2013-01-27 16:06:58 +00:00
|
|
|
# blessed
|
|
|
|
|
|
|
|
A curses-like library for node.js.
|
|
|
|
|
2013-07-16 01:33:47 +00:00
|
|
|
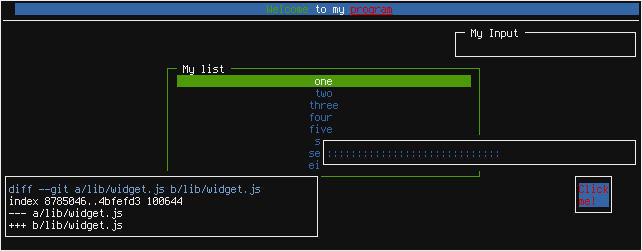
|
|
|
|
|
2013-07-17 00:12:08 +00:00
|
|
|
## Install
|
|
|
|
|
|
|
|
``` bash
|
2013-07-16 05:18:31 +00:00
|
|
|
$ npm install blessed
|
2013-07-17 00:12:08 +00:00
|
|
|
```
|
2013-07-16 05:18:31 +00:00
|
|
|
|
2013-07-15 22:45:08 +00:00
|
|
|
## Example
|
2013-01-27 17:14:06 +00:00
|
|
|
|
2013-07-16 22:50:02 +00:00
|
|
|
This will render a box with line borders containing the text `'Hello world!'`,
|
2013-06-14 00:02:02 +00:00
|
|
|
perfectly centered horizontally and vertically.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
``` js
|
2013-07-15 22:45:08 +00:00
|
|
|
var blessed = require('blessed');
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-07-15 22:45:08 +00:00
|
|
|
// Create a screen object.
|
|
|
|
var screen = blessed.screen();
|
|
|
|
|
|
|
|
// Create a box perfectly centered horizontally and vertically.
|
|
|
|
var box = blessed.box({
|
2013-06-11 16:48:37 +00:00
|
|
|
top: 'center',
|
|
|
|
left: 'center',
|
|
|
|
width: '50%',
|
|
|
|
height: '50%',
|
|
|
|
border: {
|
2013-07-16 22:50:02 +00:00
|
|
|
type: 'line',
|
2013-07-15 22:45:08 +00:00
|
|
|
fg: '#ffffff'
|
2013-06-11 16:48:37 +00:00
|
|
|
},
|
2013-06-14 00:02:02 +00:00
|
|
|
fg: 'white',
|
|
|
|
bg: 'magenta',
|
2013-07-15 22:45:08 +00:00
|
|
|
content: 'Hello {bold}world{/bold}!',
|
|
|
|
tags: true,
|
|
|
|
hoverEffects: {
|
|
|
|
bg: 'green'
|
|
|
|
}
|
2013-06-24 11:16:02 +00:00
|
|
|
});
|
|
|
|
|
2013-07-15 22:45:08 +00:00
|
|
|
// Append our box to the screen.
|
2013-06-24 11:16:02 +00:00
|
|
|
screen.append(box);
|
|
|
|
|
2013-07-15 22:45:08 +00:00
|
|
|
// If our box is clicked, change the content.
|
2013-06-24 11:16:02 +00:00
|
|
|
box.on('click', function(data) {
|
|
|
|
box.setContent('{center}Some different {red-fg}content{/red-fg}.{/center}');
|
2013-06-30 17:17:27 +00:00
|
|
|
screen.render();
|
2013-06-24 11:16:02 +00:00
|
|
|
});
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-07-15 22:45:08 +00:00
|
|
|
// If box is focused, handle `enter` and give us some more content.
|
|
|
|
box.key('enter', function() {
|
|
|
|
box.setContent('{right}Even different {black-fg}content{/black-fg}.{/right}\n');
|
|
|
|
box.setLine(1, 'bar');
|
|
|
|
box.insertLine(1, 'foo');
|
|
|
|
screen.render();
|
|
|
|
});
|
|
|
|
|
|
|
|
// Quit on Escape, q, or Control-C.
|
|
|
|
screen.key(['escape', 'q', 'C-c'], function(ch, key) {
|
2013-07-12 09:59:58 +00:00
|
|
|
return process.exit(0);
|
2013-06-11 16:48:37 +00:00
|
|
|
});
|
|
|
|
|
2013-07-15 22:45:08 +00:00
|
|
|
// Focus our element.
|
|
|
|
box.focus();
|
|
|
|
|
|
|
|
// Render the screen.
|
2013-06-11 16:48:37 +00:00
|
|
|
screen.render();
|
|
|
|
```
|
|
|
|
|
2013-07-03 23:46:06 +00:00
|
|
|
|
2013-07-15 22:45:08 +00:00
|
|
|
## High-level Documentation
|
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
### Widgets
|
|
|
|
|
|
|
|
Blessed comes with a number of high-level widgets so you can avoid all the
|
|
|
|
nasty low-level terminal stuff.
|
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### Node (from EventEmitter)
|
|
|
|
|
|
|
|
The base node which everything inherits from.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
- **screen** - the screen to be associated with.
|
|
|
|
- **parent** - the desired parent.
|
|
|
|
- **children** - an arrray of children.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Properties:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from EventEmitter.
|
2013-07-17 05:53:34 +00:00
|
|
|
- **type** - type of the node (e.g. `box`).
|
2013-06-18 11:30:26 +00:00
|
|
|
- **options** - original options object.
|
|
|
|
- **parent** - parent node.
|
|
|
|
- **screen** - parent screen.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **children** - array of node's children.
|
2013-06-18 11:30:26 +00:00
|
|
|
- **data, _, $** - an object for any miscellanous user data.
|
2013-07-12 03:52:46 +00:00
|
|
|
- **index** - render index (document order index) of the last render call.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Events:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from EventEmitter.
|
2013-06-11 18:13:49 +00:00
|
|
|
- **adopt** - received when node is added to a parent.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **remove** - received when node is removed from it's current parent.
|
|
|
|
- **reparent** - received when node gains a new parent.
