2015-01-30 01:10:49 +00:00
|
|
|
/**
|
2017-05-06 03:50:47 +00:00
|
|
|
* Copyright (c) 2015-present, Facebook, Inc.
|
2016-07-12 12:51:57 +00:00
|
|
|
*
|
2018-02-17 02:24:55 +00:00
|
|
|
* This source code is licensed under the MIT license found in the
|
|
|
|
* LICENSE file in the root directory of this source tree.
|
2016-07-12 12:51:57 +00:00
|
|
|
*
|
2015-03-23 22:07:33 +00:00
|
|
|
* @flow
|
2015-01-30 01:10:49 +00:00
|
|
|
*/
|
|
|
|
'use strict';
|
|
|
|
|
2016-04-09 03:36:40 +00:00
|
|
|
var React = require('react');
|
|
|
|
var ReactNative = require('react-native');
|
2015-01-30 01:10:49 +00:00
|
|
|
var {
|
2016-10-25 07:18:34 +00:00
|
|
|
Animated,
|
2015-01-30 01:10:49 +00:00
|
|
|
Image,
|
|
|
|
StyleSheet,
|
|
|
|
Text,
|
|
|
|
TouchableHighlight,
|
2015-03-02 18:45:03 +00:00
|
|
|
TouchableOpacity,
|
2016-02-24 13:25:51 +00:00
|
|
|
Platform,
|
|
|
|
TouchableNativeFeedback,
|
2015-01-30 01:10:49 +00:00
|
|
|
View,
|
2016-04-09 03:36:40 +00:00
|
|
|
} = ReactNative;
|
2015-01-30 01:10:49 +00:00
|
|
|
|
2017-03-22 16:58:00 +00:00
|
|
|
const NativeModules = require('NativeModules');
|
|
|
|
|
|
|
|
const forceTouchAvailable = (NativeModules.PlatformConstants &&
|
|
|
|
NativeModules.PlatformConstants.forceTouchAvailable) || false;
|
|
|
|
|
2015-06-22 16:43:30 +00:00
|
|
|
exports.displayName = (undefined: ?string);
|
2015-09-15 21:46:54 +00:00
|
|
|
exports.description = 'Touchable and onPress examples.';
|
2015-01-30 01:10:49 +00:00
|
|
|
exports.title = '<Touchable*> and onPress';
|
|
|
|
exports.examples = [
|
|
|
|
{
|
|
|
|
title: '<TouchableHighlight>',
|
|
|
|
description: 'TouchableHighlight works by adding an extra view with a ' +
|
|
|
|
'black background under the single child view. This works best when the ' +
|
|
|
|
'child view is fully opaque, although it can be made to work as a simple ' +
|
|
|
|
'background color change as well with the activeOpacity and ' +
|
|
|
|
'underlayColor props.',
|
|
|
|
render: function() {
|
|
|
|
return (
|
|
|
|
<View>
|
|
|
|
<View style={styles.row}>
|
|
|
|
<TouchableHighlight
|
|
|
|
style={styles.wrapper}
|
|
|
|
onPress={() => console.log('stock THW image - highlight')}>
|
|
|
|
<Image
|
|
|
|
source={heartImage}
|
|
|
|
style={styles.image}
|
|
|
|
/>
|
|
|
|
</TouchableHighlight>
|
|
|
|
<TouchableHighlight
|
|
|
|
style={styles.wrapper}
|
|
|
|
activeOpacity={1}
|
|
|
|
animationVelocity={0}
|
onPress animation with magnification
Summary:
Related to: #15454
Motivation: Improve tvOS feeling for TouchableHighlight
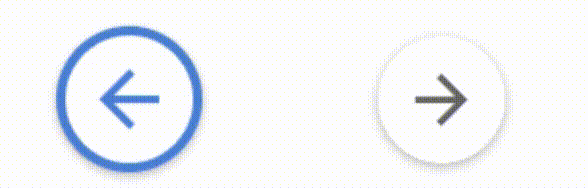
- When you select the button he is focus and the underlay is show
- When you press the button, there is an animation, but after the animation, the focus is on the button and the underlay is show
Play with tvParallaxProperties on tvOS, test with and without patch just to see the actual behaviour
```
<TouchableHighlight
tvParallaxProperties={{
enabled: true,
shiftDistanceX: 0,
shiftDistanceY: 0,
