2015-01-30 01:10:49 +00:00
|
|
|
/**
|
2015-03-23 22:07:33 +00:00
|
|
|
* Copyright (c) 2015-present, Facebook, Inc.
|
|
|
|
*
|
2018-02-17 02:24:55 +00:00
|
|
|
* This source code is licensed under the MIT license found in the
|
|
|
|
* LICENSE file in the root directory of this source tree.
|
2015-01-30 01:10:49 +00:00
|
|
|
*
|
2017-07-12 11:12:02 +00:00
|
|
|
* @flow
|
2017-12-14 01:21:20 +00:00
|
|
|
* @format
|
2015-01-30 01:10:49 +00:00
|
|
|
*/
|
|
|
|
'use strict';
|
|
|
|
|
2017-07-12 11:12:02 +00:00
|
|
|
const ColorPropType = require('ColorPropType');
|
|
|
|
const NativeMethodsMixin = require('NativeMethodsMixin');
|
2017-04-12 23:09:48 +00:00
|
|
|
const PropTypes = require('prop-types');
|
onPress animation with magnification
Summary:
Related to: #15454
Motivation: Improve tvOS feeling for TouchableHighlight
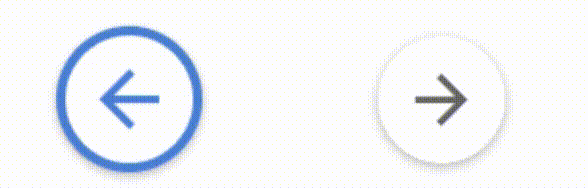
- When you select the button he is focus and the underlay is show
- When you press the button, there is an animation, but after the animation, the focus is on the button and the underlay is show
Play with tvParallaxProperties on tvOS, test with and without patch just to see the actual behaviour
```
<TouchableHighlight
tvParallaxProperties={{
enabled: true,
shiftDistanceX: 0,
shiftDistanceY: 0,
tiltAngle: 0,
magnification: 1.1,
pressMagnification: 1.0,
pressDuration: 0.3,
}}
underlayColor="black"
onShowUnderlay={() => (console.log("onShowUnderlay")}
onHideUnderlay={() => (console.log("onHideUnderlay")}
onPress={() => (console.log("onPress")}
>
<Image
style={styles.image}
source={ uri: 'https://www.facebook.com/images/fb_icon_325x325.png' }
/>
</TouchableHighlight>
```
Closes https://github.com/facebook/react-native/pull/15455
Differential Revision: D6887437
Pulled By: hramos
fbshipit-source-id: e18b695068bc99643ba4006fb3f39215b38a74c1
2018-02-27 20:52:21 +00:00
|
|
|
const Platform = require('Platform');
|
2017-07-12 11:12:02 +00:00
|
|
|
const React = require('React');
|
|
|
|
const ReactNativeViewAttributes = require('ReactNativeViewAttributes');
|
|
|
|
const StyleSheet = require('StyleSheet');
|
|
|
|
const Touchable = require('Touchable');
|
|
|
|
const TouchableWithoutFeedback = require('TouchableWithoutFeedback');
|
|
|
|
const View = require('View');
|
2017-03-24 07:22:57 +00:00
|
|
|
const ViewPropTypes = require('ViewPropTypes');
|
|
|
|
|
2017-07-12 11:12:02 +00:00
|
|
|
const createReactClass = require('create-react-class');
|
|
|
|
const ensurePositiveDelayProps = require('ensurePositiveDelayProps');
|
2015-01-30 01:10:49 +00:00
|
|
|
|
2017-12-19 02:19:22 +00:00
|
|
|
import type {PressEvent} from 'CoreEventTypes';
|
2018-05-13 06:10:46 +00:00
|
|
|
import type {Props as TouchableWithoutFeedbackProps} from 'TouchableWithoutFeedback';
|
|
|
|