|
2013-06-20 11:43:56 +00:00
|
|
|
- **attach** - received when node is attached to the screen directly or
|
|
|
|
somewhere in its ancestry.
|
|
|
|
- **detach** - received when node is detached from the screen directly or
|
|
|
|
somewhere in its ancestry.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Methods:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from EventEmitter.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **prepend(node)** - prepend a node to this node's children.
|
|
|
|
- **append(node)** - append a node to this node's children.
|
|
|
|
- **remove(node)** - remove child node from node.
|
2013-07-15 06:44:11 +00:00
|
|
|
- **insert(node, i)** - insert a node to this node's children at index `i`.
|
|
|
|
- **insertBefore(node, refNode)** - insert a node to this node's children
|
|
|
|
before the reference node.
|
|
|
|
- **insertAfter(node, refNode)** - insert a node from node after the reference
|
|
|
|
node.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **detach()** - remove node from its parent.
|
2013-06-20 12:18:04 +00:00
|
|
|
- **emitDescendants(type, args..., [iterator])** - emit event for element, and
|
|
|
|
recursively emit same event for all descendants.
|
2013-07-12 09:59:58 +00:00
|
|
|
- **get(name, [default])** - get user property with a potential default value.
|
|
|
|
- **set(name, value)** - set user property to value.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### Screen (from Node)
|
|
|
|
|
|
|
|
The screen on which every other node renders.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
- **program** - the blessed Program to be associated with.
|
2013-07-15 06:44:11 +00:00
|
|
|
- **smartCSR** - attempt to perform CSR optimization on all possible elements
|
|
|
|
(not just full-width ones, elements with uniform cells to their sides).
|
|
|
|
this is known to cause flickering with elements that are not full-width,
|
|
|
|
however, it is more optimal for terminal rendering.
|
2013-07-23 10:04:04 +00:00
|
|
|
- **useBCE** - attempt to perform `back_color_erase` optimizations for terminals
|
|
|
|
that support it. it will also work with terminals that don't support it, but
|
|
|
|
only on lines with the default background color. as it stands with the current
|
|
|
|
implementation, it's uncertain how much terminal performance this adds at the
|
|
|
|
cost of overhead within node.
|
2013-07-17 06:32:49 +00:00
|
|
|
- **resizeTimeout** - amount of time (in ms) to redraw the screen after the
|
|
|
|
terminal is resized (default: 300).
|
2013-07-25 06:20:47 +00:00
|
|
|
- **tabSize** - the width of tabs within an element's content.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Properties:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Node.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **program** - the blessed Program object.
|
2013-06-13 21:49:48 +00:00
|
|
|
- **tput** - the blessed Tput object (only available if you passed `tput: true`
|
|
|
|
to the Program constructor.)
|
2013-06-11 17:52:18 +00:00
|
|
|
- **focused** - top of the focus history stack.
|
|
|
|
- **width** - width of the screen (same as `program.cols`).
|
|
|
|
- **height** - height of the screen (same as `program.rows`).
|
2013-06-13 21:49:48 +00:00
|
|
|
- **cols** - same as `screen.width`.
|
|
|
|
- **rows** - same as `screen.height`.
|
|
|
|
- **left**, **rleft** - left offset, always zero.
|
|
|
|
- **right**, **rright** - right offset, always zero.
|
|
|
|
- **top**, **rtop** - top offset, always zero.
|
|
|
|
- **bottom**, **rbottom** - bottom offset, always zero.
|
2013-06-18 15:52:48 +00:00
|
|
|
- **grabKeys** - whether the focused element grabs all keypresses.
|
|
|
|
- **lockKeys** - prevent keypresses from being received by any element.
|
2013-07-03 23:46:06 +00:00
|
|
|
- **hover** - the currently hovered element. only set if mouse events are bound.
|
2013-06-11 17:52:18 +00:00
|
|
|
|
|
|
|
##### Events:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Node.
|
2013-06-16 14:31:55 +00:00
|
|
|
- **resize** - received on screen resize.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **mouse** - received on mouse events.
|
|
|
|
- **keypress** - received on key events.
|
|
|
|
- **element [name]** - global events received for all elements.
|
2013-07-04 04:15:09 +00:00
|
|
|
- **key [name]** - received on key event for [name].
|
2013-07-15 23:53:04 +00:00
|
|
|
- **focus, blur** - received when the terminal window focuses/blurs. requires a
|
|
|
|
terminal supporting the focus protocol and focus needs to be passed to
|
2013-07-14 04:38:43 +00:00
|
|
|
program.enableMouse().
|
2013-07-15 23:53:04 +00:00
|
|
|
- **prerender** - received before render.
|
|
|
|
- **render** - received on render.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Methods:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Node.
|
2013-06-25 23:17:21 +00:00
|
|
|
- **alloc()** - allocate a new pending screen buffer and a new output screen
|
|
|
|
buffer.
|
|
|
|
- **draw(start, end)** - draw the screen based on the contents of the screen
|
|
|
|
buffer.
|
|
|
|
- **render()** - render all child elements, writing all data to the screen
|
|
|
|
buffer and drawing the screen.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **clearRegion(x1, x2, y1, y2)** - clear any region on the screen.
|
2013-06-25 23:17:21 +00:00
|
|
|
- **fillRegion(attr, ch, x1, x2, y1, y2)** - fill any region with a character
|
|
|
|
of a certain attribute.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **focus(offset)** - focus element by offset of focusable elements.
|
|
|
|
- **focusPrev()** - focus previous element in the index.
|
|
|
|
- **focusNext()** - focus next element in the index.
|
2013-06-25 23:17:21 +00:00
|
|
|
- **focusPush(element)** - push element on the focus stack (equivalent to
|
|
|
|
`screen.focused = el`).
|
2013-06-11 17:52:18 +00:00
|
|
|
- **focusPop()/focusLast()** - pop element off the focus stack.
|
2013-06-20 18:03:31 +00:00
|
|
|
- **saveFocus()** - save the focused element.
|
|
|
|
- **restoreFocus()** - restore the saved focused element.
