mirror of https://github.com/embarklabs/embark.git
fix: remove unneeded test_dapps/ directory in the monorepo root
This commit is contained in:
parent
20d3acb8b1
commit
5c2e30c26c
|
@ -1,23 +0,0 @@
|
|||
{
|
||||
"name": "packaged",
|
||||
"version": "1.0.0",
|
||||
"main": "index.js",
|
||||
"scripts": {
|
||||
"test": "echo \"Error: no test specified\" && exit 1"
|
||||
},
|
||||
"keywords": [],
|
||||
"author": "",
|
||||
"license": "ISC",
|
||||
"dependencies": {
|
||||
"embark": "file:../../../packages/embark/embark-4.0.0-beta.0.tgz",
|
||||
"embark-compiler": "file:../../../packages/embark-compiler/embark-compiler-4.0.0-beta.0.tgz",
|
||||
"embark-core": "file:../../../packages/embark-core/embark-core-4.0.0-beta.0.tgz",
|
||||
"embark-graph": "file:../../../packages/embark-graph/embark-graph-4.0.0-beta.0.tgz",
|
||||
"embark-ui": "file:../../../packages/embark-ui/embark-ui-4.0.0-beta.0.tgz",
|
||||
"embark-utils": "file:../../../packages/embark-utils/embark-utils-4.0.0-beta.0.tgz",
|
||||
"embark-vyper": "file:../../../packages/embark-vyper/embark-vyper-4.0.0-beta.0.tgz",
|
||||
"embarkjs": "file:../../../packages/embarkjs/embarkjs-4.0.0-beta.0.tgz"
|
||||
},
|
||||
"devDependencies": {},
|
||||
"description": ""
|
||||
}
|
|
@ -1,21 +0,0 @@
|
|||
pragma solidity ^0.4.17;
|
||||
|
||||
contract SimpleStorageTest2 {
|
||||
uint public storedData;
|
||||
|
||||
function() public payable { }
|
||||
|
||||
function SimpleStorage(uint initialValue) public {
|
||||
storedData = initialValue;
|
||||
}
|
||||
|
||||
function set(uint x) public {
|
||||
storedData = x;
|
||||
}
|
||||
|
||||
function get() public view returns (uint retVal) {
|
||||
return storedData;
|
||||
}
|
||||
|
||||
}
|
||||
|
|
@ -1,35 +0,0 @@
|
|||
pragma solidity ^0.4.17;
|
||||
|
||||
import "/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/.embark/contracts/embark-framework/embark/master/test_dapps/contracts_app/contracts/ownable.sol";
|
||||
import "/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/.embark/contracts/embark-framework/embark/master/test_dapps/contracts_app/contracts/contract_args.sol";
|
||||
|
||||
|
||||
contract SimpleStorageWithHttpImport is Ownable {
|
||||
uint public storedData;
|
||||
|
||||
function() public payable { }
|
||||
|
||||
constructor(uint initialValue) public {
|
||||
storedData = initialValue;
|
||||
}
|
||||
|
||||
function set(uint x) public {
|
||||
storedData = x;
|
||||
for(uint i = 0; i < 1000; i++) {
|
||||
storedData += i;
|
||||
}
|
||||
}
|
||||
|
||||
function set2(uint x) public onlyOwner {
|
||||
storedData = x;
|
||||
}
|
||||
|
||||
function get() public view returns (uint retVal) {
|
||||
return storedData;
|
||||
}
|
||||
|
||||
function getS() public pure returns (string d) {
|
||||
return "hello";
|
||||
}
|
||||
|
||||
}
|
|
@ -1,10 +0,0 @@
|
|||
pragma solidity ^0.4.18;
|
||||
contract AnotherStorage {
|
||||
address public simpleStorageAddress;
|
||||
address simpleStorageAddress2;
|
||||
|
||||
constructor(address addr) public {
|
||||
simpleStorageAddress = addr;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,16 +0,0 @@
|
|||
pragma solidity ^0.4.24;
|
||||
|
||||
contract ContractArgs {
|
||||
address public addr_1;
|
||||
address public addr_2;
|
||||
uint public value;
|
||||
|
||||
function() public payable { }
|
||||
|
||||
constructor(address[] _addresses, uint initialValue) public {
|
||||
addr_1 = _addresses[0];
|
||||
addr_2 = _addresses[1];
|
||||
value = initialValue;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,42 +0,0 @@
|
|||
pragma solidity ^0.4.24;
|
||||
|
||||
/**
|
||||
* @title Ownable
|
||||
* @dev The Ownable contract has an owner address, and provides basic authorization control
|
||||
* functions, this simplifies the implementation of "user permissions".
|
||||
*/
|
||||
contract Ownable {
|
||||
address public owner;
|
||||
|
||||
|
||||
/**
|
||||
* @dev The Ownable constructor sets the original `owner` of the contract to the sender
|
||||
* account.
|
||||
*/
|
||||
constructor() public {
|
||||
owner = msg.sender;
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* @dev Throws if called by any account other than the owner.
|
||||
*/
|
||||
modifier onlyOwner() {
|
||||
if (msg.sender != owner) {
|
||||
revert();
|
||||
}
|
||||
_;
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* @dev Allows the current owner to transfer control of the contract to a newOwner.
|
||||
* @param newOwner The address to transfer ownership to.
|
||||
*/
|
||||
function transferOwnership(address newOwner) public onlyOwner {
|
||||
if (newOwner != address(0)) {
|
||||
owner = newOwner;
|
||||
}
|
||||
}
|
||||
|
||||
}
|
|
@ -1,38 +0,0 @@
|
|||
pragma solidity ^0.4.25;
|
||||
|
||||
contract SimpleStorage {
|
||||
uint public storedData;
|
||||
address public registar;
|
||||
address owner;
|
||||
event EventOnSet2(bool passed, string message);
|
||||
|
||||
constructor(uint initialValue) public {
|
||||
storedData = initialValue;
|
||||
owner = msg.sender;
|
||||
}
|
||||
|
||||
function set(uint x) public {
|
||||
storedData = x;
|
||||
//require(msg.sender == owner);
|
||||
//require(msg.sender == 0x0);
|
||||
//storedData = x + 2;
|
||||
}
|
||||
|
||||
function set2(uint x) public {
|
||||
storedData = x;
|
||||
emit EventOnSet2(true, "hi");
|
||||
}
|
||||
|
||||
function get() public view returns (uint retVal) {
|
||||
return storedData;
|
||||
}
|
||||
|
||||
function getS() public pure returns (string d) {
|
||||
return "hello";
|
||||
}
|
||||
|
||||
function setRegistar(address x) public {
|
||||
registar = x;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,39 +0,0 @@
|
|||
pragma solidity ^0.4.25;
|
||||
|
||||
import "/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/.embark/app/contracts/ownable.sol";
|
||||
|
||||
contract SimpleStorageTest is Ownable {
|
||||
uint public storedData;
|
||||
address owner;
|
||||
|
||||
constructor(uint initialValue) public {
|
||||
storedData = initialValue;
|
||||
owner = msg.sender;
|
||||
}
|
||||
|
||||
function set(uint x) public onlyOwner {
|
||||
storedData = x;
|
||||
require(msg.sender != owner);
|
||||
storedData = x + 2;
|
||||
}
|
||||
|
||||
function set2(uint x) public onlyOwner {
|
||||
storedData = x;
|
||||
storedData = x + 2;
|
||||
}
|
||||
|
||||
function test(uint x) public {
|
||||
uint value = 1;
|
||||
assembly {
|
||||
let a := 1
|
||||
let b := 2
|
||||
revert(0, 0)
|
||||
}
|
||||
value = 2;
|
||||
}
|
||||
|
||||
function get() public view returns (uint retVal) {
|
||||
return storedData;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,16 +0,0 @@
|
|||
pragma solidity ^0.4.24;
|
||||
|
||||
contract SomeContract {
|
||||
address public addr_1;
|
||||
address public addr_2;
|
||||
uint public value;
|
||||
|
||||
function() public payable { }
|
||||
|
||||
constructor(address[] _addresses, uint initialValue) public {
|
||||
addr_1 = _addresses[0];
|
||||
addr_2 = _addresses[1];
|
||||
value = initialValue;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,27 +0,0 @@
|
|||
pragma solidity ^0.4.17;
|
||||
|
||||
library ZAMyLib {
|
||||
|
||||
function add(uint _a, uint _b) public pure returns (uint _c) {
|
||||
return _a + _b;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
contract Test {
|
||||
address public addr;
|
||||
address public ens;
|
||||
|
||||
function testAdd() public pure returns (uint _result) {
|
||||
return ZAMyLib.add(1, 2);
|
||||
}
|
||||
|
||||
function changeAddress(address _addr) public {
|
||||
addr = _addr;
|
||||
}
|
||||
|
||||
function changeENS(address _ens) public {
|
||||
ens = _ens;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,16 +0,0 @@
|
|||
pragma solidity ^0.4.17;
|
||||
|
||||
import "/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/.embark/app/contracts/zlib2.sol";
|
||||
|
||||
contract Test2 {
|
||||
address public addr;
|
||||
|
||||
function testAdd() public pure returns (uint _result) {
|
||||
return ZAMyLib2.add(1, 2);
|
||||
}
|
||||
|
||||
function changeAddress(address _addr) public {
|
||||
addr = _addr;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,9 +0,0 @@
|
|||
pragma solidity ^0.4.17;
|
||||
|
||||
library ZAMyLib2 {
|
||||
|
||||
function add(uint _a, uint _b) public pure returns (uint _c) {
|
||||
return _a + _b;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,78 +0,0 @@
|
|||
{
|
||||
"0x2288b08dab35c1b5a00cdcdacda800b2935cc01d5fa8f28ab31e68d8b60f6ac8": {
|
||||
"contracts": {
|
||||
"0x3043b04ad856d169c8f0b0509c0bc63192dc7edd92d6933c58708298a0e381be": {
|
||||
"name": "ENSRegistry",
|
||||
"address": "0x0774395587AAb8ccFC2E6964F333e7955bd27c3D"
|
||||
},
|
||||
"0x19e5f4df7b9e8e7528b7b03fedebef4be25ff0e0f6ccb1a2f53e20d031b23b7e": {
|
||||
"name": "Resolver",
|
||||
"address": "0xb9b263410fbC24EDc1F83e4b5b4Fe4af0412Ca6C"
|
||||
},
|
||||
"0xc5ec3e3e891e8f90132e3dca5833bc3d8a084cd7df05f2f5f00990414c7d3a86": {
|
||||
"name": "FIFSRegistrar",
|
||||
"address": "0x0462568b84451CE9F8a46826f13768359240F890"
|
||||
},
|
||||
"0x75704f0275c5f13dd702a407c9fe3c41dced3d87906beb9ef1f2bcc56e792db7": {
|
||||
"name": "AlreadyDeployedToken",
|
||||
"address": "0xece374063fe5cc7efbaca0a498477cada94e5ad6"
|
||||
},
|
||||
"0x18562ce34987cc469d7b4665456fef41487087286106e053cb4c8573a7b51bce": {
|
||||
"name": "SimpleStorageWithHttpImport",
|
||||
"address": "0x9755d892efCab7161b4144374a7793FC66D09Edb"
|
||||
},
|
||||
"0x5b1458851ee6947057448dc806e135f61a2e7e9f2a672e0127f3852cf4d18bf9": {
|
||||
"name": "SimpleStorageTest",
|
||||
"address": "0x3AF38647d845849259Af53005EF79E858A2Fd9C1"
|
||||
},
|
||||
"0xebf6bdbfcbbcfc4153e10195e078ae314cbcee7ea9f999bafabb75cebf7de5b6": {
|
||||
"name": "MyToken2",
|
||||
"address": "0x5A364263dc4a9a50486C1af36c41DE6587CF13e5"
|
||||
},
|
||||
"0x9fd5d7a1dfcfeeca8114e8796f3f80f4bedd1c2baf0cb8bd3d23ed27e8c4937e": {
|
||||
"name": "SimpleStorage",
|
||||
"address": "0x6C70B9DD83a3934D1a7308D9E56e52A386b68f51"
|
||||
},
|
||||
"0xd3a67cbdb116b16223fc2c7db7b216ee5259124157609ddc0d92d6ae37017fdb": {
|
||||
"name": "MyToken",
|
||||
"address": "0x29AF75f8Ba57AEb6a5CA58E273302DBa0074bA0E"
|
||||
},
|
||||
"0x9433d4ea0ccbb59dee8b86d356998ea95db82c777b684376370985d89747128d": {
|
||||
"name": "ZAMyLib",
|
||||
"address": "0x88007F6424abb34ce5Ceff9403227454f3385B5F"
|
||||
},
|
||||
"0x1e87da6d4df94ee1e64a4656034ed73323ee50c94907d79996cf5b620433dc22": {
|
||||
"name": "SimpleStorageTest2",
|
||||
"address": "0x16a9427FFB448FB27639b6a3Fe77866Ecb1FA4ED"
|
||||
},
|
||||
"0xb9ebc2cdee85ad7acfc01a006c4885e272030fd227425b5dca0bcc4888ca7a6f": {
|
||||
"name": "ZAMyLib2",
|
||||
"address": "0xED2eEe6F7ede991fA104fB235694F0fD3ad51F59"
|
||||
},
|
||||
"0xc46a9a85efd2efd118e2606eb5e7fe1d0241115f56ba14c5d33a479c83153ffd": {
|
||||
"name": "Test",
|
||||
"address": "0x49b71966a123d07E41df95CF8446032F1A4401da"
|
||||
},
|
||||
"0x1c84e96ba7f6dc02e17840ee4c4d5ebe78b9d96ac764aa5303011c274c77fb10": {
|
||||
"name": "PluginStorage",
|
||||
"address": "0x97084B04060db7996A241f5699C6D091927E78aA"
|
||||
},
|
||||
"0x2fe44ad30689f3eb9de7c50915e51d55b986abcc0e9e62374b5da4e04a615c11": {
|
||||
"name": "ContractArgs",
|
||||
"address": "0x3ea5e8894E7384DD32DFFCcccB8970108D2aC26E"
|
||||
},
|
||||
"0x49471cf26723c0c8710a86ee41398f8a29de47aa54f26540f656eef84e545542": {
|
||||
"name": "AnotherStorage",
|
||||
"address": "0x47E3d3Fd20519e809aF422476C95948ff72BA447"
|
||||
},
|
||||
"0x8885aba2715be8880e8f1c8ac66c1f6004e7c8d25cb1ec4c72120a0eaf1ad76e": {
|
||||
"name": "Test2",
|
||||
"address": "0xac9FFAf3FFCaA0d514f835d2ECEd5919B3729754"
|
||||
},
|
||||
"0x4d84db2a5e0779e933fa86c17fc9777f4e0b74ce7fe54549968015e254c88a60": {
|
||||
"name": "SimpleStorageTest",
|
||||
"address": "0x494748735312D87C54Ff36E9dc71f90fb800D7Df"
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
|
@ -1,17 +0,0 @@
|
|||
token
|
||||
token
|
||||
token
|
||||
versions
|
||||
help
|
||||
SimpleStorage.options
|
||||
await web3.eth.getAccounts()
|
||||
web3.eth.getAccounts()
|
||||
await web3.eth.getAccounts()
|
||||
web3.eth.getAccounts()
|
||||
Token.methods.