import type {ViewStyleProp} from 'StyleSheet';
|
|
|
|
import type {ColorValue} from 'StyleSheetTypes';
|
2015-08-21 08:52:13 +00:00
|
|
|
|
2017-07-12 11:12:02 +00:00
|
|
|
const DEFAULT_PROPS = {
|
2016-09-21 14:00:38 +00:00
|
|
|
activeOpacity: 0.85,
|
2017-12-14 01:21:20 +00:00
|
|
|
delayPressOut: 100,
|
2015-03-09 23:18:15 +00:00
|
|
|
underlayColor: 'black',
|
|
|
|
};
|
|
|
|
|
2017-07-12 11:12:02 +00:00
|
|
|
const PRESS_RETENTION_OFFSET = {top: 20, left: 20, right: 20, bottom: 30};
|
2015-11-16 21:51:13 +00:00
|
|
|
|
2018-05-13 06:10:46 +00:00
|
|
|
type IOSProps = $ReadOnly<{|
|
|
|
|
hasTVPreferredFocus?: ?boolean,
|
|
|
|
tvParallaxProperties?: ?Object,
|
|
|
|
|}>;
|
|
|
|
|
|
|
|
type Props = $ReadOnly<{|
|
|
|
|
...TouchableWithoutFeedbackProps,
|
|
|
|
...IOSProps,
|
|
|
|
|
|
|
|
activeOpacity?: ?number,
|
|
|
|
underlayColor?: ?ColorValue,
|
|
|
|
style?: ?ViewStyleProp,
|
|
|
|
onShowUnderlay?: ?Function,
|
|
|
|
onHideUnderlay?: ?Function,
|
|
|
|
testOnly_pressed?: ?boolean,
|
|
|
|
|}>;
|
|
|
|
|
2015-01-30 01:10:49 +00:00
|
|
|
/**
|
2015-03-09 23:18:15 +00:00
|
|
|
* A wrapper for making views respond properly to touches.
|
2015-01-30 01:10:49 +00:00
|
|
|
* On press down, the opacity of the wrapped view is decreased, which allows
|
2017-02-27 09:49:36 +00:00
|
|
|
* the underlay color to show through, darkening or tinting the view.
|
|
|
|
*
|
|
|
|
* The underlay comes from wrapping the child in a new View, which can affect
|
|
|
|
* layout, and sometimes cause unwanted visual artifacts if not used correctly,
|
|
|
|
* for example if the backgroundColor of the wrapped view isn't explicitly set
|
|
|
|
* to an opaque color.
|
|
|
|
*
|
|
|
|
* TouchableHighlight must have one child (not zero or more than one).
|
|
|
|
* If you wish to have several child components, wrap them in a View.
|
2015-01-30 01:10:49 +00:00
|
|
|
*
|
2015-03-09 23:18:15 +00:00
|
|
|
* Example:
|
2015-01-30 01:10:49 +00:00
|
|
|
*
|
2015-03-09 23:18:15 +00:00
|
|
|
* ```
|
|
|
|
* renderButton: function() {
|
|
|
|
* return (
|
|
|
|
* <TouchableHighlight onPress={this._onPressButton}>
|
|
|
|
* <Image
|
|
|
|
* style={styles.button}
|
2016-07-14 06:11:28 +00:00
|
|
|
* source={require('./myButton.png')}
|
2015-03-09 23:18:15 +00:00
|
|
|
* />
|
2015-03-31 03:12:32 +00:00
|
|
|
* </TouchableHighlight>
|
2015-03-09 23:18:15 +00:00
|
|
|
* );
|
|
|
|
* },
|
|
|
|
* ```
|
2017-09-22 00:31:53 +00:00
|
|
|
*
|
|
|
|
*
|
|
|
|
* ### Example
|
|
|
|
*
|
|
|
|
* ```ReactNativeWebPlayer
|
|
|
|
* import React, { Component } from 'react'
|
|
|
|
* import {
|
|
|
|
* AppRegistry,
|
|
|
|
* StyleSheet,
|
|
|
|
* TouchableHighlight,
|
|
|
|
* Text,
|
|
|
|
* View,
|
|
|
|
* } from 'react-native'
|
|
|
|
*
|
|
|
|
* class App extends Component {
|
|
|
|
* constructor(props) {
