- Keyboard layout now updates when changing keyboardType while it has focus (#19027)
Summary: This PR makes sure that changing the `keyboardType` props of `<TextInput>` is reflected while the text field has focus. It is something that is also discusses in #13782. The workaround mentioned in that issue using `key` causes the TextInput to re-render itself which has some undesired side-effects. Fixes #13782 ```javascript export default class KeyboardTypeApp extends Component { state = { keyboardType: 'default' }; toggleKeyboardType = () => { this.setState({ keyboardType: this.state.keyboardType === 'default' ? 'numeric' : 'default' }); } render() { return ( <View style={{ padding: 40 }}> <TextInput autoFocus value="Press Toggle :)" keyboardType={this.state.keyboardType} /> <Button title="Toggle" onPress={this.toggleKeyboardType} /> </View> ); } } ``` 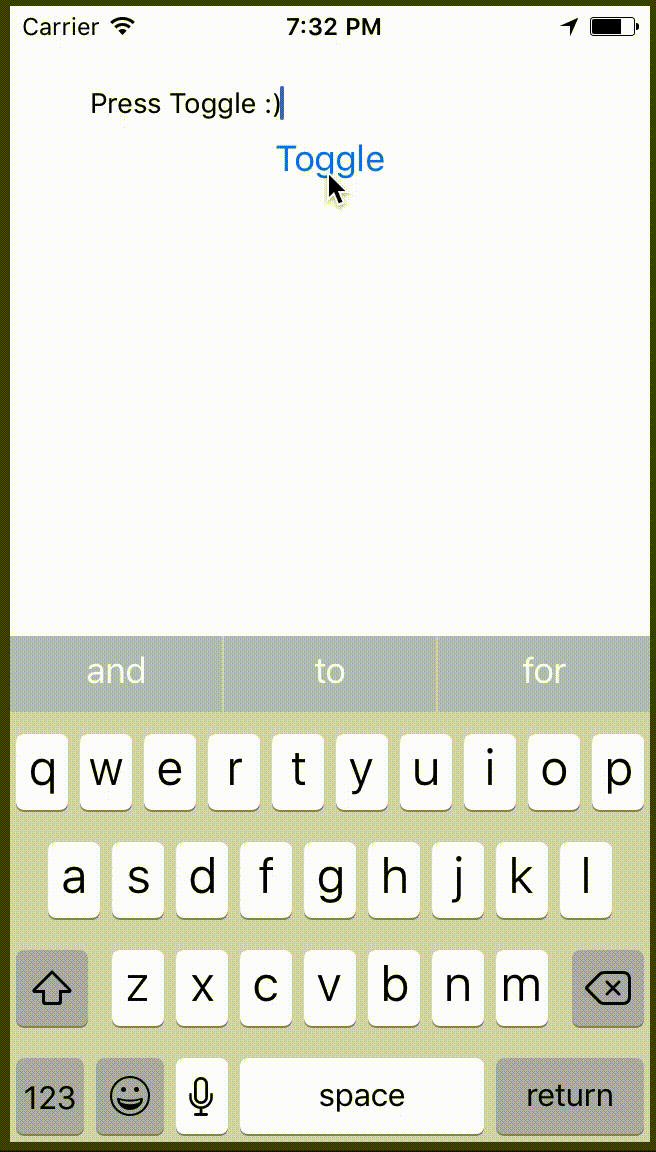 <!-- Does this PR require a documentation change? Create a PR at https://github.com/facebook/react-native-website and add a link to it here. --> [IOS] [ENHANCEMENT] [TextInput] - Keyboard layout now updates when changing `keyboardType` while it has focus <!-- **INTERNAL and MINOR tagged notes will not be included in the next version's final release notes.** CATEGORY [----------] TYPE [ CLI ] [-------------] LOCATION [ DOCS ] [ BREAKING ] [-------------] [ GENERAL ] [ BUGFIX ] [ {Component} ] [ INTERNAL ] [ ENHANCEMENT ] [ {Filename} ] [ IOS ] [ FEATURE ] [ {Directory} ] |-----------| [ ANDROID ] [ MINOR ] [ {Framework} ] - | {Message} | [----------] [-------------] [-------------] |-----------| EXAMPLES: [IOS] [BREAKING] [FlatList] - Change a thing that breaks other things [ANDROID] [BUGFIX] [TextInput] - Did a thing to TextInput [CLI] [FEATURE] [local-cli/info/info.js] - CLI easier to do things with [DOCS] [BUGFIX] [GettingStarted.md] - Accidentally a thing/word [GENERAL] [ENHANCEMENT] [Yoga] - Added new yoga thing/position [INTERNAL] [FEATURE] [./scripts] - Added thing to script that nobody will see --> Closes https://github.com/facebook/react-native/pull/19027 Differential Revision: D8416007 Pulled By: PeteTheHeat fbshipit-source-id: c4f89ab3dc0819bca52feddbc9c7a9f62fd96794
This commit is contained in:
parent
f6da9e1a9a
commit
8baaacb664
|
@ -47,6 +47,7 @@ NS_ASSUME_NONNULL_BEGIN
|
|||
@property (nonatomic, strong, nullable) NSNumber *maxLength;
|
||||
@property (nonatomic, copy) NSAttributedString *attributedText;