|
2013-07-04 04:15:09 +00:00
|
|
|
- **key(name, listener)** - bind a keypress listener for a specific key.
|
2013-07-12 09:59:58 +00:00
|
|
|
- **onceKey(name, listener)** - bind a keypress listener for a specific key
|
|
|
|
once.
|
|
|
|
- **unkey(name, listener)** - remove a keypress listener for a specific key.
|
2013-07-04 04:15:09 +00:00
|
|
|
- **spawn(file, args, options)** - spawn a process in the foreground, return to
|
|
|
|
blessed app after exit.
|
|
|
|
- **exec(file, args, options, callback)** - spawn a process in the foreground,
|
|
|
|
return to blessed app after exit. executes callback on error or exit.
|
2013-07-04 05:10:01 +00:00
|
|
|
- **readEditor([options], callback)** - read data from text editor.
|
2013-07-12 09:59:58 +00:00
|
|
|
- **setEffects(el, fel, over, out, effects, temp)** - set effects based on
|
|
|
|
two events and attributes.
|
|
|
|
- **insertLine(n, y, top, bottom)** - insert a line into the screen (using csr:
|
|
|
|
this bypasses the output buffer).
|
|
|
|
- **deleteLine(n, y, top, bottom)** - delete a line from the screen (using csr:
|
|
|
|
this bypasses the output buffer).
|
|
|
|
- **insertBottom(top, bottom)** - insert a line at the bottom of the screen.
|
|
|
|
- **insertTop(top, bottom)** - insert a line at the top of the screen.
|
|
|
|
- **deleteBottom(top, bottom)** - delete a line at the bottom of the screen.
|
|
|
|
- **deleteTop(top, bottom)** - delete a line at the top of the screen.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### Element (from Node)
|
|
|
|
|
|
|
|
The base element.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
- **fg, bg, bold, underline** - attributes.
|
2013-07-17 03:28:56 +00:00
|
|
|
- **style** - may contain attributes in the format of:
|
|
|
|
``` js
|
|
|
|
{
|
|
|
|
fg: 'blue',
|
|
|
|
bg: 'black',
|
|
|
|
border: {
|
|
|
|
fg: 'blue'
|
|
|
|
},
|
|
|
|
scrollbar: {
|
|
|
|
bg: 'blue'
|
2013-07-17 06:37:57 +00:00
|
|
|
},
|
|
|
|
focus: {
|
|
|
|
bg: 'red'
|
|
|
|
},
|
|
|
|
hover: {
|
|
|
|
bg: 'red'
|
2013-07-17 03:28:56 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
```
|
2013-06-11 17:52:18 +00:00
|
|
|
- **border** - border object, see below.
|
|
|
|
- **content** - element's text content.
|
|
|
|
- **clickable** - element is clickable.
|
|
|
|
- **input** - element is focusable and can receive key input.
|
|
|
|
- **hidden** - whether the element is hidden.
|
|
|
|
- **label** - a simple text label for the element.
|
2013-06-13 09:14:14 +00:00
|
|
|
- **align** - text alignment: `left`, `center`, or `right`.
|
2013-07-17 03:28:56 +00:00
|
|
|
- **valign** - vertical text alignment: `top`, `middle`, or `bottom`.
|
2013-07-17 09:20:22 +00:00
|
|
|
- **shrink** - shrink/flex/grow to content and child elements. width/height
|
|
|
|
during render.
|
2013-07-17 03:28:56 +00:00
|
|
|
- **padding** - amount of padding on the inside of the element.
|
2013-07-17 09:20:22 +00:00
|
|
|
- **width, height** - width/height of the element, can be a number, percentage
|
|
|
|
(`0-100%`), or keyword (`half` or `shrink`).
|
|
|
|
- **left, right, top, bottom** - offsets of the element **relative to its
|
|
|
|
parent**. can be a number, percentage (`0-100%`), or keyword (`center`).
|
2013-07-19 01:55:16 +00:00
|
|
|
`right` and `bottom` do not accept keywords.
|
2013-07-23 11:54:21 +00:00
|
|
|
- **position** - can contain the above options.
|
2013-07-25 06:20:47 +00:00
|
|
|
- **scrollable** - whether the element is scrollable or not.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Properties:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Node.
|
2013-07-17 09:20:22 +00:00
|
|
|
- **name** - name of the element. useful for form submission.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **border** - border object.
|
2013-07-16 22:50:02 +00:00
|
|
|
- **type** - type of border (`line` or `bg`). `bg` by default.
|
2013-06-11 17:53:28 +00:00
|
|
|
- **ch** - character to use if `bg` type, default is space.
|
2013-06-25 23:17:21 +00:00
|
|
|
- **bg, fg** - border foreground and background, must be numbers (-1 for
|
|
|
|
default).
|
2013-06-11 17:52:18 +00:00
|
|
|
- **bold, underline** - border attributes.
|
2013-07-17 09:38:11 +00:00
|
|
|
- **style** - contains attributes (e.g. `fg/bg/underline`). see above.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **position** - raw width, height, and offsets.
|
2013-07-23 23:37:56 +00:00
|
|
|
- **content** - raw text content.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **hidden** - whether the element is hidden or not.
|
2013-07-17 09:38:11 +00:00
|
|
|
- **visible** - whether the element is visible or not.
|
|
|
|
- **detached** - whether the element is attached to a screen in its ancestry
|
|
|
|
somewhere.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **fg, bg** - foreground and background, must be numbers (-1 for default).
|
|
|
|
- **bold, underline** - attributes.
|
|
|
|
- **width** - calculated width.
|
|
|
|
- **height** - calculated height.
|
|
|
|
- **left** - calculated absolute left offset.
|
|
|
|
- **right** - calculated absolute right offset.
|
|
|
|
- **top** - calculated absolute top offset.
|
|
|
|
- **bottom** - calculated absolute bottom offset.
|
|
|
|
- **rleft** - calculated relative left offset.
|
|
|
|
- **rright** - calculated relative right offset.
|
|
|
|
- **rtop** - calculated relative top offset.