|
|
|
|
* super(props)
|
|
|
|
* this.state = { count: 0 }
|
|
|
|
* }
|
|
|
|
*
|
|
|
|
* onPress = () => {
|
|
|
|
* this.setState({
|
|
|
|
* count: this.state.count+1
|
|
|
|
* })
|
|
|
|
* }
|
|
|
|
*
|
|
|
|
* render() {
|
|
|
|
* return (
|
|
|
|
* <View style={styles.container}>
|
|
|
|
* <TouchableHighlight
|
|
|
|
* style={styles.button}
|
|
|
|
* onPress={this.onPress}
|
|
|
|
* >
|
|
|
|
* <Text> Touch Here </Text>
|
|
|
|
* </TouchableHighlight>
|
|
|
|
* <View style={[styles.countContainer]}>
|
|
|
|
* <Text style={[styles.countText]}>
|
|
|
|
* { this.state.count !== 0 ? this.state.count: null}
|
|
|
|
* </Text>
|
|
|
|
* </View>
|
|
|
|
* </View>
|
|
|
|
* )
|
|
|
|
* }
|
|
|
|
* }
|
|
|
|
*
|
|
|
|
* const styles = StyleSheet.create({
|
|
|
|
* container: {
|
|
|
|
* flex: 1,
|
|
|
|
* justifyContent: 'center',
|
|
|
|
* paddingHorizontal: 10
|
|
|
|
* },
|
|
|
|
* button: {
|
|
|
|
* alignItems: 'center',
|
|
|
|
* backgroundColor: '#DDDDDD',
|
|
|
|
* padding: 10
|
|
|
|
* },
|
|
|
|
* countContainer: {
|
|
|
|
* alignItems: 'center',
|
|
|
|
* padding: 10
|
|
|
|
* },
|
|
|
|
* countText: {
|
|
|
|
* color: '#FF00FF'
|
|
|
|
* }
|
|
|
|
* })
|
|
|
|
*
|
|
|
|
* AppRegistry.registerComponent('App', () => App)
|
|
|
|
* ```
|
2017-10-10 00:37:08 +00:00
|
|
|
*
|
2015-01-30 01:10:49 +00:00
|
|
|
*/
|
|
|
|
|
2018-05-13 06:10:46 +00:00
|
|
|
const TouchableHighlight = ((createReactClass({
|
2017-07-07 21:24:25 +00:00
|
|
|
displayName: 'TouchableHighlight',
|
2015-01-30 01:10:49 +00:00
|
|
|
propTypes: {
|
2015-03-18 22:57:49 +00:00
|
|
|
...TouchableWithoutFeedback.propTypes,
|
2015-01-30 01:10:49 +00:00
|
|
|
/**
|
|
|
|
* Determines what the opacity of the wrapped view should be when touch is
|
|
|
|
* active.
|
|
|
|
*/
|
2017-04-12 23:09:48 +00:00
|
|
|
activeOpacity: PropTypes.number,
|
2015-01-30 01:10:49 +00:00
|
|
|
/**
|
|
|
|
* The color of the underlay that will show through when the touch is
|
|
|
|
* active.
|
|
|
|
*/
|
2015-12-23 03:29:01 +00:00
|
|
|
underlayColor: ColorPropType,
|
2017-12-14 01:21:20 +00:00
|
|
|
/**
|
|
|
|
* Style to apply to the container/underlay. Most commonly used to make sure
|
|
|
|
* rounded corners match the wrapped component.
|
|
|
|
*/
|
2017-03-24 07:22:57 +00:00
|
|
|
style: ViewPropTypes.style,
|
2015-05-26 22:16:42 +00:00
|
|
|
/**
|
|
|
|
* Called immediately after the underlay is shown
|
|
|
|
*/
|
2017-04-12 23:09:48 +00:00
|
|
|
onShowUnderlay: PropTypes.func,
|
2015-05-26 22:16:42 +00:00
|
|
|
/**
|
|
|
|
* Called immediately after the underlay is hidden
|
|
|
|
*/
|
2017-04-12 23:09:48 +00:00
|
|
|
onHideUnderlay: PropTypes.func,
|
2016-12-19 14:26:07 +00:00
|
|
|
/**
|
|
|
|
* *(Apple TV only)* TV preferred focus (see documentation for the View component).