|
||||
Token.methods
|
||||
token
|
||||
Token
|
||||
2
|
||||
Token
|
||||
SimpleStorage
|
|
@ -1 +0,0 @@
|
|||
{"1550861848274":{"name":"ENSRegistry","functionName":"constructor","paramString":"","address":"0x0774395587AAb8ccFC2E6964F333e7955bd27c3D","status":true,"gasUsed":518184,"blockNumber":1,"transactionHash":"0xe4ced04bd8e714cd9804d61ddacbe8820ea614c83ee35217937ccffdda736a82"},"1550861848308":{"name":"Resolver","functionName":"constructor","paramString":"","address":"0xb9b263410fbC24EDc1F83e4b5b4Fe4af0412Ca6C","status":true,"gasUsed":934121,"blockNumber":2,"transactionHash":"0xa69c7dc77202533e8b9da1a69894774f523a1d39e5bd37c995a0ecdeb7d298df"},"1550861848336":{"name":"FIFSRegistrar","functionName":"constructor","paramString":"","address":"0x0462568b84451CE9F8a46826f13768359240F890","status":true,"gasUsed":279560,"blockNumber":3,"transactionHash":"0x3afa7f8bb7f605b96f55847a4a5a38a27ef336ea4f9407242f164f61e042e85a"},"1550861848510":{"name":"SimpleStorageWithHttpImport","functionName":"constructor","paramString":"","address":"0x9755d892efCab7161b4144374a7793FC66D09Edb","status":true,"gasUsed":288444,"blockNumber":14,"transactionHash":"0xdf21651c36d13ca7cbb2136fcbfed01475f98ae27fd67d96a6ccaca2ab3b4912"},"1550861848526":{"name":"SimpleStorage","functionName":"constructor","paramString":"","address":"0x6C70B9DD83a3934D1a7308D9E56e52A386b68f51","status":true,"gasUsed":306566,"blockNumber":15,"transactionHash":"0xa47cc2c96d69feab0b6a5e097c421247455e419bc237f6430fe762acc17e4729"},"1550861848535":{"name":"ZAMyLib","functionName":"constructor","paramString":"","address":"0x88007F6424abb34ce5Ceff9403227454f3385B5F","status":true,"gasUsed":97578,"blockNumber":16,"transactionHash":"0x19b7e2e707b1384bfea5fd4ea41e5d0cb26906a2f43d3f4b769fe954c86a3721"},"1550861848554":{"name":"ZAMyLib2","functionName":"constructor","paramString":"","address":"0xED2eEe6F7ede991fA104fB235694F0fD3ad51F59","status":true,"gasUsed":97578,"blockNumber":15,"transactionHash":"0xc3b175b1f1b4966e084a27a528c3c66bdc18246cdf759c2aa99fbf5189425026"},"1550861848572":{"name":"Test","functionName":"constructor","paramString":"","address":"0x49b71966a123d07E41df95CF8446032F1A4401da","status":true,"gasUsed":217035,"blockNumber":18,"transactionHash":"0xaf1c7890d73880c39042a4d76cb9d8e5ed292918ac8138cffe9f1b4e0e10ccdc"},"1550861848591":{"name":"PluginStorage","functionName":"constructor","paramString":"","address":"0x97084B04060db7996A241f5699C6D091927E78aA","status":true,"gasUsed":130874,"blockNumber":19,"transactionHash":"0x82f0697ae6ae7437f4804f13078867c8f91854279843af1bc035ddd8b315c603"},"1550861848607":{"name":"Test2","functionName":"constructor","paramString":"","address":"0xac9FFAf3FFCaA0d514f835d2ECEd5919B3729754","status":true,"gasUsed":194353,"blockNumber":22,"transactionHash":"0xf0fab7a195a1ad4c7804950a8d958b9d229c889cc2859be2347b799284628f5c"},"1550872682006":{"type":"contract-log","address":"0x6c70b9dd83a3934d1a7308d9e56e52a386b68f51","data":"0x60fe47b1000000000000000000000000000000000000000000000000000000000000000a","transactionHash":"0x17497132504491d2cde22f21edce7fb477f3e3b50d5fd2e751ed3edc7ee9a695","blockNumber":33,"gasUsed":26647,"status":"0x1","name":"SimpleStorage","functionName":"set","paramString":"10"},"1551194123539":{"name":"SimpleStorageTest","functionName":"constructor","paramString":"","address":"0x494748735312D87C54Ff36E9dc71f90fb800D7Df","status":true,"gasUsed":275308,"blockNumber":40,"transactionHash":"0x411cfbe51d8b2e41fa0d48d8ddb4f0a96a784488e4685fb3be2341b4ac39670a"}}
|
|
@ -1,16 +0,0 @@
|
|||
pragma solidity ^0.4.24;
|
||||
|
||||
contract ContractArgs {
|
||||
address public addr_1;
|
||||
address public addr_2;
|
||||
uint public value;
|
||||
|
||||
function() public payable { }
|
||||
|
||||
constructor(address[] _addresses, uint initialValue) public {
|
||||
addr_1 = _addresses[0];
|
||||
addr_2 = _addresses[1];
|
||||
value = initialValue;
|
||||
}
|
||||
|
||||
}
|
|
@ -1,42 +0,0 @@
|
|||
pragma solidity ^0.4.17;
|
||||
|
||||
/**
|
||||
* @title Ownable
|
||||
* @dev The Ownable contract has an owner address, and provides basic authorization control
|
||||
* functions, this simplifies the implementation of "user permissions".
|
||||
*/
|
||||
contract Ownable {
|
||||
address public owner;
|
||||
|
||||
|
||||
/**
|
||||
* @dev The Ownable constructor sets the original `owner` of the contract to the sender
|
||||
* account.
|
||||
*/
|
||||
constructor() public {
|
||||
owner = msg.sender;
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* @dev Throws if called by any account other than the owner.
|
||||
*/
|
||||
modifier onlyOwner() {
|
||||
if (msg.sender != owner) {
|
||||
revert();
|
||||
}
|
||||
_;
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* @dev Allows the current owner to transfer control of the contract to a newOwner.
|
||||
* @param newOwner The address to transfer ownership to.
|
||||
*/
|
||||
function transferOwnership(address newOwner) public onlyOwner {
|
||||
if (newOwner != address(0)) {
|
||||
owner = newOwner;
|
||||
}
|
||||
}
|
||||
|
||||
}
|
|
@ -1,53 +0,0 @@
|
|||
pragma solidity ^0.4.23;
|
||||
|
||||
// Abstract contract for the full ERC 20 Token standard
|
||||
// https://github.com/ethereum/EIPs/issues/20
|
||||
|
||||
interface ERC20Token {
|
||||
|
||||
/**
|
||||
* @notice send `_value` token to `_to` from `msg.sender`
|
||||
* @param _to The address of the recipient
|
||||
* @param _value The amount of token to be transferred
|
||||
* @return Whether the transfer was successful or not
|
||||
*/
|
||||
function transfer(address _to, uint256 _value) external returns (bool success);
|
||||
|
||||
/**
|
||||
* @notice `msg.sender` approves `_spender` to spend `_value` tokens
|
||||
* @param _spender The address of the account able to transfer the tokens
|
||||
* @param _value The amount of tokens to be approved for transfer
|
||||
* @return Whether the approval was successful or not
|
||||
*/
|
||||
function approve(address _spender, uint256 _value) external returns (bool success);
|
||||
|
||||
/**
|
||||
* @notice send `_value` token to `_to` from `_from` on the condition it is approved by `_from`
|
||||
* @param _from The address of the sender
|
||||
* @param _to The address of the recipient
|
||||
* @param _value The amount of token to be transferred
|
||||
* @return Whether the transfer was successful or not
|
||||
*/
|
||||
function transferFrom(address _from, address _to, uint256 _value) external returns (bool success);
|
||||
|
||||
/**
|
||||
* @param _owner The address from which the balance will be retrieved
|
||||
* @return The balance
|
||||
*/
|
||||
function balanceOf(address _owner) external view returns (uint256 balance);
|
||||
|
||||
/**
|
||||
* @param _owner The address of the account owning tokens
|
||||
* @param _spender The address of the account able to transfer the tokens
|
||||
* @return Amount of remaining tokens allowed to spent
|
||||
*/
|
||||
function allowance(address _owner, address _spender) external view returns (uint256 remaining);
|
||||
|
||||
/**
|
||||
* @notice return total supply of tokens
|
||||
*/
|
||||
function totalSupply() external view returns (uint256 supply);
|
||||
|
||||
event Transfer(address indexed _from, address indexed _to, uint256 _value);
|
||||
event Approval(address indexed _owner, address indexed _spender, uint256 _value);
|
||||
}
|
|
@ -1,128 +0,0 @@
|
|||
pragma solidity ^0.4.24;
|
||||
|
||||
import "/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/.embark/contracts/status-im/contracts/151-embark31/contracts/token/ERC20Token.sol";
|
||||
|
||||
contract StandardToken is ERC20Token {
|
||||
|
||||
uint256 private supply;
|
||||
mapping (address => uint256) balances;
|
||||
mapping (address => mapping (address => uint256)) allowed;
|
||||
|
||||
constructor() internal { }
|
||||
|
||||
function transfer(
|
||||
address _to,
|
||||
uint256 _value
|
||||
)
|
||||
external
|
||||
returns (bool success)
|
||||
{
|
||||
return transfer(msg.sender, _to, _value);
|
||||
}
|
||||
|
||||
function approve(
|
||||
address _to,
|
||||
uint256 _value
|
||||
)
|
||||
external
|
||||
returns (bool success)
|
||||
{
|
||||
return approve(msg.sender, _to, _value);
|
||||
}
|
||||
|
||||
function transferFrom(
|
||||
address _from,
|
||||
address _to,
|
||||
uint256 _value
|
||||
)
|
||||
external
|
||||
returns (bool success)
|
||||
{
|
||||
if (balances[_from] >= _value &&
|
||||
allowed[_from][msg.sender] >= _value &&
|
||||
_value > 0) {
|
||||
allowed[_from][msg.sender] -= _value;
|
||||
return transfer(_from, _to, _value);
|
||||
} else {
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
function allowance(address _owner, address _spender)
|
||||
external
|
||||
view
|
||||
returns (uint256 remaining)
|
||||
{
|
||||
return allowed[_owner][_spender];
|
||||
}
|
||||
|
||||
function balanceOf(address _owner)
|
||||
external
|
||||
view
|
||||
returns (uint256 balance)
|
||||
{
|
||||
return balances[_owner];
|
||||
}
|
||||
|
||||
function totalSupply()
|
||||
external
|
||||
view
|
||||
returns(uint256 currentTotalSupply)
|
||||
{
|
||||
return supply;
|
||||
}
|
||||
|
||||
/**
|
||||
* @dev Aprove the passed address to spend the specified amount of tokens on behalf of msg.sender.
|
||||
* @param _from The address that is approving the spend
|
||||
* @param _spender The address which will spend the funds.
|
||||
* @param _value The amount of tokens to be spent.
|
||||
*/
|
||||
function approve(address _from, address _spender, uint256 _value) internal returns (bool) {
|
||||
|
||||
// To change the approve amount you first have to reduce the addresses`
|
||||
// allowance to zero by calling `approve(_spender, 0)` if it is not
|
||||
// already 0 to mitigate the race condition described here:
|
||||
// https://github.com/ethereum/EIPs/issues/20#issuecomment-263524729
|
||||
require((_value == 0) || (allowed[_from][_spender] == 0), "Bad usage");
|
||||
|
||||
allowed[_from][_spender] = _value;
|
||||
emit Approval(_from, _spender, _value);
|
||||
return true;
|
||||
}
|
||||
|
||||
function mint(
|
||||
address _to,
|
||||
uint256 _amount
|
||||
)
|
||||
internal
|
||||
{
|
||||
balances[_to] += _amount;
|
||||
supply += _amount;
|
||||
emit Transfer(0x0, _to, _amount);
|
||||
}
|
||||
|
||||
function transfer(
|
||||
address _from,
|
||||
address _to,
|
||||
uint256 _value
|
||||
)
|
||||
internal
|
||||
returns (bool success)
|
||||
{
|
||||
if (balances[_from] >= _value && _value > 0) {
|
||||
balances[_from] -= _value;
|
||||
if(_to == address(0)) {
|
||||
supply -= _value;
|
||||
} else {
|
||||
balances[_to] += _value;
|
||||
}
|
||||
emit Transfer(_from, _to, _value);
|
||||
return true;
|
||||
} else {
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
}
|
|
@ -1,69 +0,0 @@
|
|||
// https://github.com/nexusdev/erc20/blob/master/contracts/base.sol
|
||||
|
||||
pragma solidity ^0.4.17;
|
||||
contract Token {
|
||||
|
||||
event Transfer(address indexed from, address indexed to, uint value);
|
||||
event Approval( address indexed owner, address indexed spender, uint value);
|
||||
|
||||
mapping( address => uint ) _balances;
|
||||
mapping( address => mapping( address => uint ) ) _approvals;
|
||||
uint public _supply;
|
||||
//uint public _supply2;
|
||||
constructor( uint initial_balance ) public {
|
||||
_balances[msg.sender] = initial_balance;
|
||||
_supply = initial_balance;
|
||||
}
|
||||
function totalSupply() public constant returns (uint supply) {
|
||||
return _supply;
|
||||
}
|
||||
function balanceOf( address who ) public constant returns (uint value) {
|
||||
return _balances[who];
|
||||
}
|
||||
function transfer( address to, uint value) public returns (bool ok) {
|
||||
if( _balances[msg.sender] < value ) {
|
||||
revert();
|
||||
}
|
||||
if( !safeToAdd(_balances[to], value) ) {
|
||||
revert();
|
||||
}
|
||||
_balances[msg.sender] -= value;
|
||||
_balances[to] += value;
|
||||
emit Transfer( msg.sender, to, value );
|
||||
return true;
|
||||
}
|
||||
function transferFrom( address from, address to, uint value) public returns (bool ok) {
|
||||
// if you don't have enough balance, throw
|
||||
if( _balances[from] < value ) {
|
||||
revert();
|
||||
}
|
||||
// if you don't have approval, throw
|
||||
if( _approvals[from][msg.sender] < value ) {
|
||||
revert();
|
||||
}
|
||||
if( !safeToAdd(_balances[to], value) ) {
|
||||
revert();
|
||||
}
|
||||
// transfer and return true
|
||||
_approvals[from][msg.sender] -= value;
|
||||
_balances[from] -= value;
|
||||
_balances[to] += value;
|
||||
emit Transfer( from, to, value );
|
||||
return true;
|
||||
}
|
||||
function approve(address spender, uint value) public returns (bool ok) {
|
||||
// TODO: should increase instead
|
||||
_approvals[msg.sender][spender] = value;
|
||||
emit Approval( msg.sender, spender, value );
|
||||
return true;
|
||||
}
|
||||
function allowance(address owner, address spender) public constant returns (uint _allowance) {
|
||||
return _approvals[owner][spender];
|
||||
}
|
||||
function safeToAdd(uint a, uint b) internal pure returns (bool) {
|
||||
return (a + b >= a);
|
||||
}
|
||||
function isAvailable() public pure returns (bool) {
|
||||
return false;
|
||||
}
|
||||
}
|
Binary file not shown.