|
|
|
|
- **rbottom** - calculated relative bottom offset.
|
|
|
|
|
|
|
|
##### Events:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Node.
|
2013-06-16 14:31:55 +00:00
|
|
|
- **blur, focus** - received when an element is focused or unfocused.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **mouse** - received on mouse events for this element.
|
2013-06-16 14:31:55 +00:00
|
|
|
- **mousedown, mouseup** - mouse button was pressed or released.
|
|
|
|
- **wheeldown, wheelup** - wheel was scrolled down or up.
|
|
|
|
- **mouseover, mouseout** - element was hovered or unhovered.
|
|
|
|
- **mousemove** - mouse was moved somewhere on this element.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **keypress** - received on key events for this element.
|
|
|
|
- **move** - received when the element is moved.
|
|
|
|
- **resize** - received when the element is resized.
|
2013-07-04 04:15:09 +00:00
|
|
|
- **key [name]** - received on key event for [name].
|
2013-07-25 06:20:47 +00:00
|
|
|
- **prerender** - received before a call to render.
|
|
|
|
- **render** - received after a call to render.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Methods:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Node.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **render()** - write content and children to the screen buffer.
|
|
|
|
- **hide()** - hide element.
|
|
|
|
- **show()** - show element.
|
|
|
|
- **toggle()** - toggle hidden/shown.
|
|
|
|
- **focus()** - focus element.
|
2013-07-04 04:15:09 +00:00
|
|
|
- **key(name, listener)** - bind a keypress listener for a specific key.
|
2013-07-12 09:59:58 +00:00
|
|
|
- **onceKey(name, listener)** - bind a keypress listener for a specific key
|
|
|
|
once.
|
|
|
|
- **unkey(name, listener)** - remove a keypress listener for a specific key.
|
|
|
|
- **onScreenEvent(type, listener)** - same as`el.on('screen', ...)` except this
|
|
|
|
will automatically cleanup listeners after the element is detached.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-07-23 23:37:56 +00:00
|
|
|
###### Content Methods
|
|
|
|
|
|
|
|
Methods for dealing with text content, line by line. Useful for writing a
|
|
|
|
text editor, irc client, etc.
|
|
|
|
|
|
|
|
Note: all of these methods deal with pre-aligned, pre-wrapped text. If you use
|
|
|
|
deleteTop() on a box with a wrapped line at the top, it may remove 3-4 "real"
|
|
|
|
lines (rows) depending on how long the original line was.
|
|
|
|
|
|
|
|
The `lines` parameter can be a string or an array of strings. The `line`
|
|
|
|
parameter must be a string.
|
|
|
|
|
|
|
|
- **setContent(text)** - set the content. note: when text is input, it will be
|
|
|
|
stripped of all non-SGR escape codes, tabs will be replaced with 8 spaces,
|
|
|
|
and tags will be replaced with SGR codes (if enabled).
|
|
|
|
- **getContent(text)** - return content, slightly different from `el.content`.
|
|
|
|
assume the above formatting.
|
|
|
|
- **insertLine(i, lines)** - insert a line into the box's content.
|
|
|
|
- **deleteLine(i)** - delete a line from the box's content.
|
|
|
|
- **getLine(i)** - get a line from the box's content.
|
|
|
|
- **getBaseLine(i)** - get a line from the box's content from the visible top.
|
|
|
|
- **setLine(i, line)** - set a line in the box's content.
|
|
|
|
- **setBaseLine(i, line)** - set a line in the box's content from the visible
|
|
|
|
top.
|
|
|
|
- **clearLine(i)** - clear a line from the box's content.
|
|
|
|
- **clearBaseLine(i)** - clear a line from the box's content from the visible
|
|
|
|
top.
|
|
|
|
- **insertTop(lines)** - insert a line at the top of the box.
|
|
|
|
- **insertBottom(lines)** - insert a line at the bottom of the box.
|
|
|
|
- **deleteTop()** - delete a line at the top of the box.
|
|
|
|
- **deleteBottom()** - delete a line at the bottom of the box.
|
|
|
|
- **unshiftLine(lines)** - unshift a line onto the top of the content.
|
|
|
|
- **shiftLine(i)** - shift a line off the top of the content.
|
|
|
|
- **pushLine(lines)** - push a line onto the bottom of the content.
|
|
|
|
- **popLine(i)** - pop a line off the top of the content.
|
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### Box (from Element)
|
|
|
|
|
|
|
|
A box element which draws a simple box containing `content` or other elements.
|
|
|
|
|
2013-07-12 09:59:58 +00:00
|
|
|
##### Options:
|
|
|
|
|
|
|
|
- inherits all from Element.
|
|
|
|
|
|
|
|
##### Properties:
|
|
|
|
|
|
|
|
- inherits all from Element.
|
|
|
|
|
|
|
|
##### Events:
|
|
|
|
|
|
|
|
- inherits all from Element.
|
|
|
|
|
|
|
|
##### Methods:
|
|
|
|
|
|
|
|
- inherits all from Element.
|
|
|
|
|
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### Text (from Element)
|
|
|
|
|
|
|
|
An element similar to Box, but geared towards rendering simple text elements.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Element.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **fill** - fill the entire line with chosen bg until parent bg ends, even if
|
2013-06-13 21:49:48 +00:00
|
|
|
there is not enough text to fill the entire width. **(deprecated)**
|
2013-06-13 09:14:14 +00:00
|
|
|
- **align** - text alignment: `left`, `center`, or `right`.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
Inherits all options, properties, events, and methods from Element.
|
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### Line (from Box)
|
|
|
|
|
2013-07-16 22:50:02 +00:00
|
|
|
A simple line which can be `line` or `bg` styled.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Box.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **orientation** - can be `vertical` or `horizontal`.
|
|
|
|
- **type, bg, fg, ch** - treated the same as a border object.
|
2013-07-17 03:28:56 +00:00
|
|
|
(attributes can be contained in `style`).