tiltAngle: 0,
magnification: 1.1,
pressMagnification: 1.0,
pressDuration: 0.3,
}}
underlayColor="black"
onShowUnderlay={() => (console.log("onShowUnderlay")}
onHideUnderlay={() => (console.log("onHideUnderlay")}
onPress={() => (console.log("onPress")}
>
<Image
style={styles.image}
source={ uri: 'https://www.facebook.com/images/fb_icon_325x325.png' }
/>
</TouchableHighlight>
```
Closes https://github.com/facebook/react-native/pull/15455
Differential Revision: D6887437
Pulled By: hramos
fbshipit-source-id: e18b695068bc99643ba4006fb3f39215b38a74c1
2018-02-27 20:52:21 +00:00
|
|
|
tvParallaxProperties={{pressMagnification: 1.3, pressDuration: 0.6}}
|
2015-01-30 01:10:49 +00:00
|
|
|
underlayColor="rgb(210, 230, 255)"
|
2016-02-24 12:34:12 +00:00
|
|
|
onPress={() => console.log('custom THW text - highlight')}>
|
2015-01-30 01:10:49 +00:00
|
|
|
<View style={styles.wrapperCustom}>
|
|
|
|
<Text style={styles.text}>
|
|
|
|
Tap Here For Custom Highlight!
|
|
|
|
</Text>
|
|
|
|
</View>
|
|
|
|
</TouchableHighlight>
|
|
|
|
</View>
|
|
|
|
</View>
|
|
|
|
);
|
|
|
|
},
|
2016-10-25 07:18:34 +00:00
|
|
|
}, {
|
|
|
|
title: 'TouchableNativeFeedback with Animated child',
|
|
|
|
description: 'TouchableNativeFeedback can have an AnimatedComponent as a' +
|
|
|
|
'direct child.',
|
|
|
|
platform: 'android',
|
|
|
|
render: function() {
|
|
|
|
const mScale = new Animated.Value(1);
|
|
|
|
Animated.timing(mScale, {toValue: 0.3, duration: 1000}).start();
|
|
|
|
const style = {
|
|
|
|
backgroundColor: 'rgb(180, 64, 119)',
|
|
|
|
width: 200,
|
|
|
|
height: 100,
|
|
|
|
transform: [{scale: mScale}]
|
|
|
|
};
|
|
|
|
return (
|
|
|
|
<View>
|
|
|
|
<View style={styles.row}>
|
|
|
|
<TouchableNativeFeedback>
|
|
|
|
<Animated.View style={style}/>
|
|
|
|
</TouchableNativeFeedback>
|
|
|
|
</View>
|
|
|
|
</View>
|
|
|
|
);
|
|
|
|
},
|
2015-01-30 01:10:49 +00:00
|
|
|
}, {
|
|
|
|
title: '<Text onPress={fn}> with highlight',
|
2016-10-16 11:11:59 +00:00
|
|
|
render: function(): React.Element<any> {
|
2015-01-30 01:10:49 +00:00
|
|
|
return <TextOnPressBox />;
|
|
|
|
},
|
2015-03-02 18:45:03 +00:00
|
|
|
}, {
|
|
|
|
title: 'Touchable feedback events',
|
|
|
|
description: '<Touchable*> components accept onPress, onPressIn, ' +
|
|
|
|
'onPressOut, and onLongPress as props.',
|
2016-10-16 11:11:59 +00:00
|
|
|
render: function(): React.Element<any> {
|
2015-03-02 18:45:03 +00:00
|
|
|
return <TouchableFeedbackEvents />;
|
|
|
|
},
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
}, {
|
|
|
|
title: 'Touchable delay for events',
|
|
|
|
description: '<Touchable*> components also accept delayPressIn, ' +
|
|
|
|
'delayPressOut, and delayLongPress as props. These props impact the ' +
|
|
|
|
'timing of feedback events.',
|
2016-10-16 11:11:59 +00:00
|
|
|
render: function(): React.Element<any> {
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
return <TouchableDelayEvents />;
|
|
|
|
},
|
2016-01-27 17:04:14 +00:00
|
|
|
}, {
|