Binary file not shown.
|
@ -1 +0,0 @@
|
|||
MANIFEST-000026
|
|
@ -1 +0,0 @@
|
|||
MANIFEST-000022
|
|
@ -1,121 +0,0 @@
|
|||
=============== Feb 22, 2019 (EST) ===============
|
||||
13:57:15.617036 log@legend F·NumFile S·FileSize N·Entry C·BadEntry B·BadBlock Ke·KeyError D·DroppedEntry L·Level Q·SeqNum T·TimeElapsed
|
||||
13:57:15.617906 db@open opening
|
||||
13:57:15.622591 version@stat F·[] S·0B[] Sc·[]
|
||||
13:57:15.622996 db@janitor F·2 G·0
|
||||
13:57:15.623025 db@open done T·5.102904ms
|
||||
14:00:52.710375 db@close closing
|
||||
14:00:52.711033 db@close done T·654.209µs
|
||||
=============== Feb 22, 2019 (EST) ===============
|
||||
14:33:53.785874 log@legend F·NumFile S·FileSize N·Entry C·BadEntry B·BadBlock Ke·KeyError D·DroppedEntry L·Level Q·SeqNum T·TimeElapsed
|
||||
14:33:53.787217 version@stat F·[] S·0B[] Sc·[]
|
||||
14:33:53.787250 db@open opening
|
||||
14:33:53.787334 journal@recovery F·1
|
||||
14:33:53.791411 journal@recovery recovering @1
|
||||
14:33:53.795243 memdb@flush created L0@2 N·482 S·59KiB "\x02ƚ..\xc8Ɨ,v390":"\xff\xc5n..\tO\a,v407"
|
||||
14:33:53.805480 version@stat F·[1] S·59KiB[59KiB] Sc·[0.25]
|
||||
14:33:53.807948 db@janitor F·3 G·0
|
||||
14:33:53.807992 db@open done T·20.727465ms
|
||||
14:41:03.713161 db@close closing
|
||||
14:41:03.714463 db@close done T·1.301616ms
|
||||
=============== Feb 22, 2019 (EST) ===============
|
||||
14:41:10.594349 log@legend F·NumFile S·FileSize N·Entry C·BadEntry B·BadBlock Ke·KeyError D·DroppedEntry L·Level Q·SeqNum T·TimeElapsed
|
||||
14:41:10.595460 version@stat F·[1] S·59KiB[59KiB] Sc·[0.25]
|
||||
14:41:10.595509 db@open opening
|
||||
14:41:10.595640 journal@recovery F·1
|
||||
14:41:10.599862 journal@recovery recovering @3
|
||||
14:41:10.600842 memdb@flush created L0@5 N·3 S·417B "Dat..ion,v485":"eth..\x0fj\xc8,v484"
|
||||
14:41:10.610907 version@stat F·[2] S·59KiB[59KiB] Sc·[0.50]
|
||||
14:41:10.612895 db@janitor F·4 G·0
|
||||
14:41:10.612950 db@open done T·17.420889ms
|
||||
=============== Feb 26, 2019 (EST) ===============
|
||||
07:41:12.635767 log@legend F·NumFile S·FileSize N·Entry C·BadEntry B·BadBlock Ke·KeyError D·DroppedEntry L·Level Q·SeqNum T·TimeElapsed
|
||||
07:41:12.637809 version@stat F·[2] S·59KiB[59KiB] Sc·[0.50]
|
||||
07:41:12.637884 db@open opening
|
||||
07:41:12.638155 journal@recovery F·1
|
||||
07:41:12.643516 journal@recovery recovering @6
|
||||
07:41:12.644936 memdb@flush created L0@8 N·13 S·1KiB "Dat..ion,v489":"r\x00\x00..f\x88\xc3,v495"
|
||||
07:41:12.658050 version@stat F·[3] S·60KiB[60KiB] Sc·[0.75]
|
||||
07:41:12.661225 db@janitor F·5 G·0
|
||||
07:41:12.661291 db@open done T·23.387448ms
|
||||
07:41:12.943295 table@compaction L0·3 -> L1·0 S·60KiB Q·514
|
||||
07:41:12.945552 table@build created L1@11 N·390 S·56KiB "\x02ƚ..\xc8Ɨ,v390":"\xff\xc5n..\tO\a,v407"
|
||||
07:41:12.945611 version@stat F·[0 1] S·56KiB[0B 56KiB] Sc·[0.00 0.00]
|
||||
07:41:12.945879 table@compaction committed F-2 S-3KiB Ke·0 D·108 T·2.517049ms
|
||||
07:41:12.946032 table@remove removed @8
|
||||
07:41:12.946137 table@remove removed @5
|
||||
07:41:12.946805 table@remove removed @2
|
||||
07:41:17.017947 db@close closing
|
||||
07:41:17.018152 db@close done T·203.855µs
|
||||
=============== Feb 26, 2019 (EST) ===============
|
||||
07:41:27.982217 log@legend F·NumFile S·FileSize N·Entry C·BadEntry B·BadBlock Ke·KeyError D·DroppedEntry L·Level Q·SeqNum T·TimeElapsed
|
||||
07:41:27.983713 version@stat F·[0 1] S·56KiB[0B 56KiB] Sc·[0.00 0.00]
|
||||
07:41:27.983772 db@open opening
|
||||
07:41:27.983919 journal@recovery F·1
|
||||
07:41:27.989446 journal@recovery recovering @9
|
||||
07:41:27.990547 memdb@flush created L0@12 N·40 S·3KiB "\x18s\xa6..\xa9q\xac,v530":"\xfbȴ..\x82\xcc&,v532"
|
||||
07:41:28.004389 version@stat F·[1 1] S·60KiB[3KiB 56KiB] Sc·[0.25 0.00]
|
||||
07:41:28.007540 db@janitor F·4 G·0
|
||||
07:41:28.007598 db@open done T·23.80168ms
|
||||
07:41:41.052151 table@compaction L0·1 -> L1·1 S·60KiB Q·545
|
||||
07:41:41.054115 table@build created L1@15 N·417 S·60KiB "\x02ƚ..\xc8Ɨ,v390":"\xff\xc5n..\tO\a,v407"
|
||||
07:41:41.054177 version@stat F·[0 1] S·60KiB[0B 60KiB] Sc·[0.00 0.00]
|
||||
07:41:41.054431 table@compaction committed F-1 S-586B Ke·0 D·13 T·2.111022ms
|
||||
07:41:41.054577 table@remove removed @12
|
||||
07:41:41.054692 table@remove removed @11
|
||||
09:56:14.800008 db@close closing
|
||||
09:56:14.800827 db@close done T·832.454µs
|
||||
=============== Feb 26, 2019 (EST) ===============
|
||||
09:56:21.683346 log@legend F·NumFile S·FileSize N·Entry C·BadEntry B·BadBlock Ke·KeyError D·DroppedEntry L·Level Q·SeqNum T·TimeElapsed
|
||||
09:56:21.684826 version@stat F·[0 1] S·60KiB[0B 60KiB] Sc·[0.00 0.00]
|
||||
09:56:21.684874 db@open opening
|
||||
09:56:21.684987 journal@recovery F·1
|
||||
09:56:21.688869 journal@recovery recovering @13
|
||||
09:56:21.689974 memdb@flush created L0@16 N·21 S·2KiB "\b\xef\x8e..45F,v559":"\xf3\xa0\xc3..\xe8-\xdb,v563"
|
||||
09:56:21.698639 version@stat F·[1 1] S·62KiB[2KiB 60KiB] Sc·[0.25 0.00]
|
||||
09:56:21.700855 db@janitor F·4 G·0
|
||||
09:56:21.700892 db@open done T·16.006483ms
|
||||
09:56:21.925612 table@compaction L0·1 -> L1·1 S·62KiB Q·567
|
||||
09:56:21.927638 table@build created L1@19 N·430 S·61KiB "\x02ƚ..\xc8Ɨ,v390":"\xff\xc5n..\tO\a,v407"
|
||||
09:56:21.927693 version@stat F·[0 1] S·61KiB[0B 61KiB] Sc·[0.00 0.00]
|
||||
09:56:21.928055 table@compaction committed F-1 S-509B Ke·0 D·8 T·2.393151ms
|
||||
09:56:21.928226 table@remove removed @16
|
||||
09:56:21.928349 table@remove removed @15
|
||||
09:58:35.950903 db@close closing
|
||||
09:58:35.951380 db@close done T·475.162µs
|
||||
=============== Feb 26, 2019 (EST) ===============
|
||||
09:58:40.646909 log@legend F·NumFile S·FileSize N·Entry C·BadEntry B·BadBlock Ke·KeyError D·DroppedEntry L·Level Q·SeqNum T·TimeElapsed
|
||||
09:58:40.648000 version@stat F·[0 1] S·61KiB[0B 61KiB] Sc·[0.00 0.00]
|
||||
09:58:40.648034 db@open opening
|
||||
09:58:40.648117 journal@recovery F·1
|
||||
09:58:40.651818 journal@recovery recovering @17
|
||||
09:58:40.652721 memdb@flush created L0@20 N·37 S·3KiB "\x048B..\x1f#T,v596":"\xf8w\n..\x16[\xa1,v590"
|
||||
09:58:40.660783 version@stat F·[1 1] S·65KiB[3KiB 61KiB] Sc·[0.25 0.00]
|
||||
09:58:40.662488 db@janitor F·4 G·0
|
||||
09:58:40.662525 db@open done T·14.476577ms
|
||||
09:58:40.917371 table@compaction L0·1 -> L1·1 S·65KiB Q·605
|
||||
09:58:40.920293 table@build created L1@23 N·456 S·64KiB "\x02ƚ..\xc8Ɨ,v390":"\xff\xc5n..\tO\a,v407"
|
||||
09:58:40.920349 version@stat F·[0 1] S·64KiB[0B 64KiB] Sc·[0.00 0.00]
|
||||
09:58:40.920637 table@compaction committed F-1 S-1KiB Ke·0 D·11 T·3.209283ms
|
||||
09:58:40.920801 table@remove removed @20
|
||||
09:58:40.920931 table@remove removed @19
|
||||
09:58:51.082494 db@close closing
|
||||
09:58:51.082684 db@close done T·189.315µs
|
||||
=============== Feb 26, 2019 (EST) ===============
|
||||
10:15:14.064706 log@legend F·NumFile S·FileSize N·Entry C·BadEntry B·BadBlock Ke·KeyError D·DroppedEntry L·Level Q·SeqNum T·TimeElapsed
|
||||
10:15:14.066297 version@stat F·[0 1] S·64KiB[0B 64KiB] Sc·[0.00 0.00]
|
||||
10:15:14.066348 db@open opening
|
||||
10:15:14.066461 journal@recovery F·1
|
||||
10:15:14.071055 journal@recovery recovering @21
|
||||
10:15:14.073080 memdb@flush created L0@24 N·21 S·1KiB "\x01\xca4..ӧ\xe5,v619":"\xef\xe0\xe5..\\\xb6a,v620"
|
||||
10:15:14.081751 version@stat F·[1 1] S·66KiB[1KiB 64KiB] Sc·[0.25 0.00]
|
||||
10:15:14.085424 db@janitor F·4 G·0
|
||||
10:15:14.085480 db@open done T·19.115455ms
|
||||
10:15:22.738764 table@compaction L0·1 -> L1·1 S·66KiB Q·627
|
||||
10:15:22.742747 table@build created L1@27 N·469 S·65KiB "\x01\xca4..ӧ\xe5,v619":"\xff\xc5n..\tO\a,v407"
|
||||
10:15:22.743076 version@stat F·[0 1] S·65KiB[0B 65KiB] Sc·[0.00 0.00]
|
||||
10:15:22.743667 table@compaction committed F-1 S-723B Ke·0 D·8 T·4.365914ms
|
||||
10:15:22.743814 table@remove removed @24
|
||||
10:15:22.743931 table@remove removed @23
|
||||
12:15:10.997161 db@close closing
|
||||
12:15:10.997852 db@close done T·691.018µs
|
Binary file not shown.