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
Inherits all options, properties, events, and methods from Box.
|
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### ScrollableBox (from Box)
|
|
|
|
|
2013-07-25 06:20:47 +00:00
|
|
|
**DEPRECATED** - Use box with the `scrollable` option instead.
|
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
A box with scrollable content.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Box.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **baseLimit** - a limit to the childBase. default is `Infinity`.
|
2013-06-25 23:17:21 +00:00
|
|
|
- **alwaysScroll** - a option which causes the ignoring of `childOffset`. this
|
|
|
|
in turn causes the childBase to change every time the element is scrolled.
|
|
|
|
- **scrollbar** - object enabling a scrollbar. allows `ch`, `fg`, and `bg`
|
|
|
|
properties.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Properties:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Box.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **childBase** - the offset of the top of the scroll content.
|
|
|
|
- **childOffset** - the offset of the chosen item (if there is one).
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Events:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Box.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **scroll** - received when the element is scrolled.
|
2013-06-25 22:31:13 +00:00
|
|
|
- **resetScroll** - reset scroll offset to zero.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Methods:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
- **scroll(offset)** - scroll the content by an offset.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### List (from ScrollableBox)
|
|
|
|
|
|
|
|
A scrollable list which can display selectable items.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
2013-07-16 05:52:13 +00:00
|
|
|
- **selectedFg, selectedBg** - foreground and background for selected item,
|
2013-07-17 03:28:56 +00:00
|
|
|
treated like fg and bg. (can be contained in style: e.g. `style.selected.fg`).
|
2013-06-11 17:52:18 +00:00
|
|
|
- **selectedBold, selectedUnderline** - character attributes for selected item,
|
2013-07-17 03:28:56 +00:00
|
|
|
treated like bold and underline. (can be contained in style: e.g.
|
|
|
|
`style.selected.bold`).
|
|
|
|
- **itemFg, itemBg** - foreground and background for unselected item,
|
|
|
|
treated like fg and bg. (can be contained in style: e.g. `style.item.fg`).
|
|
|
|
- **itemBold, itemUnderline** - character attributes for an unselected item,
|
|
|
|
treated like bold and underline. (can be contained in style: e.g.
|
|
|
|
`style.item.bold`).
|
2013-06-25 23:17:21 +00:00
|
|
|
- **mouse** - whether to automatically enable mouse support for this list
|
|
|
|
(allows clicking items).
|
2013-06-18 15:52:48 +00:00
|
|
|
- **keys** - use predefined keys for navigating the list.
|
|
|
|
- **vi** - use vi keys with the `keys` option.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **items** - an array of strings which become the list's items.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Properties:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Events:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **select** - received when an item is selected.
|
2013-06-18 15:52:48 +00:00
|
|
|
- **cancel** - list was canceled (when `esc` is pressed with the `keys` option).
|
|
|
|
- **action** - either a select or a cancel event was received.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Methods:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **add(text)** - add an item based on a string.
|
|
|
|
- **select(index)** - select an index of an item.
|
|
|
|
- **move(offset)** - select item based on current offset.
|
|
|
|
- **up(amount)** - select item above selected.
|
|
|
|
- **down(amount)** - select item below selected.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### ScrollableText (from ScrollableBox)
|
|
|
|
|
2013-07-25 06:20:47 +00:00
|
|
|
**DEPRECATED** - Use box with the `scrollable` option instead.
|
|
|
|
|
2013-06-25 23:17:21 +00:00
|
|
|
A scrollable text box which can display and scroll text, as well as handle
|
|
|
|
pre-existing newlines and escape codes.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **mouse** - whether to enable automatic mouse support for this element.
|
2013-06-18 15:52:48 +00:00
|
|
|
- **keys** - use predefined keys for navigating the text.
|
|
|
|
- **vi** - use vi keys with the `keys` option.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Properties:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Events:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Methods:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
|
|
|
|
2013-07-17 05:53:34 +00:00
|
|
|
|
|
|
|
#### Form (from Box)
|
|
|
|
|
|
|
|
A form which can contain form elements.
|
|
|
|
|
|
|
|
##### Options:
|
|
|
|
|
|
|
|
- inherits all from Box.
|
|
|
|
- **keys** - allow default keys (tab, vi keys, enter).
|
|
|
|
- **vi** - allow vi keys.
|
|
|
|
|
|
|
|
##### Properties:
|
|
|
|
|
|
|
|
- inherits all from Box.
|
|
|
|
- **submission** - last submitted data.
|
|
|
|
|
|
|
|
##### Events:
|
|
|
|
|
|
|
|
- inherits all from Box.
|
|
|
|
- **submit** - form is submitted. receives a data object.
|
|
|
|
- **cancel** - form is discarded.
|
|
|
|
- **reset** - form is cleared.
|
|
|
|
|
|
|
|
##### Methods:
|
|
|
|
|
|
|
|
- inherits all from Box.
|
|
|
|
- **focusNext()** - focus next form element.
|
|
|
|
- **focusPrevious()** - focus previous form element.
|
|
|
|
- **submit()** - submit the form.
|
|
|
|
- **cancel()** - discard the form.
|
|
|
|
- **reset()** - clear the form.
|
|
|
|
|
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### Input (from Box)
|
|
|
|
|
|
|
|
A form input.
|
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### Textbox (from Input)
|
|
|
|
|
|
|
|
A box which allows text input.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Properties:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Events:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Input.
|
2013-07-17 05:53:34 +00:00
|
|
|
- **submit** - value is submitted (enter).
|
|
|
|
- **cancel** - value is discared (escape).
|
|
|
|
- **action** - either submit or cancel.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Methods:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Input.
|
2013-07-04 05:10:01 +00:00
|
|
|
- **readInput(callback)** - grab key events and start reading text from the
|
|
|
|
keyboard. takes a callback which receives the final value.
|
|
|
|
- **readEditor(callback)** - open text editor in `$EDITOR`, read the output from
|
|
|
|
the resulting file. takes a callback which receives the final value.
|
2013-07-04 06:50:50 +00:00
|
|
|
- **clearInput** - clear input.