|
|
|
title: '3D Touch / Force Touch',
|
|
|
|
description: 'iPhone 6s and 6s plus support 3D touch, which adds a force property to touches',
|
2016-10-16 11:11:59 +00:00
|
|
|
render: function(): React.Element<any> {
|
2016-01-27 17:04:14 +00:00
|
|
|
return <ForceTouchExample />;
|
|
|
|
},
|
|
|
|
platform: 'ios',
|
2016-02-17 00:50:35 +00:00
|
|
|
}, {
|
|
|
|
title: 'Touchable Hit Slop',
|
|
|
|
description: '<Touchable*> components accept hitSlop prop which extends the touch area ' +
|
|
|
|
'without changing the view bounds.',
|
2016-10-16 11:11:59 +00:00
|
|
|
render: function(): React.Element<any> {
|
2016-02-17 00:50:35 +00:00
|
|
|
return <TouchableHitSlop />;
|
|
|
|
},
|
2016-10-25 07:18:34 +00:00
|
|
|
}, {
|
2016-02-24 03:38:51 +00:00
|
|
|
title: 'Disabled Touchable*',
|
|
|
|
description: '<Touchable*> components accept disabled prop which prevents ' +
|
|
|
|
'any interaction with component',
|
2016-10-16 11:11:59 +00:00
|
|
|
render: function(): React.Element<any> {
|
2016-02-24 03:38:51 +00:00
|
|
|
return <TouchableDisabled />;
|
|
|
|
},
|
2016-02-17 00:50:35 +00:00
|
|
|
}];
|
2015-01-30 01:10:49 +00:00
|
|
|
|
2017-08-18 01:36:54 +00:00
|
|
|
class TextOnPressBox extends React.Component<{}, $FlowFixMeState> {
|
2016-07-26 08:00:02 +00:00
|
|
|
state = {
|
|
|
|
timesPressed: 0,
|
|
|
|
};
|
|
|
|
|
|
|
|
textOnPress = () => {
|
2015-01-30 01:10:49 +00:00
|
|
|
this.setState({
|
|
|
|
timesPressed: this.state.timesPressed + 1,
|
|
|
|
});
|
2016-07-26 08:00:02 +00:00
|
|
|
};
|
|
|
|
|
|
|
|
render() {
|
2015-01-30 01:10:49 +00:00
|
|
|
var textLog = '';
|
|
|
|
if (this.state.timesPressed > 1) {
|
|
|
|
textLog = this.state.timesPressed + 'x text onPress';
|
|
|
|
} else if (this.state.timesPressed > 0) {
|
|
|
|
textLog = 'text onPress';
|
|
|
|
}
|
|
|
|
|
|
|
|
return (
|
|
|
|
<View>
|
|
|
|
<Text
|
|
|
|
style={styles.textBlock}
|
|
|
|
onPress={this.textOnPress}>
|
|
|
|
Text has built-in onPress handling
|
|
|
|
</Text>
|
|
|
|
<View style={styles.logBox}>
|
|
|
|
<Text>
|
|
|
|
{textLog}
|
|
|
|
</Text>
|
|
|
|
</View>
|
|
|
|
</View>
|
|
|
|
);
|
|
|
|
}
|
2016-07-26 08:00:02 +00:00
|
|
|
}
|
2015-01-30 01:10:49 +00:00
|
|
|
|
2017-08-18 01:36:54 +00:00
|
|
|
class TouchableFeedbackEvents extends React.Component<{}, $FlowFixMeState> {
|
2016-07-26 08:00:02 +00:00
|
|
|
state = {
|
|
|
|
eventLog: [],
|
|
|
|
};
|
|
|
|
|
|
|
|
render() {
|
2015-03-02 18:45:03 +00:00
|
|
|
return (
|
2015-07-10 10:59:29 +00:00
|
|
|
<View testID="touchable_feedback_events">
|
2015-03-02 18:45:03 +00:00
|
|
|
<View style={[styles.row, {justifyContent: 'center'}]}>
|
2016-01-27 17:04:14 +00:00
|
|
|
<TouchableOpacity
|
|
|
|
style={styles.wrapper}
|
|
|
|
testID="touchable_feedback_events_button"
|
2016-02-04 13:12:36 +00:00
|
|
|
accessibilityLabel="touchable feedback events"
|
|
|
|
accessibilityTraits="button"
|
|
|
|
accessibilityComponentType="button"
|
2016-01-27 17:04:14 +00:00
|
|
|