|
@ -1 +0,0 @@
|
|||
d77a627d8fae10c775082fe473579bf914f2ac29df9d3ff763ce0bf64088ad8f
|
|
@ -1 +0,0 @@
|
|||
{"address":"60697d2be95c9400f37fa636fe215a42ad863af0","crypto":{"cipher":"aes-128-ctr","ciphertext":"4c9d873f70473541e814e6451c7d2cc6ab8cdaea0998b746e577730c851501bd","cipherparams":{"iv":"7b6838281363271606ebdca28981a7a3"},"kdf":"scrypt","kdfparams":{"dklen":32,"n":262144,"p":1,"r":8,"salt":"8352da93b44c34e2232a622e88f60b1e32a0053c4f2725ac29571fdb1e059603"},"mac":"ae102c88af3bc633497463ed118a75717478ddf13e7e67bf6d9c15873af25140"},"id":"164314d3-20af-4859-a933-fadcbbd3ef0f","version":3}
|
|
@ -1 +0,0 @@
|
|||
{"Embark/contracts/ERC20Token":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/ERC20Token.js","Embark/contracts/PluginStorage":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/PluginStorage.js","Embark/contracts/StandardToken":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/StandardToken.js","Embark/contracts/ZAMyLib2":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/ZAMyLib2.js","Embark/contracts/ContractArgs":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/ContractArgs.js","Embark/contracts/AnotherStorage":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/AnotherStorage.js","Embark/contracts/SomeContract":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/SomeContract.js","Embark/contracts/ZAMyLib":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/ZAMyLib.js","Embark/contracts/SimpleStorageWithHttpImport":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/SimpleStorageWithHttpImport.js","Embark/contracts/Test2":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/Test2.js","Embark/contracts/SimpleStorageTest":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/SimpleStorageTest.js","Embark/contracts/Ownable":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/Ownable.js","Embark/contracts/SimpleStorage":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/SimpleStorage.js","Embark/contracts/Test":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/Test.js","Embark/contracts/AlreadyDeployedToken":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/AlreadyDeployedToken.js","Embark/contracts/Token":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/Token.js","Embark/contracts/MyToken2":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/MyToken2.js","Embark/contracts/MyToken":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts/MyToken.js","Embark/EmbarkJS":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/embarkjs.js","Embark/contracts":"/Users/iurimatias/Projects/Status/embark/test_dapps/test_app/embarkArtifacts/contracts"}
|
File diff suppressed because one or more lines are too long
|
@ -1 +0,0 @@
|
|||
{"typescript":false}
|
|
@ -1,23 +0,0 @@
|
|||
pragma solidity ^0.4.17;
|
||||
|
||||
import "../another_folder/another_test.sol";
|
||||
import "zeppelin-solidity/contracts/ownership/Ownable.sol";
|
||||
|
||||
contract SimpleStorageTest is Ownable {
|
||||
uint public storedData;
|
||||
|
||||
function() public payable { }
|
||||
|
||||
function SimpleStorage(uint initialValue) public {
|
||||
storedData = initialValue;
|
||||
}
|
||||
|
||||
function set(uint x) public {
|
||||
storedData = x;
|
||||
}
|
||||
|
||||
function get() public view returns (uint retVal) {
|
||||
return storedData;
|
||||
}
|
||||
|
||||
}
|
File diff suppressed because one or more lines are too long
File diff suppressed because it is too large
Load Diff
|
@ -1,18 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
const factory = require('./test/ipfs-factory/tasks')
|
||||
|
||||
module.exports = {
|
||||
karma: {
|
||||
files: [{
|
||||
pattern: 'node_modules/interface-ipfs-core/test/fixtures/**/*',
|
||||
watched: false,
|
||||
served: true,
|
||||
included: false
|
||||
}]
|
||||
},
|
||||
hooks: {
|
||||
pre: factory.start,
|
||||
post: factory.stop
|
||||
}
|
||||
}
|
|
@ -1 +0,0 @@
|
|||
test/test-folder/ipfs-add.js
|
|
@ -1,32 +0,0 @@
|
|||
# Warning: This file is automatically synced from https://github.com/ipfs/ci-sync so if you want to change it, please change it there and ask someone to sync all repositories.
|
||||
sudo: false
|
||||
language: node_js
|
||||
|
||||
matrix:
|
||||
include:
|
||||
- node_js: 6
|
||||
env: CXX=g++-4.8
|
||||
- node_js: 8
|
||||
env: CXX=g++-4.8
|
||||
# - node_js: stable
|
||||
# env: CXX=g++-4.8
|
||||
|
||||
script:
|
||||
- npm run lint
|
||||
- npm run test
|
||||
- npm run coverage
|
||||
|
||||
before_script:
|
||||
- export DISPLAY=:99.0
|
||||
- sh -e /etc/init.d/xvfb start
|
||||
|
||||
after_success:
|
||||
- npm run coverage-publish
|
||||
|
||||
addons:
|
||||
firefox: 'latest'
|
||||
apt:
|
||||
sources:
|
||||
- ubuntu-toolchain-r-test
|
||||
packages:
|
||||
- g++-4.8
|
|
@ -1,238 +0,0 @@
|
|||
<a name="17.2.7"></a>
|
||||
## [17.2.7](https://github.com/ipfs/js-ipfs-api/compare/v17.2.6...v17.2.7) (2018-01-11)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* name and key tests ([#661](https://github.com/ipfs/js-ipfs-api/issues/661)) ([5ab1d02](https://github.com/ipfs/js-ipfs-api/commit/5ab1d02))
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* normalize KEY API ([#659](https://github.com/ipfs/js-ipfs-api/issues/659)) ([1b10821](https://github.com/ipfs/js-ipfs-api/commit/1b10821))
|
||||
* normalize NAME API ([#658](https://github.com/ipfs/js-ipfs-api/issues/658)) ([9b8ef48](https://github.com/ipfs/js-ipfs-api/commit/9b8ef48))
|
||||
|
||||
|
||||
|
||||
<a name="17.2.6"></a>
|
||||
## [17.2.6](https://github.com/ipfs/js-ipfs-api/compare/v17.2.5...v17.2.6) (2017-12-28)
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* support key/export and key/import ([#653](https://github.com/ipfs/js-ipfs-api/issues/653)) ([496f08e](https://github.com/ipfs/js-ipfs-api/commit/496f08e))
|
||||
|
||||
|
||||
|
||||
<a name="17.2.5"></a>
|
||||
## [17.2.5](https://github.com/ipfs/js-ipfs-api/compare/v17.2.4...v17.2.5) (2017-12-20)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* **files.add:** handle weird directory names ([#646](https://github.com/ipfs/js-ipfs-api/issues/646)) ([012b86c](https://github.com/ipfs/js-ipfs-api/commit/012b86c))
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* add files/flush ([#643](https://github.com/ipfs/js-ipfs-api/issues/643)) ([5c254eb](https://github.com/ipfs/js-ipfs-api/commit/5c254eb))
|
||||
* support key/rm and key/rename ([#641](https://github.com/ipfs/js-ipfs-api/issues/641)) ([113030a](https://github.com/ipfs/js-ipfs-api/commit/113030a))
|
||||
|
||||
|
||||
|
||||
<a name="17.2.4"></a>
|
||||
## [17.2.4](https://github.com/ipfs/js-ipfs-api/compare/v17.2.3...v17.2.4) (2017-12-06)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* stats/bw uses stream ([#640](https://github.com/ipfs/js-ipfs-api/issues/640)) ([c4e922e](https://github.com/ipfs/js-ipfs-api/commit/c4e922e))
|
||||
|
||||
|
||||
|
||||
<a name="17.2.3"></a>
|
||||
## [17.2.3](https://github.com/ipfs/js-ipfs-api/compare/v17.2.2...v17.2.3) (2017-12-05)
|
||||
|
||||
|
||||
|
||||
<a name="17.2.2"></a>
|
||||
## [17.2.2](https://github.com/ipfs/js-ipfs-api/compare/v17.2.1...v17.2.2) (2017-12-05)
|
||||
|
||||
|
||||
|
||||
<a name="17.2.1"></a>
|
||||
## [17.2.1](https://github.com/ipfs/js-ipfs-api/compare/v17.2.0...v17.2.1) (2017-12-05)
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* add the stat commands ([#639](https://github.com/ipfs/js-ipfs-api/issues/639)) ([76c3068](https://github.com/ipfs/js-ipfs-api/commit/76c3068))
|
||||
|
||||
|
||||
|
||||
<a name="17.2.0"></a>
|
||||
# [17.2.0](https://github.com/ipfs/js-ipfs-api/compare/v17.1.3...v17.2.0) (2017-12-01)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* propagate trailer errors correctly ([#636](https://github.com/ipfs/js-ipfs-api/issues/636)) ([62d733e](https://github.com/ipfs/js-ipfs-api/commit/62d733e))
|
||||
|
||||
|
||||
|
||||
<a name="17.1.3"></a>
|
||||
## [17.1.3](https://github.com/ipfs/js-ipfs-api/compare/v17.1.2...v17.1.3) (2017-11-23)
|
||||
|
||||
|
||||
|
||||
<a name="17.1.2"></a>
|
||||
## [17.1.2](https://github.com/ipfs/js-ipfs-api/compare/v17.1.1...v17.1.2) (2017-11-22)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* config.replace ([#634](https://github.com/ipfs/js-ipfs-api/issues/634)) ([79d79c5](https://github.com/ipfs/js-ipfs-api/commit/79d79c5)), closes [#633](https://github.com/ipfs/js-ipfs-api/issues/633)
|
||||
|
||||
|
||||
|
||||
<a name="17.1.1"></a>
|
||||
## [17.1.1](https://github.com/ipfs/js-ipfs-api/compare/v17.1.0...v17.1.1) (2017-11-22)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* pubsub do not eat error messages ([#632](https://github.com/ipfs/js-ipfs-api/issues/632)) ([5a1bf9b](https://github.com/ipfs/js-ipfs-api/commit/5a1bf9b))
|
||||
|
||||
|
||||
|
||||
<a name="17.1.0"></a>
|
||||
# [17.1.0](https://github.com/ipfs/js-ipfs-api/compare/v17.0.1...v17.1.0) (2017-11-20)
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* send files HTTP request should stream ([#629](https://github.com/ipfs/js-ipfs-api/issues/629)) ([dae62cb](https://github.com/ipfs/js-ipfs-api/commit/dae62cb))
|
||||
|
||||
|
||||
|
||||
<a name="17.0.1"></a>
|
||||
## [17.0.1](https://github.com/ipfs/js-ipfs-api/compare/v17.0.0...v17.0.1) (2017-11-20)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* allow topicCIDs from older peers ([#631](https://github.com/ipfs/js-ipfs-api/issues/631)) ([fe7cc22](https://github.com/ipfs/js-ipfs-api/commit/fe7cc22))
|
||||
|
||||
|
||||
|
||||
<a name="17.0.0"></a>
|
||||
# [17.0.0](https://github.com/ipfs/js-ipfs-api/compare/v16.0.0...v17.0.0) (2017-11-17)
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* Implementing the new interfaces ([#619](https://github.com/ipfs/js-ipfs-api/issues/619)) ([e1b38bf](https://github.com/ipfs/js-ipfs-api/commit/e1b38bf))
|
||||
|
||||
|
||||
|
||||
<a name="16.0.0"></a>
|
||||
# [16.0.0](https://github.com/ipfs/js-ipfs-api/compare/v15.1.0...v16.0.0) (2017-11-16)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* pubsub message fields ([#627](https://github.com/ipfs/js-ipfs-api/issues/627)) ([470777d](https://github.com/ipfs/js-ipfs-api/commit/470777d))
|
||||
|
||||
|
||||
|
||||
<a name="15.1.0"></a>
|
||||
# [15.1.0](https://github.com/ipfs/js-ipfs-api/compare/v15.0.2...v15.1.0) (2017-11-14)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* adapting HTTP API to the interface-ipfs-core spec ([#625](https://github.com/ipfs/js-ipfs-api/issues/625)) ([8e58225](https://github.com/ipfs/js-ipfs-api/commit/8e58225))
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* windows interop ([#624](https://github.com/ipfs/js-ipfs-api/issues/624)) ([40557d0](https://github.com/ipfs/js-ipfs-api/commit/40557d0))
|
||||
|
||||
|
||||
|
||||
<a name="15.0.2"></a>
|
||||
## [15.0.2](https://github.com/ipfs/js-ipfs-api/compare/v15.0.1...v15.0.2) (2017-11-13)
|
||||
|
||||
|
||||
|
||||
<a name="15.0.1"></a>
|
||||
## [15.0.1](https://github.com/ipfs/js-ipfs-api/compare/v15.0.0...v15.0.1) (2017-10-22)
|
||||
|
||||
|
||||
|
||||
<a name="15.0.0"></a>
|
||||
# [15.0.0](https://github.com/ipfs/js-ipfs-api/compare/v14.3.7...v15.0.0) (2017-10-22)
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* update pin API to match interface-ipfs-core ([9102643](https://github.com/ipfs/js-ipfs-api/commit/9102643))
|
||||
|
||||
|
||||
|
||||
<a name="14.3.7"></a>
|
||||
## [14.3.7](https://github.com/ipfs/js-ipfs-api/compare/v14.3.6...v14.3.7) (2017-10-18)
|
||||
|
||||
|
||||
|
||||
<a name="14.3.6"></a>
|
||||
## [14.3.6](https://github.com/ipfs/js-ipfs-api/compare/v14.3.5...v14.3.6) (2017-10-18)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* pass the config protocol to http requests ([#609](https://github.com/ipfs/js-ipfs-api/issues/609)) ([38d7289](https://github.com/ipfs/js-ipfs-api/commit/38d7289))
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* avoid doing multiple RPC requests for files.add, fixes [#522](https://github.com/ipfs/js-ipfs-api/issues/522) ([#595](https://github.com/ipfs/js-ipfs-api/issues/595)) ([0ea5f57](https://github.com/ipfs/js-ipfs-api/commit/0ea5f57))
|
||||
* report progress on ipfs add ([e2d894c](https://github.com/ipfs/js-ipfs-api/commit/e2d894c))
|
||||
|
||||
|
||||
|
||||
<a name="14.3.5"></a>
|
||||
## [14.3.5](https://github.com/ipfs/js-ipfs-api/compare/v14.3.4...v14.3.5) (2017-09-08)
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* Support specify hash algorithm in files.add ([#597](https://github.com/ipfs/js-ipfs-api/issues/597)) ([ed68657](https://github.com/ipfs/js-ipfs-api/commit/ed68657))
|
||||
|
||||
|
||||
|
||||
<a name="14.3.4"></a>
|
||||
## [14.3.4](https://github.com/ipfs/js-ipfs-api/compare/v14.3.3...v14.3.4) (2017-09-07)
|
||||
|
||||
|
||||
|
||||
<a name="14.3.3"></a>
|
||||
## [14.3.3](https://github.com/ipfs/js-ipfs-api/compare/v14.3.2...v14.3.3) (2017-09-07)
|
||||
|
||||
|
||||
### Features
|
||||
|
||||
* support options for .add / files.add ([8c717b2](https://github.com/ipfs/js-ipfs-api/commit/8c717b2))
|
||||
|
||||
|
||||
|
||||
<a name="14.3.2"></a>
|
||||
## [14.3.2](https://github.com/ipfs/js-ipfs-api/compare/v14.3.1...v14.3.2) (2017-09-04)
|
||||
|
||||
|
||||
### Bug Fixes
|
||||
|
||||
* new fixed aegir ([93ac472](https://github.com/ipfs/js-ipfs-api/commit/93ac472))
|
||||
|
||||
|
||||
|
|
@ -1,58 +0,0 @@
|
|||
# Contributing
|
||||
|
||||
## Setup
|
||||
|
||||
You should have [node.js] and [npm] installed.