|
||||
@property (nonatomic, copy) NSString *inputAccessoryViewID;
|
||||
@property (nonatomic, assign) UIKeyboardType keyboardType;
|
||||
|
||||
@end
|
||||
|
||||
|
|
|
@ -178,6 +178,23 @@ RCT_NOT_IMPLEMENTED(- (instancetype)initWithFrame:(CGRect)frame)
|
|||
#endif
|
||||
}
|
||||
|
||||
- (UIKeyboardType)keyboardType
|
||||
{
|
||||
return self.backedTextInputView.keyboardType;
|
||||
}
|
||||
|
||||
- (void)setKeyboardType:(UIKeyboardType)keyboardType
|
||||
{
|
||||
UIView<RCTBackedTextInputViewProtocol> *textInputView = self.backedTextInputView;
|
||||
if (textInputView.keyboardType != keyboardType) {
|
||||
textInputView.keyboardType = keyboardType;
|
||||
// Without the call to reloadInputViews, the keyboard will not change until the textview field (the first responder) loses and regains focus.
|
||||
if (textInputView.isFirstResponder) {
|
||||
[textInputView reloadInputViews];
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
#pragma mark - RCTBackedTextInputDelegate
|
||||
|
||||
- (BOOL)textInputShouldBeginEditing
|
||||
|
|
|
@ -40,7 +40,6 @@ RCT_REMAP_VIEW_PROPERTY(contextMenuHidden, backedTextInputView.contextMenuHidden
|
|||
RCT_REMAP_VIEW_PROPERTY(editable, backedTextInputView.editable, BOOL)
|
||||
RCT_REMAP_VIEW_PROPERTY(enablesReturnKeyAutomatically, backedTextInputView.enablesReturnKeyAutomatically, BOOL)
|
||||
RCT_REMAP_VIEW_PROPERTY(keyboardAppearance, backedTextInputView.keyboardAppearance, UIKeyboardAppearance)
|
||||
RCT_REMAP_VIEW_PROPERTY(keyboardType, backedTextInputView.keyboardType, UIKeyboardType)
|
||||
RCT_REMAP_VIEW_PROPERTY(placeholder, backedTextInputView.placeholder, NSString)
|
||||
RCT_REMAP_VIEW_PROPERTY(placeholderTextColor, backedTextInputView.placeholderColor, UIColor)
|
||||
RCT_REMAP_VIEW_PROPERTY(returnKeyType, backedTextInputView.returnKeyType, UIReturnKeyType)
|
||||
|
@ -51,6 +50,7 @@ RCT_REMAP_VIEW_PROPERTY(caretHidden, backedTextInputView.caretHidden, BOOL)
|
|||
RCT_REMAP_VIEW_PROPERTY(clearButtonMode, backedTextInputView.clearButtonMode, UITextFieldViewMode)
|
||||
RCT_EXPORT_VIEW_PROPERTY(blurOnSubmit, BOOL)
|
||||
RCT_EXPORT_VIEW_PROPERTY(clearTextOnFocus, BOOL)
|
||||
RCT_EXPORT_VIEW_PROPERTY(keyboardType, UIKeyboardType)
|
||||
RCT_EXPORT_VIEW_PROPERTY(maxLength, NSNumber)
|
||||
RCT_EXPORT_VIEW_PROPERTY(selectTextOnFocus, BOOL)
|
||||
RCT_EXPORT_VIEW_PROPERTY(selection, RCTTextSelection)
|
||||
|
|
Loading…
Reference in New Issue