|
2013-07-04 05:10:01 +00:00
|
|
|
|
|
|
|
|
|
|
|
#### Textarea (from Input/ScrollableText)
|
|
|
|
|
|
|
|
A box which allows multiline text input.
|
|
|
|
|
|
|
|
##### Options:
|
|
|
|
|
|
|
|
- inherits all from Input/ScrollableText.
|
|
|
|
|
|
|
|
##### Properties:
|
|
|
|
|
|
|
|
- inherits all from Input/ScrollableText.
|
|
|
|
|
|
|
|
##### Events:
|
|
|
|
|
|
|
|
- inherits all from Input/ScrollableText.
|
2013-07-17 05:53:34 +00:00
|
|
|
- **submit** - value is submitted (enter).
|
|
|
|
- **cancel** - value is discared (escape).
|
|
|
|
- **action** - either submit or cancel.
|
2013-07-04 05:10:01 +00:00
|
|
|
|
|
|
|
##### Methods:
|
|
|
|
|
|
|
|
- inherits all from Input/ScrollableText.
|
2013-07-03 23:46:06 +00:00
|
|
|
- **readInput(callback)** - grab key events and start reading text from the
|
2013-06-11 16:48:37 +00:00
|
|
|
keyboard. takes a callback which receives the final value.
|
2013-07-03 23:46:06 +00:00
|
|
|
- **readEditor(callback)** - open text editor in `$EDITOR`, read the output from
|
2013-06-25 23:17:21 +00:00
|
|
|
the resulting file. takes a callback which receives the final value.
|
2013-07-04 06:50:50 +00:00
|
|
|
- **clearInput** - clear input.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
|
|
|
#### Button (from Input)
|
|
|
|
|
|
|
|
A button which can be focused and allows key and mouse input.
|
|
|
|
|
|
|
|
##### Options:
|
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
|
|
|
|
##### Properties:
|
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
|
|
|
|
##### Events:
|
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
|
|
|
- **press** - received when the button is clicked/pressed.
|
|
|
|
|
|
|
|
##### Methods:
|
|
|
|
|
|
|
|
- inherits all from ScrollableBox.
|
|
|
|
- **add(text)** - add an item based on a string.
|
|
|
|
- **select(index)** - select an index of an item.
|
|
|
|
- **move(offset)** - select item based on current offset.
|
|
|
|
- **up(amount)** - select item above selected.
|
|
|
|
- **down(amount)** - select item below selected.
|
|
|
|
|
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
#### ProgressBar (from Input)
|
|
|
|
|
2013-07-17 05:53:34 +00:00
|
|
|
A progress bar allowing various styles. This can also be used as a form input.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Options:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Input.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **barFg, barBg** - (completed) bar foreground and background.
|
2013-07-17 03:28:56 +00:00
|
|
|
(can be contained in `style`: e.g. `style.bar.fg`).
|
2013-06-11 17:52:18 +00:00
|
|
|
- **ch** - the character to fill the bar with (default is space).
|
|
|
|
- **filled** - the amount filled (0 - 100).
|
2013-07-17 05:53:34 +00:00
|
|
|
- **value** - same as `filled`.
|
|
|
|
- **keys** - enable key support.
|
|
|
|
- **mouse** - enable mouse support.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Properties:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Events:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Input.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **reset** - bar was reset.
|
|
|
|
- **complete** - bar has completely filled.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-11 17:52:18 +00:00
|
|
|
##### Methods:
|
2013-06-11 16:48:37 +00:00
|
|
|
|
|
|
|
- inherits all from Input.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **progress(amount)** - progress the bar by a fill amount.
|
2013-07-17 05:53:34 +00:00
|
|
|
- **setProgress(amount)** - set progress to specific amount.
|
2013-06-11 17:52:18 +00:00
|
|
|
- **reset()** - reset the bar.
|
2013-06-11 16:48:37 +00:00
|
|
|
|
2013-06-16 09:21:57 +00:00
|
|
|
|
2013-07-12 12:48:55 +00:00
|
|
|
#### FileManager (from List)
|
|
|
|
|
|
|
|
A very simple file manager for selecting files.
|
|
|
|
|
|
|
|
##### Options:
|
|
|
|
|
|
|
|
- inherits all from List.
|
|
|
|
- **cwd** - current working directory.
|
|
|
|
|
|
|
|
##### Properties:
|
|
|
|
|
|
|
|
- inherits all from List.
|
|
|
|
- **cwd** - current working directory.
|
|
|
|
|
|
|
|
##### Events:
|
|
|
|
|
|
|
|
- inherits all from List.
|
|
|
|
- **cd** - directory was selected and navigated to.
|
|
|
|
- **file** - file was selected.
|
|
|
|
|
|
|
|
##### Methods:
|
|
|
|
|
|
|
|
- inherits all from List.
|
2013-07-12 15:22:06 +00:00
|
|
|
- **refresh([cwd], [callback])** - refresh the file list (perform a `readdir` on `cwd`
|
2013-07-12 12:48:55 +00:00
|
|
|
and update the list items).
|
2013-07-12 15:22:06 +00:00
|
|
|
- **pick([cwd], callback)** - pick a single file and return the path in the callback.
|
|
|
|
- **reset([cwd], [callback])** - reset back to original cwd.
|
2013-07-12 12:48:55 +00:00
|
|
|
|
|
|
|
|
2013-07-17 05:53:34 +00:00
|
|
|
#### Checkbox (from Input)
|
|
|
|
|
|
|
|
A checkbox which can be used in a form element.
|
|
|
|
|
|
|
|
##### Options:
|
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
- **checked** - whether the element is checked or not.
|
|
|
|
- **mouse** - enable mouse support.
|
|
|
|
|
|
|
|
##### Properties:
|
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
- **text** - the text next to the checkbox (do not use setContent, use
|
|
|
|
`check.text = ''`).
|
|
|
|
- **checked** - whether the element is checked or not.
|
|
|
|
- **value** - same as `checked`.
|
|
|
|
|
|
|
|
##### Events:
|
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
- **check** - received when element is checked.
|
|
|
|
- **uncheck** received when element is unchecked.