|
||||
|
||||
## Linting
|
||||
|
||||
Linting is done using [eslint] and the rules are based on [standard].
|
||||
|
||||
```bash
|
||||
$ npm run lint
|
||||
```
|
||||
|
||||
## Tests
|
||||
|
||||
Tests in node
|
||||
|
||||
```bash
|
||||
$ npm run test:node
|
||||
```
|
||||
|
||||
Tests in the browser
|
||||
|
||||
```bash
|
||||
$ npm run test:browser
|
||||
```
|
||||
|
||||
## Building browser version
|
||||
|
||||
```bash
|
||||
$ npm run build
|
||||
```
|
||||
|
||||
## Releases
|
||||
|
||||
The `release` task will
|
||||
|
||||
1. Run a build
|
||||
2. Commit the build
|
||||
3. Bump the version in `package.json`
|
||||
4. Commit the version change
|
||||
5. Create a git tag
|
||||
6. Run `git push` to `upstream/master` (You can change this with `--remote my-remote`)
|
||||
|
||||
```bash
|
||||
# Major release
|
||||
$ npm run release-major
|
||||
# Minor relase
|
||||
$ npm run release-minor
|
||||
# Patch release
|
||||
$ npm run release
|
||||
```
|
||||
|
||||
[node.js]: https://nodejs.org/
|
||||
[npm]: http://npmjs.org/
|
||||
[eslint]: http://eslint.org/
|
||||
[standard]: https://github.com/feross/standard
|
|
@ -1,22 +0,0 @@
|
|||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2016 Protocol Labs, Inc.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
||||
|
|
@ -1,413 +0,0 @@
|
|||
<h1 align="center">
|
||||
<a href="ipfs.io"><img width="650px" src="https://ipfs.io/ipfs/QmQJ68PFMDdAsgCZvA1UVzzn18asVcf7HVvCDgpjiSCAse" alt="IPFS http client lib logo" /></a>
|
||||
</h1>
|
||||
|
||||
<h3 align="center">The JavaScript HTTP client library for IPFS implementations.</h3>
|
||||
|
||||
<p align="center">
|
||||
<a href="http://ipn.io"><img src="https://img.shields.io/badge/made%20by-Protocol%20Labs-blue.svg?style=flat-square" /></a>
|
||||
<a href="http://ipfs.io/"><img src="https://img.shields.io/badge/project-IPFS-blue.svg?style=flat-square" /></a>
|
||||
<a href="http://webchat.freenode.net/?channels=%23ipfs"><img src="https://img.shields.io/badge/freenode-%23ipfs-blue.svg?style=flat-square" /></a>
|
||||
<a href="https://waffle.io/ipfs/js-ipfs"><img src="https://img.shields.io/badge/pm-waffle-blue.svg?style=flat-square" /></a>
|
||||
<a href="https://github.com/ipfs/interface-ipfs-core"><img src="https://img.shields.io/badge/interface--ipfs--core-API%20Docs-blue.svg"></a>
|
||||
</p>
|
||||
|
||||
<p align="center">
|
||||
<a href="https://app.fossa.io/projects/git%2Bhttps%3A%2F%2Fgithub.com%2Fipfs%2Fjs-ipfs-api?ref=badge_small" alt="FOSSA Status"><img src="https://app.fossa.io/api/projects/git%2Bhttps%3A%2F%2Fgithub.com%2Fipfs%2Fjs-ipfs-api.svg?type=small"/></a>
|
||||
<a href="https://travis-ci.org/ipfs/js-ipfs-api"><img src="https://travis-ci.org/ipfs/js-ipfs-api.svg?branch=master" /></a>
|
||||
<a href="https://circleci.com/gh/ipfs/js-ipfs-api"><img src="https://circleci.com/gh/ipfs/js-ipfs-api.svg?style=svg" /></a>
|
||||
<a href="https://coveralls.io/github/ipfs/js-ipfs-api?branch=master"><img src="https://coveralls.io/repos/github/ipfs/js-ipfs-api/badge.svg?branch=master"></a>
|
||||
<br>
|
||||
<a href="https://david-dm.org/ipfs/js-ipfs-api"><img src="https://david-dm.org/ipfs/js-ipfs-api.svg?style=flat-square" /></a>
|
||||
<a href="https://github.com/feross/standard"><img src="https://img.shields.io/badge/code%20style-standard-brightgreen.svg?style=flat-square"></a>
|
||||
<a href="https://github.com/RichardLitt/standard-readme"><img src="https://img.shields.io/badge/standard--readme-OK-green.svg?style=flat-square" /></a>
|
||||
<a href=""><img src="https://img.shields.io/badge/npm-%3E%3D3.0.0-orange.svg?style=flat-square" /></a>
|
||||
<a href=""><img src="https://img.shields.io/badge/Node.js-%3E%3D6.0.0-orange.svg?style=flat-square" /></a>
|
||||
<br>
|
||||
</p>
|
||||
|
||||
> A client library for the IPFS HTTP API, implemented in JavaScript. This client library implements the [interface-ipfs-core](https://github.com/ipfs/interface-ipfs-core) enabling applications to change between a embebed js-ipfs node and any remote IPFS node without having to change the code. In addition, this client library implements a set of utility functions.
|
||||
|
||||
## Table of Contents
|
||||
|
||||
- [Install](#install)
|
||||
- [Running the daemon with the right port](#running-the-daemon-with-the-right-port)
|
||||
- [Importing the module and usage](#importing-the-module-and-usage)
|
||||
- [Importing a sub-module and usage](#importing-a-sub-module-and-usage)
|
||||
- [In a web browser through Browserify](#in-a-web-browser-through-browserify)
|
||||
- [In a web browser from CDN](#in-a-web-browser-from-cdn)
|
||||
- [CORS](#cors)
|
||||
- [Usage](#usage)
|
||||
- [API Docs](#api)
|
||||
- [Callbacks and promises](#callbacks-and-promises)
|
||||
- [Contribute](#contribute)
|
||||
- [License](#license)
|
||||
|
||||
## Install
|
||||
|
||||
This module uses node.js, and can be installed through npm:
|
||||
|
||||
```bash
|
||||
> npm install --save ipfs-api
|
||||
```
|
||||
|
||||
**Note:** ipfs-api requires Node.js v6 (LTS) or higher.
|
||||
|
||||
### Running the daemon with the right port
|
||||
|
||||
To interact with the API, you need to have a local daemon running. It needs to be open on the right port. `5001` is the default, and is used in the examples below, but it can be set to whatever you need.
|
||||
|
||||
```sh
|
||||
# Show the ipfs config API port to check it is correct
|
||||
> ipfs config Addresses.API
|
||||
/ip4/127.0.0.1/tcp/5001
|
||||
# Set it if it does not match the above output
|
||||
> ipfs config Addresses.API /ip4/127.0.0.1/tcp/5001
|
||||
# Restart the daemon after changing the config
|
||||
|
||||
# Run the daemon
|
||||
> ipfs daemon
|
||||
```
|
||||
|
||||
### Importing the module and usage
|
||||
|
||||
```javascript
|
||||
var ipfsAPI = require('ipfs-api')
|
||||
|
||||
// connect to ipfs daemon API server
|
||||
var ipfs = ipfsAPI('localhost', '5001', {protocol: 'http'}) // leaving out the arguments will default to these values
|
||||
|
||||
// or connect with multiaddr
|
||||
var ipfs = ipfsAPI('/ip4/127.0.0.1/tcp/5001')
|
||||
|
||||
// or using options
|
||||
var ipfs = ipfsAPI({host: 'localhost', port: '5001', protocol: 'http'})
|
||||
```
|
||||
### Importing a sub-module and usage
|
||||
```javascript
|
||||
const bitswap = require('ipfs-api/src/bitswap')('/ip4/127.0.0.1/tcp/5001')
|
||||
|
||||
bitswap.unwant(key, (err) => {
|
||||
// ...
|
||||
}
|
||||
```
|
||||
|
||||
### In a web browser through Browserify
|
||||
|
||||
Same as in Node.js, you just have to [browserify](http://browserify.org) the code before serving it. See the browserify repo for how to do that.
|
||||
|
||||
See the example in the [examples folder](/examples/bundle-browserify) to get a boilerplate.
|
||||
|
||||
### In a web browser through webpack
|
||||
|
||||
See the example in the [examples folder](/examples/bundle-webpack) to get an idea on how to use js-ipfs-api with webpack
|
||||
|
||||
### In a web browser from CDN
|
||||
|
||||
Instead of a local installation (and browserification) you may request a remote copy of IPFS API from [unpkg CDN](https://unpkg.com/).
|
||||
|
||||
To always request the latest version, use the following:
|
||||
|
||||
```html
|
||||
<script src="https://unpkg.com/ipfs-api/dist/index.js"></script>
|
||||
```
|
||||
|
||||
For maximum security you may also decide to:
|
||||
|
||||
* reference a specific version of IPFS API (to prevent unexpected breaking changes when a newer latest version is published)
|
||||
|
||||
* [generate a SRI hash](https://www.srihash.org/) of that version and use it to ensure integrity
|
||||
|
||||
* set the [CORS settings attribute](https://developer.mozilla.org/en-US/docs/Web/HTML/CORS_settings_attributes) to make anonymous requests to CDN
|
||||
|
||||
Example:
|
||||
|
||||
```html
|
||||
<script src="https://unpkg.com/ipfs-api@9.0.0/dist/index.js"
|
||||
integrity="sha384-5bXRcW9kyxxnSMbOoHzraqa7Z0PQWIao+cgeg327zit1hz5LZCEbIMx/LWKPReuB"
|
||||
crossorigin="anonymous"></script>
|
||||
```
|
||||
|
||||
CDN-based IPFS API provides the `IpfsApi` constructor as a method of the global `window` object. Example:
|
||||
|
||||
```
|
||||
var ipfs = window.IpfsApi('localhost', '5001')
|
||||
```
|
||||
|
||||
If you omit the host and port, the API will parse `window.host`, and use this information. This also works, and can be useful if you want to write apps that can be run from multiple different gateways:
|
||||
|
||||
```
|
||||
var ipfs = window.IpfsApi()
|
||||
```
|
||||
|
||||
### CORS
|
||||
|
||||
In a web browser IPFS API (either browserified or CDN-based) might encounter an error saying that the origin is not allowed. This would be a CORS ("Cross Origin Resource Sharing") failure: IPFS servers are designed to reject requests from unknown domains by default. You can whitelist the domain that you are calling from by changing your ipfs config like this:
|
||||
|
||||
```bash
|
||||
$ ipfs config --json API.HTTPHeaders.Access-Control-Allow-Origin "[\"http://example.com\"]"
|
||||
$ ipfs config --json API.HTTPHeaders.Access-Control-Allow-Credentials "[\"true\"]"
|
||||
$ ipfs config --json API.HTTPHeaders.Access-Control-Allow-Methods "[\"PUT\", \"POST\", \"GET\"]"
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
### API
|
||||
|
||||
[](https://github.com/ipfs/interface-ipfs-core)
|
||||
|
||||
> `js-ipfs-api` follows the spec defined by [`interface-ipfs-core`](https://github.com/ipfs/interface-ipfs-core), which concerns the interface to expect from IPFS implementations. This interface is a currently active endeavor. You can use it today to consult the methods available.
|
||||
|
||||
#### `Files`
|
||||
|
||||
- [files](https://github.com/ipfs/interface-ipfs-core/blob/master/SPEC/FILES.md)
|
||||
- [`ipfs.files.add(data, [options], [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#add). Alias to `ipfs.add`.
|
||||
- [`ipfs.files.addReadableStream([options])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#addreadablestream)
|
||||
- [`ipfs.files.addPullStream([options])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#addpullstream)
|
||||
- [`ipfs.files.cat(ipfsPath, [options], [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#cat). Alias to `ipfs.cat`.
|
||||
- [`ipfs.files.catReadableStream(ipfsPath, [options])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#catreadablestream)
|
||||
- [`ipfs.files.catPullStream(ipfsPath, [options])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#catpullstream)
|
||||
- [`ipfs.files.get(ipfsPath, [options], [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#get). Alias to `ipfs.get`.