onPress={() => this._appendEvent('press')}
|
|
|
|
onPressIn={() => this._appendEvent('pressIn')}
|
|
|
|
onPressOut={() => this._appendEvent('pressOut')}
|
|
|
|
onLongPress={() => this._appendEvent('longPress')}>
|
|
|
|
<Text style={styles.button}>
|
|
|
|
Press Me
|
|
|
|
</Text>
|
|
|
|
</TouchableOpacity>
|
|
|
|
</View>
|
2015-07-10 10:59:29 +00:00
|
|
|
<View testID="touchable_feedback_events_console" style={styles.eventLogBox}>
|
2015-03-02 18:45:03 +00:00
|
|
|
{this.state.eventLog.map((e, ii) => <Text key={ii}>{e}</Text>)}
|
|
|
|
</View>
|
|
|
|
</View>
|
|
|
|
);
|
2016-07-26 08:00:02 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
_appendEvent = (eventName) => {
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
var limit = 6;
|
|
|
|
var eventLog = this.state.eventLog.slice(0, limit - 1);
|
|
|
|
eventLog.unshift(eventName);
|
|
|
|
this.setState({eventLog});
|
2016-07-26 08:00:02 +00:00
|
|
|
};
|
|
|
|
}
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
|
2017-08-18 01:36:54 +00:00
|
|
|
class TouchableDelayEvents extends React.Component<{}, $FlowFixMeState> {
|
2016-07-26 08:00:02 +00:00
|
|
|
state = {
|
|
|
|
eventLog: [],
|
|
|
|
};
|
|
|
|
|
|
|
|
render() {
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
return (
|
2015-07-10 10:59:29 +00:00
|
|
|
<View testID="touchable_delay_events">
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
<View style={[styles.row, {justifyContent: 'center'}]}>
|
2016-01-27 17:04:14 +00:00
|
|
|
<TouchableOpacity
|
|
|
|
style={styles.wrapper}
|
|
|
|
testID="touchable_delay_events_button"
|
|
|
|
onPress={() => this._appendEvent('press')}
|
|
|
|
delayPressIn={400}
|
|
|
|
onPressIn={() => this._appendEvent('pressIn - 400ms delay')}
|
|
|
|
delayPressOut={1000}
|
|
|
|
onPressOut={() => this._appendEvent('pressOut - 1000ms delay')}
|
|
|
|
delayLongPress={800}
|
|
|
|
onLongPress={() => this._appendEvent('longPress - 800ms delay')}>
|
|
|
|
<Text style={styles.button}>
|
|
|
|
Press Me
|
|
|
|
</Text>
|
|
|
|
</TouchableOpacity>
|
|
|
|
</View>
|
2015-07-10 10:59:29 +00:00
|
|
|
<View style={styles.eventLogBox} testID="touchable_delay_events_console">
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
{this.state.eventLog.map((e, ii) => <Text key={ii}>{e}</Text>)}
|
|
|
|
</View>
|
|
|
|
</View>
|
|
|
|
);
|
2016-07-26 08:00:02 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
_appendEvent = (eventName) => {
|
2015-03-02 18:45:03 +00:00
|
|
|
var limit = 6;
|
|
|
|
var eventLog = this.state.eventLog.slice(0, limit - 1);
|
|
|
|
eventLog.unshift(eventName);
|
|
|
|
this.setState({eventLog});
|
2016-07-26 08:00:02 +00:00
|
|
|
};
|
|
|
|
}
|
2015-03-02 18:45:03 +00:00
|
|
|
|
2017-08-18 01:36:54 +00:00
|
|
|
class ForceTouchExample extends React.Component<{}, $FlowFixMeState> {
|
2016-07-26 08:00:02 +00:00
|
|
|
state = {
|
|
|
|
force: 0,
|
|
|
|
};
|
|
|
|
|
|
|
|
_renderConsoleText = () => {
|
2017-03-22 16:58:00 +00:00
|
|
|
return forceTouchAvailable ?