|
|
|
|
*
|
|
|
|
* @platform ios
|
|
|
|
*/
|
2017-04-12 23:09:48 +00:00
|
|
|
hasTVPreferredFocus: PropTypes.bool,
|
2016-12-19 14:26:07 +00:00
|
|
|
/**
|
|
|
|
* *(Apple TV only)* Object with properties to control Apple TV parallax effects.
|
|
|
|
*
|
|
|
|
* enabled: If true, parallax effects are enabled. Defaults to true.
|
|
|
|
* shiftDistanceX: Defaults to 2.0.
|
|
|
|
* shiftDistanceY: Defaults to 2.0.
|
|
|
|
* tiltAngle: Defaults to 0.05.
|
|
|
|
* magnification: Defaults to 1.0.
|
onPress animation with magnification
Summary:
Related to: #15454
Motivation: Improve tvOS feeling for TouchableHighlight
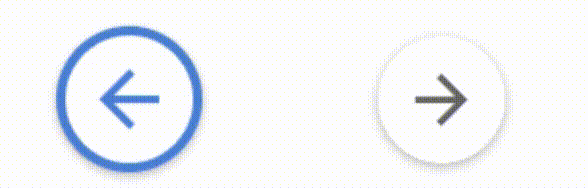
- When you select the button he is focus and the underlay is show
- When you press the button, there is an animation, but after the animation, the focus is on the button and the underlay is show
Play with tvParallaxProperties on tvOS, test with and without patch just to see the actual behaviour
```
<TouchableHighlight
tvParallaxProperties={{
enabled: true,
shiftDistanceX: 0,
shiftDistanceY: 0,
tiltAngle: 0,
magnification: 1.1,
pressMagnification: 1.0,
pressDuration: 0.3,
}}
underlayColor="black"
onShowUnderlay={() => (console.log("onShowUnderlay")}
onHideUnderlay={() => (console.log("onHideUnderlay")}
onPress={() => (console.log("onPress")}
>
<Image
style={styles.image}
source={ uri: 'https://www.facebook.com/images/fb_icon_325x325.png' }
/>
</TouchableHighlight>
```
Closes https://github.com/facebook/react-native/pull/15455
Differential Revision: D6887437
Pulled By: hramos
fbshipit-source-id: e18b695068bc99643ba4006fb3f39215b38a74c1
2018-02-27 20:52:21 +00:00
|
|
|
* pressMagnification: Defaults to 1.0.
|
|
|
|
* pressDuration: Defaults to 0.3.
|
|
|
|
* pressDelay: Defaults to 0.0.
|
2016-12-19 14:26:07 +00:00
|
|
|
*
|
|
|
|
* @platform ios
|
|
|
|
*/
|
2017-04-12 23:09:48 +00:00
|
|
|
tvParallaxProperties: PropTypes.object,
|
2018-02-26 21:41:48 +00:00
|
|
|
/**
|
|
|
|
* Handy for snapshot tests.
|
|
|
|
*/
|
|
|
|
testOnly_pressed: PropTypes.bool,
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
2017-12-14 01:21:20 +00:00
|
|
|
mixins: [NativeMethodsMixin, Touchable.Mixin],
|
2015-01-30 01:10:49 +00:00
|
|
|
|
|
|
|
getDefaultProps: () => DEFAULT_PROPS,
|
|
|
|
|
|
|
|
getInitialState: function() {
|
2017-05-08 17:52:31 +00:00
|
|
|
this._isMounted = false;
|
2018-02-26 21:41:48 +00:00
|
|
|
if (this.props.testOnly_pressed) {
|
|
|
|
return {
|
|
|
|
...this.touchableGetInitialState(),
|
|
|
|
extraChildStyle: {
|
|
|
|
opacity: this.props.activeOpacity,
|
|
|
|
},
|
|
|
|
extraUnderlayStyle: {
|
|
|
|
backgroundColor: this.props.underlayColor,
|
|
|
|
},
|
|
|
|
};
|
|
|
|
} else {
|
|
|
|
return {
|
|
|
|
...this.touchableGetInitialState(),
|
|
|
|
extraChildStyle: null,
|
|
|
|
extraUnderlayStyle: null,
|
|
|
|
};
|
|
|
|
}
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
|
|
|
componentDidMount: function() {
|
2017-05-08 17:52:31 +00:00
|
|
|
this._isMounted = true;
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
ensurePositiveDelayProps(this.props);
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
2017-05-10 03:50:46 +00:00
|
|
|
componentWillUnmount: function() {
|
2017-05-08 17:52:31 +00:00
|
|
|
this._isMounted = false;
|
2017-12-14 01:21:20 +00:00
|
|
|
clearTimeout(this._hideTimeout);
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
2018-02-08 18:26:45 +00:00
|
|
|
UNSAFE_componentWillReceiveProps: function(nextProps) {
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
ensurePositiveDelayProps(nextProps);
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
|
|
|
viewConfig: {
|
|
|
|
uiViewClassName: 'RCTView',
|
2017-12-14 01:21:20 +00:00
|
|
|
validAttributes: ReactNativeViewAttributes.RCTView,
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
|
|
|
/**
|
|
|
|
* `Touchable.Mixin` self callbacks. The mixin will invoke these if they are
|
|
|
|
* defined on your component.