|
||||
- [`ipfs.files.getReadableStream(ipfsPath, [options])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#getreadablestream)
|
||||
- [`ipfs.files.getPullStream(ipfsPath, [options])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/FILES.md#getpullstream)
|
||||
- `ipfs.ls`
|
||||
- MFS (mutable file system) specific:
|
||||
- `ipfs.files.cp`
|
||||
- `ipfs.files.ls`
|
||||
- `ipfs.files.mkdir`
|
||||
- `ipfs.files.stat`
|
||||
- `ipfs.files.rm`
|
||||
- `ipfs.files.read`
|
||||
- `ipfs.files.write`
|
||||
- `ipfs.files.mv`
|
||||
- `ipfs.files.flush(path, [callback])`
|
||||
|
||||
- [block](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/BLOCK.md)
|
||||
- [`ipfs.block.get(cid, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/BLOCK.md#get)
|
||||
- [`ipfs.block.put(block, cid, [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/BLOCK.md#put)
|
||||
- [`ipfs.block.stat(cid, [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/BLOCK.md#stat)
|
||||
|
||||
- repo
|
||||
- `ipfs.repo.stat()`
|
||||
- `ipfs.repo.gc()`
|
||||
|
||||
#### `Graph`
|
||||
|
||||
- [dag (not implemented, yet!)](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/DAG.md)
|
||||
- [`ipfs.dag.put(dagNode, options, callback)`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/DAG.md#dagput)
|
||||
- [`ipfs.dag.get(cid [, path, options], callback)`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/DAG.md#dagget)
|
||||
- [`ipfs.dag.tree(cid [, path, options], callback)`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/DAG.md#dagtree)
|
||||
|
||||
- [object](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md).
|
||||
- [`ipfs.object.new([template][, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectnew)
|
||||
- [`ipfs.object.put(obj, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectput)
|
||||
- [`ipfs.object.get(multihash, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectget)
|
||||
- [`ipfs.object.data(multihash, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectdata)
|
||||
- [`ipfs.object.links(multihash, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectlinks)
|
||||
- [`ipfs.object.stat(multihash, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectstat)
|
||||
- [`ipfs.object.patch.addLink(multihash, DAGLink, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectpatchaddlink)
|
||||
- [`ipfs.object.patch.rmLink(multihash, DAGLink, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectpatchrmlink)
|
||||
- [`ipfs.object.patch.appendData(multihash, data, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectpatchappenddata)
|
||||
- [`ipfs.object.patch.setData(multihash, data, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/OBJECT.md#objectpatchsetdata)
|
||||
- [pin](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/)
|
||||
- [`ipfs.pin.add()`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PIN.md#add)
|
||||
- [`ipfs.pin.rm()`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PIN.md#rm)
|
||||
- [`ipfs.pin.ls()`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PIN.md#ls)
|
||||
- [refs](https://github.com/ipfs/interface-ipfs-core/tree/master/API/refs)
|
||||
- [`ipfs.refs.local()`](https://github.com/ipfs/interface-ipfs-core/tree/master/API/refs#local)
|
||||
|
||||
|
||||
#### `Network`
|
||||
|
||||
- [bootstrap](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/)
|
||||
- `ipfs.bootstrap.list`
|
||||
- `ipfs.bootstrap.add`
|
||||
- `ipfs.bootstrap.rm`
|
||||
|
||||
- [bitswap](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/)
|
||||
- `ipfs.bitswap.wantlist()`
|
||||
- `ipfs.bitswap.stat()`
|
||||
- `ipfs.bitswap.unwant()`
|
||||
|
||||
- [dht](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/)
|
||||
- [`ipfs.dht.findprovs()`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/DHT.md#findprovs)
|
||||
- [`ipfs.dht.get()`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/DHT.md#get)
|
||||
- [`ipfs.dht.put()`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/DHT.md#put)
|
||||
|
||||
- [pubsub](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PUBSUB.md)
|
||||
- [`ipfs.pubsub.subscribe(topic, options, handler, callback)`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PUBSUB.md#pubsubsubscribe)
|
||||
- [`ipfs.pubsub.unsubscribe(topic, handler)`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PUBSUB.md#pubsubunsubscribe)
|
||||
- [`ipfs.pubsub.publish(topic, data, callback)`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PUBSUB.md#pubsubpublish)
|
||||
- [`ipfs.pubsub.ls(topic, callback)`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PUBSUB.md#pubsubls)
|
||||
- [`ipfs.pubsub.peers(topic, callback)`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/PUBSUB.md#pubsubpeers)
|
||||
|
||||
- [swarm](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/SWARM.md)
|
||||
- [`ipfs.swarm.addrs([callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/SWARM.md#addrs)
|
||||
- [`ipfs.swarm.connect(addr, [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/SWARM.md#connect)
|
||||
- [`ipfs.swarm.disconnect(addr, [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/SWARM.md#disconnect)
|
||||
- [`ipfs.swarm.peers([opts] [, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/SWARM.md#peers)
|
||||
|
||||
- [name](https://github.com/ipfs/interface-ipfs-core/tree/master/API/name)
|
||||
- [`ipfs.name.publish(addr, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/NAME.md#publish)
|
||||
- [`ipfs.name.resolve(addr, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/NAME.md#resolve)
|
||||
|
||||
#### `Node Management`
|
||||
|
||||
- [miscellaneous operations](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/MISCELLANEOUS.md)
|
||||
- [`ipfs.id([callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/MISCELLANEOUS.md#id)
|
||||
- [`ipfs.version([callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/MISCELLANEOUS.md#version)
|
||||
- [`ipfs.ping()`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/MISCELLANEOUS.md#ping)
|
||||
|
||||
- [config](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/CONFIG.md)
|
||||
- [`ipfs.config.get([key, callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/CONFIG.md#configget)
|
||||
- [`ipfs.config.set(key, value, [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/CONFIG.md#configset)
|
||||
- [`ipfs.config.replace(config, [callback])`](https://github.com/ipfs/interface-ipfs-core/tree/master/SPEC/CONFIG.md#configreplace)
|
||||
|
||||
- stats:
|
||||
- `ipfs.stats.bitswap([callback])`
|
||||
- `ipfs.stats.bw([options, callback])`
|
||||
- `ipfs.stats.repo([options, callback])`
|
||||
|
||||
- log:
|
||||
- `ipfs.log.ls([callback])`
|
||||
- `ipfs.log.tail([callback])`
|
||||
- `ipfs.log.level(subsystem, level, [options, callback])`
|
||||
|
||||
- key:
|
||||
- [`ipfs.key.gen(name, [options, callback])`](https://github.com/ipfs/interface-ipfs-core/blob/master/SPEC/KEY.md#javascript---ipfskeygenname-options-callback)
|
||||
- [`ipfs.key.list([options, callback])`](https://github.com/ipfs/interface-ipfs-core/blob/master/SPEC/KEY.md#javascript---ipfskeylistcallback)
|
||||
- [`ipfs.key.rm(name, [callback])`](https://github.com/ipfs/interface-ipfs-core/blob/master/SPEC/KEY.md#javascript---ipfskeyrmname-callback)
|
||||
- [`ipfs.key.rename(oldName, newName, [callback])`](https://github.com/ipfs/interface-ipfs-core/blob/master/SPEC/KEY.md#javascript---ipfskeyrenameoldname-newname-callback)
|
||||
- [`ipfs.key.export(name, password, [callback])`](https://github.com/ipfs/interface-ipfs-core/blob/master/SPEC/KEY.md#javascript---ipfskeyexportname-password-callback)
|
||||
- [`ipfs.key.import(name, pem, password, [callback])`](https://github.com/ipfs/interface-ipfs-core/blob/master/SPEC/KEY.md#javascript---ipfskeyimportname-pem-password-callback)
|
||||
|
||||
#### `Pubsub Caveat`
|
||||
|
||||
**Currently, the [PubSub API only works in Node.js envinroment](https://github.com/ipfs/js-ipfs-api/issues/518)**
|
||||
|
||||
We currently don't support pubsub when run in the browser, and we test it with separate set of tests to make sure if it's being used in the browser, pubsub errors.
|
||||
|
||||
More info: https://github.com/ipfs/js-ipfs-api/issues/518
|
||||
|
||||
This means:
|
||||
- You can use pubsub from js-ipfs-api in Node.js
|
||||
- You can use pubsub from js-ipfs-api in Electron
|
||||
(when js-ipfs-api is ran in the main process of Electron)
|
||||
- You can't use pubsub from js-ipfs-api in the browser
|
||||
- You can't use pubsub from js-ipfs-api in Electron's
|
||||
renderer process
|
||||
- You can use pubsub from js-ipfs in the browsers
|
||||
- You can use pubsub from js-ipfs in Node.js
|
||||
- You can use pubsub from js-ipfs in Electron
|
||||
(in both the main process and the renderer process)
|
||||
- See https://github.com/ipfs/js-ipfs for details on
|
||||
pubsub in js-ipfs
|
||||
|
||||
#### `Utility functions`
|
||||
|
||||
Adding to the methods defined by [`interface-ipfs-core`](https://github.com/ipfs/interface-ipfs-core), `js-ipfs-api` exposes a set of extra utility methods. These utility functions are scoped behind the `ipfs.util`.
|
||||
|
||||
Complete documentation for these methods is coming with: https://github.com/ipfs/js-ipfs-api/pull/305
|
||||
|
||||
##### Add files or entire directories from the FileSystem to IPFS
|
||||
|
||||
> `ipfs.util.addFromFs(path, option, callback)`
|
||||
|
||||
Reads a file or folder from `path` on the filesystem and adds it to IPFS. Options:
|
||||
- **recursive**: If `path` is a directory, use option `{ recursive: true }` to add the directory and all its sub-directories.
|
||||
- **ignore**: To exclude fileglobs from the directory, use option `{ ignore: ['ignore/this/folder/**', 'and/this/file'] }`.
|
||||
- **hidden**: hidden/dot files (files or folders starting with a `.`, for example, `.git/`) are not included by default. To add them, use the option `{ hidden: true }`.
|
||||
|
||||
```JavaScript
|
||||
ipfs.util.addFromFs('path/to/a/folder', { recursive: true , ignore: ['subfolder/to/ignore/**']}, (err, result) => {
|
||||
if (err) { throw err }
|
||||
console.log(result)
|
||||
})
|
||||
```
|
||||
|
||||
`result` is an array of objects describing the files that were added, such as:
|
||||
|
||||
```
|
||||
[
|
||||
{
|
||||
path: 'test-folder',
|
||||
hash: 'QmRNjDeKStKGTQXnJ2NFqeQ9oW23WcpbmvCVrpDHgDg3T6',
|
||||
size: 2278
|
||||
},
|
||||
// ...
|
||||
]
|
||||
```
|
||||
|
||||
##### Add a file from a URL to IPFS
|
||||
|
||||
> `ipfs.util.addFromURL(url, callback)`
|
||||
|
||||
```JavaScript
|
||||
ipfs.util.addFromURL('http://example.com/', (err, result) => {
|
||||
if (err) {
|
||||
throw err
|
||||
}
|
||||
console.log(result)
|
||||
})
|
||||
|
||||
```
|
||||
|
||||
##### Add a file from a stream to IPFS
|
||||
|
||||
> `ipfs.util.addFromStream(stream, callback)`
|
||||
|
||||
This is very similar to `ipfs.files.add({path:'', content: stream})`. It is like the reverse of cat
|
||||
|
||||
```JavaScript
|
||||
ipfs.util.addFromStream(<readable-stream>, (err, result) => {
|
||||
if (err) {
|
||||
throw err
|
||||
}
|
||||
console.log(result)
|
||||
})
|
||||
```
|
||||
|
||||
### Callbacks and Promises
|
||||
|
||||
If you do not pass in a callback all API functions will return a `Promise`. For example:
|
||||
|
||||
```js
|
||||
ipfs.id()
|
||||
.then((id) => {
|
||||
console.log('my id is: ', id)
|
||||
})
|
||||
.catch((err) => {
|
||||
console.log('Fail: ', err)
|
||||
})
|
||||
```
|
||||
|
||||
This relies on a global `Promise` object. If you are in an environment where that is not yet available you need to bring your own polyfill.
|
||||
|
||||
## Development
|
||||
|
||||
### Testing
|
||||
|
||||
We run tests by executing `npm test` in a terminal window. This will run both Node.js and Browser tests, both in Chrome and PhantomJS. To ensure that the module conforms with the [`interface-ipfs-core`](https://github.com/ipfs/interface-ipfs-core) spec, we run the batch of tests provided by the interface module, which can be found [here](https://github.com/ipfs/interface-ipfs-core/tree/master/src).
|
||||
|
||||
## Contribute
|
||||
|
||||
The js-ipfs-api is a work in progress. As such, there's a few things you can do right now to help out:
|
||||
|
||||
* **[Check out the existing issues](https://github.com/ipfs/js-ipfs-api/issues)**!
|
||||
* **Perform code reviews**. More eyes will help a) speed the project along b) ensure quality and c) reduce possible future bugs.
|
||||
* **Add tests**. There can never be enough tests. Note that interface tests exist inside [`interface-ipfs-core`](https://github.com/ipfs/interface-ipfs-core/tree/master/src).
|
||||
* **Contribute to the [FAQ repository](https://github.com/ipfs/faq/issues)** with any questions you have about IPFS or any of the relevant technology. A good example would be asking, 'What is a merkledag tree?'. If you don't know a term, odds are, someone else doesn't either. Eventually, we should have a good understanding of where we need to improve communications and teaching together to make IPFS and IPN better.
|
||||
|
||||
**Want to hack on IPFS?**
|
||||
|
||||
[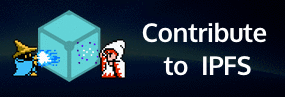](https://github.com/ipfs/community/blob/master/contributing.md)
|
||||
|
||||
## Historical context
|
||||
|
||||
This module started as a direct mapping from the go-ipfs cli to a JavaScript implementation, although this was useful and familiar to a lot of developers that were coming to IPFS for the first time, it also created some confusion on how to operate the core of IPFS and have access to the full capacity of the protocol. After much consideration, we decided to create `interface-ipfs-core` with the goal of standardizing the interface of a core implementation of IPFS, and keep the utility functions the IPFS community learned to use and love, such as reading files from disk and storing them directly to IPFS.
|
||||
|
||||
## License
|
||||
|
||||
[MIT](LICENSE)
|
||||
|
||||
[](https://app.fossa.io/projects/git%2Bhttps%3A%2F%2Fgithub.com%2Fipfs%2Fjs-ipfs-api?ref=badge_large)
|
|
@ -1,29 +0,0 @@
|
|||
# Warning: This file is automatically synced from https://github.com/ipfs/ci-sync so if you want to change it, please change it there and ask someone to sync all repositories.