|
2016-01-27 17:04:14 +00:00
|
|
|
'Force: ' + this.state.force.toFixed(3) :
|
|
|
|
'3D Touch is not available on this device';
|
2016-07-26 08:00:02 +00:00
|
|
|
};
|
|
|
|
|
|
|
|
render() {
|
2016-01-27 17:04:14 +00:00
|
|
|
return (
|
|
|
|
<View testID="touchable_3dtouch_event">
|
|
|
|
<View style={styles.forceTouchBox} testID="touchable_3dtouch_output">
|
|
|
|
<Text>{this._renderConsoleText()}</Text>
|
|
|
|
</View>
|
|
|
|
<View style={[styles.row, {justifyContent: 'center'}]}>
|
|
|
|
<View
|
|
|
|
style={styles.wrapper}
|
|
|
|
testID="touchable_3dtouch_button"
|
|
|
|
onStartShouldSetResponder={() => true}
|
|
|
|
onResponderMove={(event) => this.setState({force: event.nativeEvent.force})}
|
|
|
|
onResponderRelease={(event) => this.setState({force: 0})}>
|
|
|
|
<Text style={styles.button}>
|
|
|
|
Press Me
|
|
|
|
</Text>
|
|
|
|
</View>
|
|
|
|
</View>
|
|
|
|
</View>
|
|
|
|
);
|
2016-07-26 08:00:02 +00:00
|
|
|
}
|
|
|
|
}
|
2016-01-27 17:04:14 +00:00
|
|
|
|
2017-08-18 01:36:54 +00:00
|
|
|
class TouchableHitSlop extends React.Component<{}, $FlowFixMeState> {
|
2016-07-26 08:00:02 +00:00
|
|
|
state = {
|
|
|
|
timesPressed: 0,
|
|
|
|
};
|
|
|
|
|
|
|
|
onPress = () => {
|
2016-02-17 00:50:35 +00:00
|
|
|
this.setState({
|
|
|
|
timesPressed: this.state.timesPressed + 1,
|
|
|
|
});
|
2016-07-26 08:00:02 +00:00
|
|
|
};
|
|
|
|
|
|
|
|
render() {
|
2016-02-17 00:50:35 +00:00
|
|
|
var log = '';
|
|
|
|
if (this.state.timesPressed > 1) {
|
|
|
|
log = this.state.timesPressed + 'x onPress';
|
|
|
|
} else if (this.state.timesPressed > 0) {
|
|
|
|
log = 'onPress';
|
|
|
|
}
|
|
|
|
|
|
|
|
return (
|
|
|
|
<View testID="touchable_hit_slop">
|
|
|
|
<View style={[styles.row, {justifyContent: 'center'}]}>
|
|
|
|
<TouchableOpacity
|
|
|
|
onPress={this.onPress}
|
|
|
|
style={styles.hitSlopWrapper}
|
|
|
|
hitSlop={{top: 30, bottom: 30, left: 60, right: 60}}
|
|
|
|
testID="touchable_hit_slop_button">
|
|
|
|
<Text style={styles.hitSlopButton}>
|
|
|
|
Press Outside This View
|
|
|
|
</Text>
|
|
|
|
</TouchableOpacity>
|
|
|
|
</View>
|
|
|
|
<View style={styles.logBox}>
|
|
|
|
<Text>
|
|
|
|
{log}
|
|
|
|
</Text>
|
|
|
|
</View>
|
|
|
|
</View>
|
|
|
|
);
|
|
|
|
}
|
2016-07-26 08:00:02 +00:00
|
|
|
}
|
2016-02-17 00:50:35 +00:00
|
|
|
|
2017-08-18 01:36:54 +00:00
|
|
|
class TouchableDisabled extends React.Component<{}> {