|
|
|
|
|
|
|
|
##### Methods:
|
|
|
|
|
|
|
|
- inherits all from Input.
|
|
|
|
- **check()** - check the element.
|
|
|
|
- **uncheck()** - uncheck the element.
|
|
|
|
- **toggle()** - toggle checked state.
|
|
|
|
|
|
|
|
|
|
|
|
#### RadioSet (from Box)
|
|
|
|
|
|
|
|
An element wrapping RadioButtons. RadioButtons within this element will be
|
|
|
|
mutually exclusive with each other.
|
|
|
|
|
|
|
|
##### Options:
|
|
|
|
|
|
|
|
- inherits all from Box.
|
|
|
|
|
|
|
|
##### Properties:
|
|
|
|
|
|
|
|
- inherits all from Box.
|
|
|
|
|
|
|
|
##### Events:
|
|
|
|
|
|
|
|
- inherits all from Box.
|
|
|
|
|
|
|
|
##### Methods:
|
|
|
|
|
|
|
|
- inherits all from Box.
|
|
|
|
|
|
|
|
|
|
|
|
#### RadioButton (from Checkbox)
|
|
|
|
|
|
|
|
A radio button which can be used in a form element.
|
|
|
|
|
|
|
|
##### Options:
|
|
|
|
|
|
|
|
- inherits all from Checkbox.
|
|
|
|
|
|
|
|
##### Properties:
|
|
|
|
|
|
|
|
- inherits all from Checkbox.
|
|
|
|
|
|
|
|
##### Events:
|
|
|
|
|
|
|
|
- inherits all from Checkbox.
|
|
|
|
|
|
|
|
##### Methods:
|
|
|
|
|
|
|
|
- inherits all from Checkbox.
|
|
|
|
|
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
### Positioning
|
|
|
|
|
|
|
|
Offsets may be a number, a percentage (e.g. `50%`), or a keyword (e.g.
|
|
|
|
`center`).
|
|
|
|
|
|
|
|
Dimensions may be a number, or a percentage (e.g. `50%`).
|
|
|
|
|
|
|
|
Positions are treated almost *exactly* the same as they are in CSS/CSSOM when
|
|
|
|
an element has the `position: absolute` CSS property.
|
|
|
|
|
|
|
|
When an element is created, it can be given coordinates in its constructor:
|
|
|
|
|
|
|
|
``` js
|
2013-07-04 04:15:09 +00:00
|
|
|
var box = blessed.box({
|
2013-06-11 16:48:37 +00:00
|
|
|
left: 'center',
|
|
|
|
top: 'center',
|
2013-06-14 00:02:02 +00:00
|
|
|
bg: 'yellow',
|
2013-06-11 16:48:37 +00:00
|
|
|
width: '50%',
|
|
|
|
height: '50%'
|
|
|
|
});
|
|
|
|
```
|
|
|
|
|
|
|
|
This tells blessed to create a box, perfectly centered **relative to its
|
|
|
|
parent**, 50% as wide and 50% as tall as its parent.
|
|
|
|
|
|
|
|
To access the calculated offsets, relative to the parent:
|
|
|
|
|
|
|
|
``` js
|
|
|
|
console.log(box.rleft);
|
|
|
|
console.log(box.rtop);
|
|
|
|
```
|
|
|
|
|
|
|
|
To access the calculated offsets, absolute (relative to the screen):
|
|
|
|
|
|
|
|
``` js
|
|
|
|
console.log(box.left);
|
|
|
|
console.log(box.top);
|
|
|
|
```
|
|
|
|
|
|
|
|
#### Overlapping offsets and dimensions greater than parents'
|
|
|
|
|
|
|
|
This still needs to be tested a bit, but it should work.
|
|
|
|
|
2013-07-03 23:46:06 +00:00
|
|
|
|
2013-06-24 11:16:02 +00:00
|
|
|
### Content
|
|
|
|
|
|
|
|
Every element can have text content via `setContent`. If `tags: true` was
|
|
|
|
passed to the element's constructor, the content can contain tags. For example:
|
|
|
|
|
|
|
|
```
|
|
|
|
box.setContent('hello {red-fg}{green-bg}{bold}world{/bold}{/green-bg}{/red-fg}');
|
|
|
|
```
|
|
|
|
|
|
|
|
To make this more concise `{/}` cancels all character attributes.
|
|
|
|
|
|
|
|
```
|
|
|
|
box.setContent('hello {red-fg}{green-bg}{bold}world{/}');
|
|
|
|
```
|
|
|
|
|
|
|
|
Newlines and alignment are also possible in content.
|
|
|
|
|
2013-07-03 23:46:06 +00:00
|
|
|
``` js
|
2013-06-24 11:16:02 +00:00
|
|
|
box.setContent('hello\n'
|
|
|
|
+ '{right}world{/right}\n'
|
|
|
|
+ '{center}foo{/center}');
|
|
|
|
```
|
|
|
|
|
|
|
|
This will produce a box that looks like:
|
|
|
|
|
|
|
|
```
|
|
|
|
| hello |
|
|
|
|
| world |
|
|
|
|
| foo |
|
|
|
|
```
|
|
|
|
|
|
|
|
Content can also handle SGR escape codes. This means if you got output from a
|
|
|
|
program, say `git log` for example, you can feed it directly to an element's
|
|
|
|
content and the colors will be parsed appropriately.
|
|
|
|
|
|
|
|
This means that while `{red-fg}foo{/red-fg}` produces `^[[31mfoo^[[39m`, you
|
|
|
|
could just feed `^[[31mfoo^[[39m` directly to the content.
|
|
|
|
|
2013-07-03 23:46:06 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
### Rendering
|
|
|
|
|
|
|
|
To actually render the screen buffer, you must call `render`.
|
|
|
|
|
|
|
|
``` js
|
|
|
|
box.setContent('Hello world.');
|
|
|
|
screen.render();
|
|
|
|
```
|
|
|
|
|
|
|
|
Elements are rendered with the lower elements in the children array being
|
|
|
|
painted first. In terms of the painter's algorithm, the lowest indicies in the
|
|
|
|
array are the furthest away, just like in the DOM.