|
|
|
|
*/
|
2017-12-19 02:19:22 +00:00
|
|
|
touchableHandleActivePressIn: function(e: PressEvent) {
|
2017-12-14 01:21:20 +00:00
|
|
|
clearTimeout(this._hideTimeout);
|
2015-01-30 01:10:49 +00:00
|
|
|
this._hideTimeout = null;
|
|
|
|
this._showUnderlay();
|
2015-08-21 08:52:13 +00:00
|
|
|
this.props.onPressIn && this.props.onPressIn(e);
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
2017-12-19 02:19:22 +00:00
|
|
|
touchableHandleActivePressOut: function(e: PressEvent) {
|
2015-01-30 01:10:49 +00:00
|
|
|
if (!this._hideTimeout) {
|
|
|
|
this._hideUnderlay();
|
|
|
|
}
|
2015-08-21 08:52:13 +00:00
|
|
|
this.props.onPressOut && this.props.onPressOut(e);
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
2017-12-19 02:19:22 +00:00
|
|
|
touchableHandlePress: function(e: PressEvent) {
|
2017-12-14 01:21:20 +00:00
|
|
|
clearTimeout(this._hideTimeout);
|
onPress animation with magnification
Summary:
Related to: #15454
Motivation: Improve tvOS feeling for TouchableHighlight
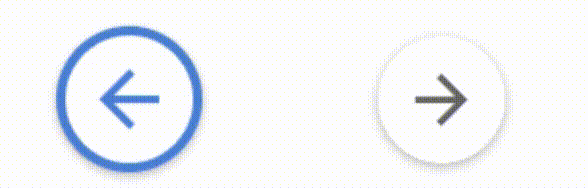
- When you select the button he is focus and the underlay is show
- When you press the button, there is an animation, but after the animation, the focus is on the button and the underlay is show
Play with tvParallaxProperties on tvOS, test with and without patch just to see the actual behaviour
```
<TouchableHighlight
tvParallaxProperties={{
enabled: true,
shiftDistanceX: 0,
shiftDistanceY: 0,
tiltAngle: 0,
magnification: 1.1,
pressMagnification: 1.0,
pressDuration: 0.3,
}}
underlayColor="black"
onShowUnderlay={() => (console.log("onShowUnderlay")}
onHideUnderlay={() => (console.log("onHideUnderlay")}
onPress={() => (console.log("onPress")}
>
<Image
style={styles.image}
source={ uri: 'https://www.facebook.com/images/fb_icon_325x325.png' }
/>
</TouchableHighlight>
```
Closes https://github.com/facebook/react-native/pull/15455
Differential Revision: D6887437
Pulled By: hramos
fbshipit-source-id: e18b695068bc99643ba4006fb3f39215b38a74c1
2018-02-27 20:52:21 +00:00
|
|
|
if (!Platform.isTVOS) {
|
|
|
|
this._showUnderlay();
|
|
|
|
this._hideTimeout = setTimeout(
|
|
|
|
this._hideUnderlay,
|
|
|
|
this.props.delayPressOut,
|
|
|
|
);
|
|
|
|
}
|
2015-08-21 08:52:13 +00:00
|
|
|
this.props.onPress && this.props.onPress(e);
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
2017-12-19 02:19:22 +00:00
|
|
|
touchableHandleLongPress: function(e: PressEvent) {
|
2015-08-21 08:52:13 +00:00
|
|
|
this.props.onLongPress && this.props.onLongPress(e);
|
2015-03-02 18:45:03 +00:00
|
|
|
},
|
|
|
|
|
2015-01-30 01:10:49 +00:00
|
|
|
touchableGetPressRectOffset: function() {
|
2015-11-16 21:51:13 +00:00
|
|
|
return this.props.pressRetentionOffset || PRESS_RETENTION_OFFSET;
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
2016-02-17 00:50:35 +00:00
|
|
|
touchableGetHitSlop: function() {
|
|
|
|
return this.props.hitSlop;
|
|
|
|
},
|
|
|
|
|
[Touchable] Add custom delay props to Touchable components
Summary:
@public
This PR adds quite a bit of functionality to the Touchable components, allowing the ms delays of each of the handlers (`onPressIn, onPressOut, onPress, onLongPress`) to be configured.