|
2016-07-26 08:00:02 +00:00
|
|
|
render() {
|
2016-02-24 03:38:51 +00:00
|
|
|
return (
|
|
|
|
<View>
|
|
|
|
<TouchableOpacity disabled={true} style={[styles.row, styles.block]}>
|
|
|
|
<Text style={styles.disabledButton}>Disabled TouchableOpacity</Text>
|
|
|
|
</TouchableOpacity>
|
|
|
|
|
|
|
|
<TouchableOpacity disabled={false} style={[styles.row, styles.block]}>
|
|
|
|
<Text style={styles.button}>Enabled TouchableOpacity</Text>
|
|
|
|
</TouchableOpacity>
|
|
|
|
|
|
|
|
<TouchableHighlight
|
|
|
|
activeOpacity={1}
|
|
|
|
disabled={true}
|
|
|
|
animationVelocity={0}
|
|
|
|
underlayColor="rgb(210, 230, 255)"
|
|
|
|
style={[styles.row, styles.block]}
|
2016-02-24 12:34:12 +00:00
|
|
|
onPress={() => console.log('custom THW text - highlight')}>
|
2016-02-24 03:38:51 +00:00
|
|
|
<Text style={styles.disabledButton}>
|
|
|
|
Disabled TouchableHighlight
|
|
|
|
</Text>
|
|
|
|
</TouchableHighlight>
|
|
|
|
|
|
|
|
<TouchableHighlight
|
|
|
|
activeOpacity={1}
|
|
|
|
animationVelocity={0}
|
|
|
|
underlayColor="rgb(210, 230, 255)"
|
|
|
|
style={[styles.row, styles.block]}
|
2016-02-24 12:34:12 +00:00
|
|
|
onPress={() => console.log('custom THW text - highlight')}>
|
2016-02-24 03:38:51 +00:00
|
|
|
<Text style={styles.button}>
|
2016-06-04 00:43:26 +00:00
|
|
|
Enabled TouchableHighlight
|
2016-02-24 03:38:51 +00:00
|
|
|
</Text>
|
|
|
|
</TouchableHighlight>
|
2016-02-24 13:25:51 +00:00
|
|
|
|
|
|
|
{Platform.OS === 'android' &&
|
|
|
|
<TouchableNativeFeedback
|
|
|
|
style={[styles.row, styles.block]}
|
|
|
|
onPress={() => console.log('custom TNF has been clicked')}
|
|
|
|
background={TouchableNativeFeedback.SelectableBackground()}>
|
|
|
|
<View>
|
|
|
|
<Text style={[styles.button, styles.nativeFeedbackButton]}>
|
|
|
|
Enabled TouchableNativeFeedback
|
|
|
|
</Text>
|
|
|
|
</View>
|
|
|
|
</TouchableNativeFeedback>
|
|
|
|
}
|
|
|
|
|
|
|
|
{Platform.OS === 'android' &&
|
|
|
|
<TouchableNativeFeedback
|
|
|
|
disabled={true}
|
|
|
|
style={[styles.row, styles.block]}
|
|
|
|
onPress={() => console.log('custom TNF has been clicked')}
|
|
|
|
background={TouchableNativeFeedback.SelectableBackground()}>
|
|
|
|
<View>
|
|
|
|
<Text style={[styles.disabledButton, styles.nativeFeedbackButton]}>
|
|
|
|
Disabled TouchableNativeFeedback
|
|
|
|
</Text>
|
|
|
|
</View>
|
|
|
|
</TouchableNativeFeedback>
|
|
|
|