|
||||
version: "{build}"
|
||||
|
||||
environment:
|
||||
matrix:
|
||||
- nodejs_version: "6"
|
||||
- nodejs_version: "8"
|
||||
|
||||
matrix:
|
||||
fast_finish: true
|
||||
|
||||
install:
|
||||
# Install Node.js
|
||||
- ps: Install-Product node $env:nodejs_version
|
||||
|
||||
# Upgrade npm
|
||||
- npm install -g npm
|
||||
|
||||
# Output our current versions for debugging
|
||||
- node --version
|
||||
- npm --version
|
||||
|
||||
# Install our package dependencies
|
||||
- npm install
|
||||
|
||||
test_script:
|
||||
- npm run test:node
|
||||
|
||||
build: off
|
|
@ -1,2 +0,0 @@
|
|||
// Warning: This file is automatically synced from https://github.com/ipfs/ci-sync so if you want to change it, please change it there and ask someone to sync all repositories.
|
||||
javascript()
|
|
@ -1,19 +0,0 @@
|
|||
# Warning: This file is automatically synced from https://github.com/ipfs/ci-sync so if you want to change it, please change it there and ask someone to sync all repositories.
|
||||
machine:
|
||||
node:
|
||||
version: stable
|
||||
|
||||
test:
|
||||
post:
|
||||
- npm run coverage -- --upload --providers coveralls
|
||||
|
||||
dependencies:
|
||||
pre:
|
||||
- google-chrome --version
|
||||
- curl -L -o google-chrome.deb https://dl.google.com/linux/direct/google-chrome-stable_current_amd64.deb
|
||||
- sudo dpkg -i google-chrome.deb || true
|
||||
- sudo apt-get update
|
||||
- sudo apt-get install -f
|
||||
- sudo apt-get install --only-upgrade lsb-base
|
||||
- sudo dpkg -i google-chrome.deb
|
||||
- google-chrome --version
|
|
@ -1,91 +0,0 @@
|
|||
<!doctype html>
|
||||
<html><head><meta charset="utf-8"/><meta name="viewport" content="width=device-width, initial-scale=1.0"/><title>ipfs-api - Documentation</title><link href="https://fonts.googleapis.com/css?family=Roboto+Mono:400,500|Roboto:300,500,700" rel="stylesheet"/><style>body{margin: 0;
|
||||
padding: 0;
|
||||
color: #000;
|
||||
font-family: Roboto, sans-serif;
|
||||
font-weight: 300;
|
||||
line-height: 26px;
|
||||
font-size: 17px;}*{-moz-box-sizing: border-box;
|
||||
box-sizing: border-box;}</style></head><body><main id="app"><div data-radium="true"><div style="position:fixed;box-shadow:0px 2px 3px 0px rgba(0, 0, 0, 0.25);width:100%;min-width:100%;margin-left:0px;margin-right:0px;height:65px;z-index:99;background:#FFFFFF;padding-top:10px;padding-bottom:10px;padding-left:20px;padding-right:20px;" data-radium="true"><div style="margin-right:auto;margin-left:auto;padding-left:15px;padding-right:15px;" class="rmq-87b609c0 rmq-159a7c96 rmq-27601239" data-radium="true"><div style="margin-left:-15px;margin-right:-15px;" data-radium="true"><div style="position:relative;min-height:1px;padding-left:15px;padding-right:15px;float:left;width:50.00000%;" class="rmq-aa1729d5 rmq-a22eaf97 rmq-1918856d rmq-6156e77b rmq-21ab0466 rmq-a01b93a4 rmq-d226cb7e rmq-5cc71c8" data-radium="true"><svg fill="currentColor" preserveAspectRatio="xMidYMid meet" height="1em" width="1em" viewBox="0 0 40 40" style="vertical-align:inherit;font-size:36px;margin-right:20px;"><g><path d="m30 22.5h-5c-1.3 0-2.5 1.3-2.5 2.5h10c0-1.3-1.2-2.5-2.5-2.5z m-2.1-16.2c-6.6 0-8.2 1.2-9.1 2.1-1-0.9-2.6-2.1-9.2-2.1s-9.6 1.7-9.6 3v22.9c1.1-0.6 4.6-1.9 8.4-2.2 4.4-0.4 9.1 0.4 9.1 1.2 0 0.7 0.3 1.3 1.2 1.3h0.1c0.9 0 1.2-0.6 1.2-1.2 0-0.9 4.6-1.7 9.1-1.3 3.7 0.3 7.3 1.6 8.4 2.2v-22.9c0-1.3-3.1-3-9.6-3z m-10.4 22.3c-1.2-0.6-4-1.1-7.5-1.1s-6.6 0.5-7.5 1.1v-17.3s2.5-2.4 7.5-2.4 7.5 1.1 7.5 2.4v17.3z m17.5 0c-0.9-0.6-4-1.1-7.5-1.1s-6.3 0.5-7.5 1.1v-17.3s2.5-2.4 7.5-2.4 7.5 1.1 7.5 2.4v17.3z m-5-11.1h-5c-1.3 0-2.5 1.3-2.5 2.5h10c0-1.3-1.2-2.5-2.5-2.5z m0-5h-5c-1.3 0-2.5 1.3-2.5 2.5h10c0-1.3-1.2-2.5-2.5-2.5z m-17.5 5h-5c-1.2 0-2.5 1.2-2.5 2.5h10c0-1.2-1.2-2.5-2.5-2.5z m0 5h-5c-1.2 0-2.5 1.2-2.5 2.5h10c0-1.2-1.2-2.5-2.5-2.5z m0-10h-5c-1.2 0-2.5 1.2-2.5 2.5h10c0-1.2-1.2-2.5-2.5-2.5z"></path></g></svg><div style="display:inline-block;" data-radium="true"><div style="text-transform:uppercase;font-family:Roboto, sans-serif;" data-radium="true">ipfs-api</div><div style="font-family:Roboto Mono, Menlo, Monaco, Courier, monospace;font-weight:300;" data-radium="true">17.2.7</div></div></div><div style="position:relative;min-height:1px;padding-left:15px;padding-right:15px;float:right !important;width:25.00000%;text-align:right;font-size:36px;" class="rmq-aa1729d5 rmq-a22eaf97 rmq-1918856d rmq-6156e77b rmq-77267d84 rmq-5c0c1446 rmq-4605701c rmq-34ee8e6a" data-radium="true"><a href="https://github.com/ipfs/js-ipfs-api"><svg fill="currentColor" preserveAspectRatio="xMidYMid meet" height="1em" width="1em" viewBox="0 0 40 40" style="vertical-align:middle;"><g><path d="m20 0c-11 0-20 9-20 20 0 8.8 5.7 16.3 13.7 19 1 0.2 1.3-0.5 1.3-1 0-0.5 0-2 0-3.7-5.5 1.2-6.7-2.4-6.7-2.4-0.9-2.3-2.2-2.9-2.2-2.9-1.9-1.2 0.1-1.2 0.1-1.2 2 0.1 3.1 2.1 3.1 2.1 1.7 3 4.6 2.1 5.8 1.6 0.2-1.3 0.7-2.2 1.3-2.7-4.5-0.5-9.2-2.2-9.2-9.8 0-2.2 0.8-4 2.1-5.4-0.2-0.5-0.9-2.6 0.2-5.3 0 0 1.7-0.5 5.5 2 1.6-0.4 3.3-0.6 5-0.6 1.7 0 3.4 0.2 5 0.7 3.8-2.6 5.5-2.1 5.5-2.1 1.1 2.8 0.4 4.8 0.2 5.3 1.3 1.4 2.1 3.2 2.1 5.4 0 7.6-4.7 9.3-9.2 9.8 0.7 0.6 1.4 1.9 1.4 3.7 0 2.7 0 4.9 0 5.5 0 0.6 0.3 1.2 1.3 1 8-2.7 13.7-10.2 13.7-19 0-11-9-20-20-20z"></path></g></svg></a></div><div style="clear:both;" data-radium="true"></div></div><div style="clear:both;" data-radium="true"></div></div></div><div style="margin-right:auto;margin-left:auto;padding-left:15px;padding-right:15px;padding-top:104px;" class="rmq-87b609c0 rmq-159a7c96 rmq-27601239" data-radium="true"><div style="margin-left:-15px;margin-right:-15px;" data-radium="true"><div style="position:fixed;min-height:1px;padding-left:15px;padding-right:15px;float:left;width:8.33333%;height:80%;max-width:300px;" class="rmq-aa1729d5 rmq-a22eaf97 rmq-1918856d rmq-6156e77b rmq-5c4c5b38 rmq-5c0c1446 rmq-4605701c rmq-34ee8e6a rmq-35757987 rmq-f09e82ae" data-radium="true"><div style="font-family:Roboto, sans-serif;border-radius:4px;padding-top:0;padding-bottom:0;margin-top:26px;border:1px solid #e6e9ed;font-weight:500;font-size:15px;line-height:18px;max-height:70vh;overflow-x:auto;" data-radium="true"><ul style="list-style:none;margin-top:0;margin-bottom:0;padding-left:0;" data-radium="true"><div data-radium="true"><li style="padding-left:20px;padding-right:20px;padding-top:13px;padding-bottom:13px;border-bottom:none;" data-radium="true"><a style="color:#000;" href="#intro" data-radium="true">Intro</a></li></div></ul></div></div><div style="position:relative;min-height:1px;padding-left:15px;padding-right:15px;float:left;width:100.00000%;" class="rmq-aa1729d5 rmq-a22eaf97 rmq-1918856d rmq-6156e77b rmq-89bb2272 rmq-2d0e68c6 rmq-3752549c rmq-3b9282ea rmq-4d29a8df rmq-ea7683a5 rmq-ad95cc33" data-radium="true"><div class="content" data-radium="true"><style>h1, h2, h3, h4, h5, h6{font-family: Roboto, sans-serif;
|
||||
font-weight: 300;}h1{font-size: 48px;
|
||||
line-height: 72px;}h2{font-size: 24px;
|
||||
line-height: 36px;}h3{font-size: 19px;
|
||||
line-height: 29px;
|
||||
font-weight: 400;}h4{font-size: 17px;
|
||||
line-height: 22px;
|
||||
font-weight: 400;}a{text-decoration: none;
|
||||
color: #00AAFF;
|
||||
:hover: [object Object];}a.anchor:before{content: "";
|
||||
display: block;
|
||||
height: 100px;
|
||||
margin: -80px 0 0;}.content blockquote{padding-left: 20px;
|
||||
margin: 0;
|
||||
border-left: 4px solid #eee;}.content ul, content li{list-style: none;}.content ul li:before{color: #CCC;
|
||||
float: left;
|
||||
margin-left: -20px;
|
||||
margin-top: 1px;
|
||||
content: "•";}pre.hljs, pre > code{margin-bottom: 30px;
|
||||
font-family: Roboto Mono, Menlo, Monaco, Courier, monospace;
|
||||
padding: 12px 15px 12px 15px;
|
||||
border-radius: 4px;
|
||||
box-shadow: inset 0 0 0 1px rgba(0, 0, 0, 0.1);
|
||||
display: block;
|
||||
position: relative;
|
||||
overflow-x: auto;
|
||||
font-size: 13px;
|
||||
color: #666;
|
||||
white-space: pre;}code{font-family: Roboto Mono, Menlo, Monaco, Courier, monospace;
|
||||
display: inline;
|
||||
font-size: 13px;
|
||||
font-weight: 400;
|
||||
margin: 0 2px;
|
||||
padding: 1px 6px;
|
||||
box-shadow: 0 0 0 1px #DDD;
|
||||
white-space: nowrap;
|
||||
border-radius: 4px;}.hljs{display: block;
|
||||
overflow-x: auto;}.hljs-comment, .hljs-quote{color: #998;
|
||||
font-style: italic;}.hljs-keyword, .hljs-selector-tag, .hljs-subst{color: #333;
|
||||
font-weight: bold;}.hljs-number, .hljs-literal, .hljs-variable, .hljs-template-variable, .hljs-tag .hljs-attr{color: #008080;}.hljs-string, .hljs-doctag{color: #d14;}.hljs-title, .hljs-section, .hljs-selector-id{color: #900;
|
||||
font-weight: bold;}.hljs-subst{font-weight: normal;}.hljs-type, .hljs-class .hljs-title{color: #458;
|
||||
font-weight: bold;}.hljs-tag, .hljs-name, .hljs-attribute{color: #000080;
|
||||
font-weight: normal;}.hljs-regexp, .hljs-link{color: #009926;}.hljs-symbol, .hljs-bullet{color: #990073;}.hljs-built_in, .hljs-builtin-name{color: #0086b3;}.hljs-meta{color: #999;
|
||||
font-weight: bold;}.hljs-deletion{background: #fdd;}.hljs-addition{background: #dfd;}.hljs-emphasis{font-style: italic;}.hljs-strong{font-weight: bold;}</style><div style="margin-bottom:104px;" data-radium="true"><h1 data-radium="true"><a class="anchor" name="intro"></a>Intro</h1><div><p>Installable via <code>npm install --save ipfs-api</code>, it can also be used directly in the browser.</p>
|
||||
<h2>Download</h2>
|
||||
<p>The source is available for download from <a href="https://github.com/ipfs/js-ipfs-api">GitHub</a>. Alternatively, you can install using npm:</p>
|
||||
<pre class='hljs'>$ npm install --save ipfs-api</pre>
|
||||
<p>You can then <code>require()</code> ipfs-api as normal:</p>
|
||||
<pre class='hljs'><span class="hljs-keyword">const</span> ipfsApi = <span class="hljs-built_in">require</span>(<span class="hljs-string">'ipfs-api'</span>)</pre>
|
||||
<h2>In the Browser</h2>
|
||||
<p>Ipfs-api should work in any ES2015 environment out of the box.</p>
|
||||
<p>Usage:</p>
|
||||
<pre class='hljs'><span class="hljs-tag"><<span class="hljs-name">script</span> <span class="hljs-attr">type</span>=<span class="hljs-string">"text/javascript"</span> <span class="hljs-attr">src</span>=<span class="hljs-string">"index.js"</span>></span><span class="undefined"></span><span class="hljs-tag"></<span class="hljs-name">script</span>></span></pre>
|
||||
<p>The portable versions of ipfs-api, including <code>index.js</code> and <code>index.min.js</code>, are included in the <code>/dist</code> folder. Ipfs-api can also be found on <a href="https://unpkg.com">unkpkg.com</a> under</p>
|
||||
<ul>
|
||||
<li><a href="https://unpkg.com/ipfs-api/dist/index.min.js">https://unpkg.com/ipfs-api/dist/index.min.js</a></li>
|
||||
<li><a href="https://unpkg.com/ipfs-api/dist/index.js">https://unpkg.com/ipfs-api/dist/index.js</a></li>
|
||||
</ul>
|
||||
</div></div></div></div><div style="clear:both;" data-radium="true"></div></div><div style="clear:both;" data-radium="true"></div></div><style>@media (min-width: 768px) and (max-width: 991px){ .rmq-87b609c0{width: 750px !important;}}
|
||||
@media (min-width: 992px) and (max-width: 1199px){ .rmq-159a7c96{width: 970px !important;}}
|
||||
@media (min-width: 1200px){ .rmq-27601239{width: 1170px !important;}}
|
||||
@media (min-width: 480px) and (max-width: 767px){ .rmq-aa1729d5{float: left !important;}}
|
||||
@media (min-width: 768px) and (max-width: 991px){ .rmq-a22eaf97{float: left !important;}}
|
||||
@media (min-width: 992px) and (max-width: 1199px){ .rmq-1918856d{float: left !important;}}
|
||||
@media (min-width: 1200px){ .rmq-6156e77b{float: left !important;}}
|
||||
@media (min-width: 480px) and (max-width: 767px) { .rmq-21ab0466{width: 50.00000% !important;}}
|
||||
@media (min-width: 768px) and (max-width: 991px) { .rmq-a01b93a4{width: 50.00000% !important;}}
|
||||
@media (min-width: 992px) and (max-width: 1199px) { .rmq-d226cb7e{width: 50.00000% !important;}}
|
||||
@media (min-width: 1200px) { .rmq-5cc71c8{width: 50.00000% !important;}}
|
||||
@media (min-width: 480px) and (max-width: 767px) { .rmq-77267d84{width: 25.00000% !important;}}
|
||||
@media (min-width: 768px) and (max-width: 991px) { .rmq-5c0c1446{width: 25.00000% !important;}}
|
||||
@media (min-width: 992px) and (max-width: 1199px) { .rmq-4605701c{width: 25.00000% !important;}}
|
||||
@media (min-width: 1200px) { .rmq-34ee8e6a{width: 25.00000% !important;}}
|
||||
@media (min-width: 480px) and (max-width: 767px) { .rmq-5c4c5b38{width: 8.33333% !important;}}
|
||||
@media (max-width: 479px){ .rmq-35757987{display: none !important;}}
|
||||
@media (min-width: 480px) and (max-width: 767px) { .rmq-f09e82ae{display: none !important;}}
|
||||
@media (min-width: 480px) and (max-width: 767px) { .rmq-89bb2272{width: 100.00000% !important;}}
|
||||
@media (min-width: 768px) and (max-width: 991px) { .rmq-2d0e68c6{width: 66.66667% !important;}}
|
||||
@media (min-width: 992px) and (max-width: 1199px) { .rmq-3752549c{width: 66.66667% !important;}}
|
||||
@media (min-width: 1200px) { .rmq-3b9282ea{width: 66.66667% !important;}}
|
||||
@media (min-width: 768px) and (max-width: 991px) { .rmq-4d29a8df{left: 33.33333% !important;}}
|
||||
@media (min-width: 992px) and (max-width: 1199px) { .rmq-ea7683a5{left: 33.33333% !important;}}
|
||||
@media (min-width: 1200px) { .rmq-ad95cc33{left: 33.33333% !important;}}</style></div></main></body></html>