It adds the following props to `TouchableWithoutFeedback, TouchableOpacity, and TouchableHighlight`:
```javascript
/**
* Delay in ms, from the release of the touch, before onPress is called.
*/
delayOnPress: React.PropTypes.number,
/**
* Delay in ms, from the start of the touch, before onPressIn is called.
*/
delayOnPressIn: React.PropTypes.number,
/**
* Delay in ms, from the release of the touch, before onPressOut is called.
*/
delayOnPressOut: React.PropTypes.number,
/**
* Delay in ms, from onPressIn, before onLongPress is called.
*/
delayOnLongPress: React.PropTypes.number,
```
`TouchableHighlight` also gets an additional set of props:
```javascript
/**
* Delay in ms, from the start of the touch, before the highlight is shown.
*/
delayHighlightShow: React.PropTypes.number,
/**
* Del
...
```
Closes https://github.com/facebook/react-native/pull/1255
Github Author: jmstout <git@jmstout.com>
Test Plan: Imported from GitHub, without a `Test Plan:` line.
2015-06-03 19:56:32 +00:00
|
|
|
touchableGetHighlightDelayMS: function() {
|
|
|
|
return this.props.delayPressIn;
|
|
|
|
},
|
|
|
|
|
|
|
|
touchableGetLongPressDelayMS: function() {
|
|
|
|
return this.props.delayLongPress;
|
|
|
|
},
|
|
|
|
|
|
|
|
touchableGetPressOutDelayMS: function() {
|
|
|
|
return this.props.delayPressOut;
|
|
|
|
},
|
|
|
|
|
2015-01-30 01:10:49 +00:00
|
|
|
_showUnderlay: function() {
|
2017-05-08 17:52:31 +00:00
|
|
|
if (!this._isMounted || !this._hasPressHandler()) {
|
2015-07-17 23:47:36 +00:00
|
|
|
return;
|
|
|
|
}
|
2017-12-14 01:21:20 +00:00
|
|
|
this.setState({
|
|
|
|
extraChildStyle: {
|
|
|
|
opacity: this.props.activeOpacity,
|
|
|
|
},
|
|
|
|
extraUnderlayStyle: {
|
|
|
|
backgroundColor: this.props.underlayColor,
|
|
|
|
},
|
|
|
|
});
|
2015-05-26 22:16:42 +00:00
|
|
|
this.props.onShowUnderlay && this.props.onShowUnderlay();
|
2015-01-30 01:10:49 +00:00
|
|
|
},
|
|
|
|
|
|
|
|
_hideUnderlay: function() {
|
2017-12-14 01:21:20 +00:00
|
|
|
clearTimeout(this._hideTimeout);
|
2015-01-30 01:10:49 +00:00
|
|
|
this._hideTimeout = null;
|
2018-02-26 21:41:48 +00:00
|
|
|
if (this.props.testOnly_pressed) {
|
|
|
|
return;
|
|
|
|
}
|
2017-12-14 01:21:20 +00:00
|
|
|
if (this._hasPressHandler()) {
|
|
|
|
this.setState({
|
|
|
|
extraChildStyle: null,
|
2017-12-15 05:06:24 +00:00
|
|
|
extraUnderlayStyle: null,
|
2015-03-18 22:57:49 +00:00
|
|
|
});
|
2015-05-26 22:16:42 +00:00
|
|
|
this.props.onHideUnderlay && this.props.onHideUnderlay();
|
2015-01-30 01:10:49 +00:00
|
|
|
}
|
|
|
|
},
|
|
|