|
|
|
|
|
2013-07-03 23:46:06 +00:00
|
|
|
|
2013-06-25 11:34:10 +00:00
|
|
|
### Optimization and CSR
|
|
|
|
|
2013-07-14 16:04:48 +00:00
|
|
|
**NOTE:** This is now automatically optimized for full-width scrollable elements.
|
|
|
|
|
|
|
|
**TODO:** Detect to see if there are any elements under/to the sides of
|
|
|
|
non-full-width elements.
|
|
|
|
|
2013-06-25 11:34:10 +00:00
|
|
|
You may notice a lot of terminal apps (e.g. mutt, irssi, vim, ncmpcpp) don't
|
|
|
|
have sidebars, and only have "elements" that take up the entire width of the
|
|
|
|
screen. The reason for this is speed (and general cleanliness). VT-like
|
|
|
|
terminals have something called a CSR (change_scroll_region) code, as well as
|
|
|
|
IL (insert_line), and DL (delete_code) codes. Using these three codes, it is
|
|
|
|
possible to create a very efficient rendering by avoiding redrawing the entire
|
|
|
|
screen when a line is inserted or removed. Since blessed is extremely dynamic,
|
|
|
|
it is hard to do this optimization automatically (blessed assumes you may
|
|
|
|
create any element of any width in any position). So, there is a solution:
|
|
|
|
|
|
|
|
``` js
|
2013-07-04 04:15:09 +00:00
|
|
|
var box = blessed.box(...);
|
2013-06-25 11:34:10 +00:00
|
|
|
box.setContent('line 1\nline 2');
|
|
|
|
box.insertBottom('line 3');
|
|
|
|
box.insertBottom('line 4');
|
|
|
|
box.insertTop('line 0');
|
2013-07-12 09:59:58 +00:00
|
|
|
box.insertLine(1, 'line 1.5');
|
2013-06-25 11:34:10 +00:00
|
|
|
```
|
|
|
|
|
|
|
|
If your element has the same width as the screen, the line insertion will be
|
|
|
|
optimized by using a combination CSR/IL/DL codes. These methods may be made
|
|
|
|
smarter in the future to detect whether any elements are being overlapped to
|
|
|
|
the sides.
|
|
|
|
|
|
|
|
Outputting:
|
|
|
|
|
|
|
|
```
|
|
|
|
| line 0 |
|
|
|
|
| line 1 |
|
2013-07-12 09:59:58 +00:00
|
|
|
| line 1.5 |
|
2013-06-25 11:34:10 +00:00
|
|
|
| line 2 |
|
|
|
|
| line 3 |
|
|
|
|
| line 4 |
|
|
|
|
```
|
|
|
|
|
2013-07-03 23:46:06 +00:00
|
|
|
|
2013-06-11 16:48:37 +00:00
|
|
|
### Testing
|
|
|
|
|
|
|
|
- For an interactive test, see `test/widget.js`.
|
|
|
|
- For a less interactive position testing, see `test/widget-pos.js`.
|
|
|
|
|
2013-07-03 23:46:06 +00:00
|
|
|
|
2013-07-15 22:45:08 +00:00
|
|
|
## Lower-Level Usage
|
|
|
|
|
|
|
|
This will actually parse the xterm terminfo and compile every
|
|
|
|
string capability to a javascript function:
|
|
|
|
|
|
|
|
``` js
|
2013-07-18 22:56:05 +00:00
|
|
|
var blessed = require('blessed')
|
|
|
|
, tput = blessed.tput('xterm-256color');
|
2013-07-15 22:45:08 +00:00
|
|
|
|
|
|
|
console.log(tput.setaf(4) + 'hello' + tput.sgr0());
|
|
|
|
```
|
|
|
|
|
|
|
|
To play around with it on the command line, it works just like tput:
|
|
|
|
|
|
|
|
``` bash
|
|
|
|
$ tput.js setaf 2
|
|
|
|
$ tput.js sgr0
|
|
|
|
$ echo "$(tput.js setaf 2)hello world$(tput.js sgr0)"
|
|
|
|
```
|
|
|
|
|
|
|
|
The main functionality is exposed in the main `blessed` module:
|
|
|
|
|
|
|
|
``` js
|
|
|
|
var blessed = require('blessed')
|
2013-07-18 22:56:05 +00:00
|
|
|
, program = blessed.program();
|
2013-07-15 22:45:08 +00:00
|
|
|
|
|
|
|
program.key('q', function(ch, key) {
|
|
|
|
program.clear();
|
|
|
|
program.disableMouse();
|
|
|
|
program.showCursor();
|
|
|
|
program.normalBuffer();
|
|
|
|
process.exit(0);
|
|
|
|
});
|
|
|
|
|
|
|
|
program.on('mouse', function(data) {
|
|
|
|
if (data.action === 'mousemove') {
|
|
|
|
program.move(data.x, data.y);
|
|
|
|
program.bg('red');
|
|
|
|
program.write('x');
|
|
|
|
program.bg('!red');
|
|
|
|
}
|
|
|
|
});
|
|
|
|
|
|
|
|
program.alternateBuffer();
|
|
|
|
program.enableMouse();
|
|
|
|
program.hideCursor();
|
|
|
|
program.clear();
|
|
|
|
|
|
|
|
program.move(1, 1);
|
|
|
|
program.bg('black');
|
|
|
|
program.write('Hello world', 'blue fg');
|
|
|
|
program.setx((program.cols / 2 | 0) - 4);
|
|
|
|
program.down(5);
|
|
|
|
program.write('Hi again!');
|
|
|
|
program.bg('!black');
|
|
|
|
program.feed();
|
|
|
|
```
|
|
|
|
|
|
|
|
|
2013-01-27 16:06:58 +00:00
|
|
|
## License
|
|
|
|
|
|
|
|
Copyright (c) 2013, Christopher Jeffrey. (MIT License)
|
|
|
|
|
|
|
|
See LICENSE for more info.
|