard can be hidden (this can easily be demonstrated in the simulator), which means the height of the keyboard is 0. When in this case a `LayoutAnimation` is scheduled, the `KeyboardAvoidingView` won't be affected, but the next layout change will be animated, which can have unintended side-effects. This can also trigger the `Overriding previous layout animation with new one before the first began` warning. <details> <summary>Screenshot</summary> 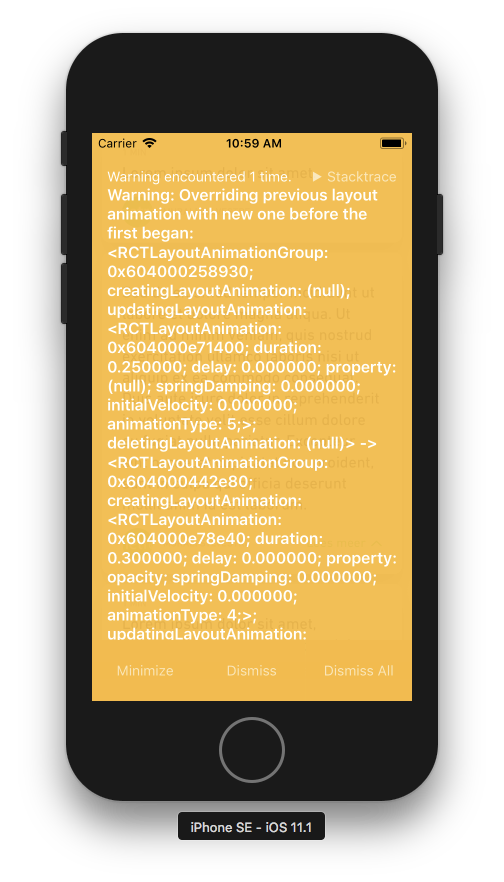 </details> Open the `KeyboardAvoidingView` example in the `RNTester` project, import `LayoutAnimation` and add something rendered conditionally to the content of the `Modal`, e.g.; ```jsx {this.state.behavior === 'position' && <Text>We're using position now</Text> } ``` Then update the `onSegmentChange` handler with a `LayoutAnimation`; ```js onSegmentChange = (segment: String) => { LayoutAnimation.easeInEaseOut(); this.setState({behavior: segment.toLowerCase()}); }; ``` Now open the example in the simulator and play with the "Toggle Software Keyboard" option; 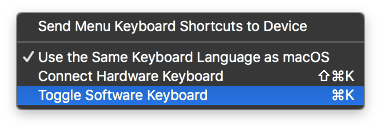 Now when you focus the input, no keyboard should appear, and when you then press an option of the segmented control, you should get the beforementioned warning. After this change this warning will no longer appear, but the component still behaves the same as before. [IOS] [BUGFIX] [KeyboardAvoidingView] - prevent scheduling unnecessary `LayoutAnimation` Closes https://github.com/facebook/react-native/pull/16984 Differential Revision: D6472300 Pulled By: shergin fbshipit-source-id: c4041dfdd846cdc88b2e9d281517ed79da99dfe7
This commit is contained in:
parent
5b83dbe25a
commit
ad4450ac13
|
@ -107,6 +107,10 @@ const KeyboardAvoidingView = createReactClass({
|
|||
const {duration, easing, endCoordinates} = event;
|
||||
const height = this._relativeKeyboardHeight(endCoordinates);
|
||||
|
||||
if (this.state.bottom === height) {
|
||||
return;
|
||||
}
|
||||
|
||||
if (duration && easing) {
|
||||
LayoutAnimation.configureNext({
|
||||
duration: duration,
|
||||
|
|
Loading…
Reference in New Issue