|
|
2016-02-12 19:32:06 +00:00
|
|
|
_hasPressHandler: function() {
|
|
|
|
return !!(
|
2016-02-15 23:13:42 +00:00
|
|
|
this.props.onPress ||
|
|
|
|
this.props.onPressIn ||
|
|
|
|
this.props.onPressOut ||
|
2016-02-12 19:32:06 +00:00
|
|
|
this.props.onLongPress
|
|
|
|
);
|
|
|
|
},
|
|
|
|
|
2015-01-30 01:10:49 +00:00
|
|
|
render: function() {
|
2017-12-14 01:21:20 +00:00
|
|
|
const child = React.Children.only(this.props.children);
|
2015-01-30 01:10:49 +00:00
|
|
|
return (
|
|
|
|
<View
|
2016-06-21 15:24:07 +00:00
|
|
|
accessible={this.props.accessible !== false}
|
2016-02-04 13:12:36 +00:00
|
|
|
accessibilityLabel={this.props.accessibilityLabel}
|
2015-09-03 19:19:15 +00:00
|
|
|
accessibilityComponentType={this.props.accessibilityComponentType}
|
|
|
|
accessibilityTraits={this.props.accessibilityTraits}
|
2017-12-14 01:21:20 +00:00
|
|
|
style={StyleSheet.compose(
|
|
|
|
this.props.style,
|
|
|
|
this.state.extraUnderlayStyle,
|
|
|
|
)}
|
2015-09-01 17:31:20 +00:00
|
|
|
onLayout={this.props.onLayout}
|
2016-02-17 00:50:35 +00:00
|
|
|
hitSlop={this.props.hitSlop}
|
2016-12-19 14:26:07 +00:00
|
|
|
isTVSelectable={true}
|
|
|
|
tvParallaxProperties={this.props.tvParallaxProperties}
|
2017-12-14 01:21:20 +00:00
|
|
|
hasTVPreferredFocus={this.props.hasTVPreferredFocus}
|
2015-01-30 01:10:49 +00:00
|
|
|
onStartShouldSetResponder={this.touchableHandleStartShouldSetResponder}
|
2017-12-14 01:21:20 +00:00
|
|
|
onResponderTerminationRequest={
|
|
|
|
this.touchableHandleResponderTerminationRequest
|
|
|
|
}
|
2015-01-30 01:10:49 +00:00
|
|
|
onResponderGrant={this.touchableHandleResponderGrant}
|
|
|
|
onResponderMove={this.touchableHandleResponderMove}
|
|
|
|
onResponderRelease={this.touchableHandleResponderRelease}
|
2015-08-11 17:41:15 +00:00
|
|
|
onResponderTerminate={this.touchableHandleResponderTerminate}
|
2017-04-07 18:47:35 +00:00
|
|
|
nativeID={this.props.nativeID}
|
2015-08-11 17:41:15 +00:00
|
|
|
testID={this.props.testID}>
|
2017-12-14 01:21:20 +00:00
|
|
|
{React.cloneElement(child, {
|
|
|
|
style: StyleSheet.compose(
|
|
|
|
child.props.style,
|
|
|
|
this.state.extraChildStyle,
|
|
|
|
),
|
|
|
|
})}
|
|
|
|
{Touchable.renderDebugView({
|
|
|
|
color: 'green',
|
|
|
|
hitSlop: this.props.hitSlop,
|
|
|
|
})}
|
2015-01-30 01:10:49 +00:00
|
|
|
</View>
|
|
|
|
);
|
2017-12-14 01:21:20 +00:00
|
|
|
},
|
2018-05-13 06:10:46 +00:00
|
|
|
}): any): React.ComponentType<Props>);
|
2015-01-30 01:10:49 +00:00
|
|
|
|
|
|
|
module.exports = TouchableHighlight;
|