}
|
2016-02-24 03:38:51 +00:00
|
|
|
</View>
|
|
|
|
);
|
|
|
|
}
|
2016-07-26 08:00:02 +00:00
|
|
|
}
|
2016-02-24 03:38:51 +00:00
|
|
|
|
2015-01-30 01:10:49 +00:00
|
|
|
var heartImage = {uri: 'https://pbs.twimg.com/media/BlXBfT3CQAA6cVZ.png:small'};
|
|
|
|
|
|
|
|
var styles = StyleSheet.create({
|
|
|
|
row: {
|
2015-03-02 18:45:03 +00:00
|
|
|
justifyContent: 'center',
|
2015-01-30 01:10:49 +00:00
|
|
|
flexDirection: 'row',
|
|
|
|
},
|
|
|
|
icon: {
|
|
|
|
width: 24,
|
|
|
|
height: 24,
|
|
|
|
},
|
|
|
|
image: {
|
|
|
|
width: 50,
|
|
|
|
height: 50,
|
|
|
|
},
|
|
|
|
text: {
|
|
|
|
fontSize: 16,
|
|
|
|
},
|
2016-02-24 03:38:51 +00:00
|
|
|
block: {
|
|
|
|
padding: 10,
|
|
|
|
},
|
2015-03-02 18:45:03 +00:00
|
|
|
button: {
|
|
|
|
color: '#007AFF',
|
|
|
|
},
|
2016-02-24 03:38:51 +00:00
|
|
|
disabledButton: {
|
|
|
|
color: '#007AFF',
|
|
|
|
opacity: 0.5,
|
|
|
|
},
|
2016-02-24 13:25:51 +00:00
|
|
|
nativeFeedbackButton: {
|
|
|
|
textAlign: 'center',
|
|
|
|
margin: 10,
|
|
|
|
},
|
2016-02-17 00:50:35 +00:00
|
|
|
hitSlopButton: {
|
|
|
|
color: 'white',
|
|
|
|
},
|
2015-01-30 01:10:49 +00:00
|
|
|
wrapper: {
|
|
|
|
borderRadius: 8,
|
|
|
|
},
|
|
|
|
wrapperCustom: {
|
|
|
|
borderRadius: 8,
|
|
|
|
padding: 6,
|
|
|
|
},
|
2016-02-17 00:50:35 +00:00
|
|
|
hitSlopWrapper: {
|
|
|
|
backgroundColor: 'red',
|
|
|
|
marginVertical: 30,
|
|
|
|
},
|
2015-01-30 01:10:49 +00:00
|
|
|
logBox: {
|
|
|
|
padding: 20,
|
|
|
|
margin: 10,
|
2016-01-15 13:14:27 +00:00
|
|
|
borderWidth: StyleSheet.hairlineWidth,
|
2015-01-30 01:10:49 +00:00
|
|
|
borderColor: '#f0f0f0',
|
|
|
|
backgroundColor: '#f9f9f9',
|
|
|
|
},
|
2015-03-02 18:45:03 +00:00
|
|
|
eventLogBox: {
|
|
|
|
padding: 10,
|
|
|
|
margin: 10,
|
|
|
|
height: 120,
|
2016-01-15 13:14:27 +00:00
|
|
|
borderWidth: StyleSheet.hairlineWidth,
|
2015-03-02 18:45:03 +00:00
|
|
|
borderColor: '#f0f0f0',
|
|
|
|
backgroundColor: '#f9f9f9',
|
|
|
|
},
|
2016-01-27 17:04:14 +00:00
|
|
|
forceTouchBox: {
|
|
|
|
padding: 10,
|
|
|
|
margin: 10,
|
|
|
|
borderWidth: StyleSheet.hairlineWidth,
|
|
|
|
borderColor: '#f0f0f0',
|
|
|
|
backgroundColor: '#f9f9f9',
|
|
|
|
alignItems: 'center',
|
|
|
|
},
|
2015-01-30 01:10:49 +00:00
|
|
|
textBlock: {
|
2015-03-26 01:19:28 +00:00
|
|
|
fontWeight: '500',
|
2015-01-30 01:10:49 +00:00
|
|
|
color: 'blue',
|
|
|
|
},
|
|
|
|
});
|