|
|
@ -1,35 +0,0 @@
|
|||
# Bundle js-ipfs-api with Browserify!
|
||||
|
||||
> In this example, you will find a boilerplate you can use to guide yourself into bundling js-ipfs-api with browserify, so that you can use it in your own web app!
|
||||
|
||||
## Setup
|
||||
|
||||
As for any js-ipfs-api example, **you need a running IPFS daemon**, you learn how to do that here:
|
||||
|
||||
- [Spawn a go-ipfs daemon](https://ipfs.io/docs/getting-started/)
|
||||
- [Spawn a js-ipfs daemon](https://github.com/ipfs/js-ipfs#usage)
|
||||
|
||||
**Note:** If you load your app from a different domain than the one the daemon is running (most probably), you will need to set up CORS, see https://github.com/ipfs/js-ipfs-api#cors to learn how to do that.
|
||||
|
||||
A quick (and dirty way to get it done) is:
|
||||
|
||||
```bash
|
||||
> ipfs config --json API.HTTPHeaders.Access-Control-Allow-Origin "[\"*\"]"
|
||||
> ipfs config --json API.HTTPHeaders.Access-Control-Allow-Credentials "[\"true\"]"
|
||||
```
|
||||
|
||||
## Run this example
|
||||
|
||||
Once the daemon is on, run the following commands within this folder:
|
||||
|
||||
```bash
|
||||
> npm install
|
||||
> npm start
|
||||
```
|
||||
|
||||
Now open your browser at `http://localhost:8888`
|
||||
|
||||
You should see the following:
|
||||
|
||||

|
||||

|
Binary file not shown.
Before Width: | Height: | Size: 98 KiB |
Binary file not shown.
Before Width: | Height: | Size: 143 KiB |
|
@ -1,26 +0,0 @@
|
|||
<!doctype html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8"/>
|
||||
<title>JS IPFS API - Example - Browser - Add</title>
|
||||
<script src="bundle.js"></script>
|
||||
<style>
|
||||
.content {
|
||||
border: 1px solid black;
|
||||
padding: 10px;
|
||||
margin: 5px 0;
|
||||
}
|
||||
</style>
|
||||
</head>
|
||||
<body>
|
||||
<h1>JS IPFS API - Add data to IPFS from the browser</h1>
|
||||
<textarea id="source">
|
||||
</textarea>
|
||||
<button id="store">add to ipfs</button>
|
||||
<div>
|
||||
<div>found in ipfs:</div>
|
||||
<div class="content" id="hash">[ipfs hash]</div>
|
||||
<div class="content" id="content">[ipfs content]</div>
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
|
@ -1,37 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
var IPFS = require('ipfs-api')
|
||||
|
||||
var ipfs = IPFS()
|
||||
|
||||
function store () {
|
||||
var toStore = document.getElementById('source').value
|
||||
ipfs.add(Buffer.from(toStore), function (err, res) {
|
||||
if (err || !res) {
|
||||
return console.error('ipfs add error', err, res)
|
||||
}
|
||||
|
||||
res.forEach(function (file) {
|
||||
if (file && file.hash) {
|
||||
console.log('successfully stored', file.hash)
|
||||
display(file.hash)
|
||||
}
|
||||
})
|
||||
})
|
||||
}
|
||||
|
||||
function display (hash) {
|
||||
// buffer: true results in the returned result being a buffer rather than a stream
|
||||
ipfs.cat(hash, {buffer: true}, function (err, res) {
|
||||
if (err || !res) {
|
||||
return console.error('ipfs cat error', err, res)
|
||||
}
|
||||
|
||||
document.getElementById('hash').innerText = hash
|
||||
document.getElementById('content').innerText = res.toString()
|
||||
})
|
||||
}
|
||||
|
||||
document.addEventListener('DOMContentLoaded', function () {
|
||||
document.getElementById('store').onclick = store
|
||||
})
|
|
@ -1,19 +0,0 @@
|
|||
{
|
||||
"name": "bundle-browserify",
|
||||
"version": "1.0.0",
|
||||
"description": "Bundle js-ipfs-api with Browserify",
|
||||
"main": "index.js",
|
||||
"scripts": {
|
||||
"start": "browserify index.js > bundle.js && http-server -a 127.0.0.1 -p 8888"
|
||||
},
|
||||
"keywords": [],
|
||||
"author": "Friedel Ziegelmayer",
|
||||
"license": "MIT",
|
||||
"devDependencies": {
|
||||
"browserify": "^13.1.1",
|
||||
"ipfs-api": "^11.1.0",
|
||||
"http-server": "^0.9.0"
|
||||
},
|
||||
"dependencies": {
|
||||
}
|
||||
}
|
|
@ -1,3 +0,0 @@
|
|||
{
|
||||
"stage": 0
|
||||
}
|
|
@ -1,11 +0,0 @@
|
|||
{
|
||||
"extends": "standard",
|
||||
"rules": {
|
||||
"react/jsx-uses-react": 2,
|
||||
"react/jsx-uses-vars": 2,
|
||||
"react/react-in-jsx-scope": 2
|
||||
},
|
||||
"plugins": [
|
||||
"react"
|
||||
]
|
||||
}
|
Binary file not shown.
Before Width: | Height: | Size: 109 KiB |
|
@ -1,35 +0,0 @@
|
|||
# Bundle js-ipfs-api with Webpack!
|
||||
|
||||
> In this example, you will find a boilerplate you can use to guide yourself into bundling js-ipfs-api with webpack, so that you can use it in your own web app!
|
||||
|
||||
## Setup
|
||||
|
||||
As for any js-ipfs-api example, **you need a running IPFS daemon**, you learn how to do that here:
|
||||
|
||||
- [Spawn a go-ipfs daemon](https://ipfs.io/docs/getting-started/)
|
||||
- [Spawn a js-ipfs daemon](https://github.com/ipfs/js-ipfs#usage)
|
||||
|
||||
**Note:** If you load your app from a different domain than the one the daemon is running (most probably), you will need to set up CORS, see https://github.com/ipfs/js-ipfs-api#cors to learn how to do that.
|
||||
|
||||
A quick (and dirty way to get it done) is:
|
||||
|
||||
```bash
|
||||
> ipfs config --json API.HTTPHeaders.Access-Control-Allow-Origin "[\"*\"]"
|
||||
> ipfs config --json API.HTTPHeaders.Access-Control-Allow-Credentials "[\"true\"]"
|
||||
```
|
||||
|
||||
## Run this example
|
||||
|
||||
Once the daemon is on, run the following commands within this folder:
|
||||
|
||||
```bash
|
||||
> npm install
|
||||
> npm start
|
||||
```
|
||||
|
||||
Now open your browser at `http://localhost:3000`
|
||||
|
||||
You should see the following:
|
||||
|
||||

|
||||
|
|
@ -1,10 +0,0 @@
|
|||
<html>
|
||||
<head>
|
||||
<title>Sample App</title>
|
||||
</head>
|
||||
<body>
|
||||
<div id='root'>
|
||||
</div>
|
||||
<script src="/static/bundle.js"></script>
|
||||
</body>
|
||||
</html>
|
|
@ -1,21 +0,0 @@
|
|||
{
|
||||
"name": "bundle-webpack",
|
||||
"version": "1.0.0",
|
||||
"description": "Bundle js-ipfs-api with Webpack",
|
||||
"scripts": {
|
||||
"start": "node server.js"
|
||||
},
|
||||
"author": "Victor Bjelkholm <victor@ipfs.io>",
|
||||
"license": "MIT",
|
||||
"keywords": [],
|
||||
"devDependencies": {
|
||||
"babel-core": "^5.4.7",
|
||||
"babel-loader": "^5.1.2",
|
||||
"ipfs-api": "^11.1.0",
|
||||
"json-loader": "^0.5.3",
|
||||
"react": "^0.13.0",
|
||||
"react-hot-loader": "^1.3.0",
|
||||
"webpack": "^1.9.6",
|
||||
"webpack-dev-server": "^1.8.2"
|
||||
}
|
||||
}
|
|
@ -1,16 +0,0 @@
|
|||
'use strict'
|
||||
var webpack = require('webpack')
|
||||
var WebpackDevServer = require('webpack-dev-server')
|
||||
var config = require('./webpack.config')
|
||||
|
||||
new WebpackDevServer(webpack(config), {
|
||||
publicPath: config.output.publicPath,
|
||||
hot: true,
|
||||
historyApiFallback: true
|
||||
}).listen(3000, 'localhost', function (err, result) {
|
||||
if (err) {
|
||||
console.log(err)
|
||||
}
|
||||
|
||||
console.log('Listening at localhost:3000')
|
||||
})
|
|
@ -1,63 +0,0 @@
|
|||
'use strict'
|
||||
const React = require('react')
|
||||
const ipfsAPI = require('ipfs-api')
|
||||
|
||||
const ipfs = ipfsAPI('localhost', '5001')
|
||||
const stringToUse = 'hello world from webpacked IPFS'
|
||||
|
||||
class App extends React.Component {
|
||||
constructor (props) {
|
||||
super(props)
|
||||
this.state = {
|
||||
id: null,
|
||||
version: null,
|
||||
protocol_version: null,
|
||||
added_file_hash: null,
|
||||
added_file_contents: null
|
||||
}
|
||||
}
|
||||
componentDidMount () {
|
||||
ipfs.id((err, res) => {
|
||||
if (err) throw err
|
||||
this.setState({
|
||||
id: res.id,
|
||||
version: res.agentVersion,
|
||||
protocol_version: res.protocolVersion
|
||||
})
|
||||
})
|
||||
ipfs.add([Buffer.from(stringToUse)], (err, res) => {
|
||||
if (err) throw err
|
||||
const hash = res[0].hash
|
||||
this.setState({added_file_hash: hash})
|
||||
ipfs.cat(hash, (err, res) => {
|
||||
if (err) throw err
|
||||
let data = ''
|
||||
res.on('data', (d) => {
|
||||
data = data + d
|
||||
})
|
||||
res.on('end', () => {
|
||||
this.setState({added_file_contents: data})
|
||||
})
|
||||
})
|
||||
})
|
||||
}
|
||||
render () {
|
||||
return <div style={{textAlign: 'center'}}>
|
||||
<h1>Everything is working!</h1>
|
||||
<p>Your ID is <strong>{this.state.id}</strong></p>
|
||||
<p>Your IPFS version is <strong>{this.state.version}</strong></p>
|
||||
<p>Your IPFS protocol version is <strong>{this.state.protocol_version}</strong></p>
|
||||
<div>
|
||||
<div>
|
||||
Added a file! <br />
|
||||
{this.state.added_file_hash}
|
||||
</div>
|
||||
<div>
|
||||
Contents of this file: <br />
|
||||
{this.state.added_file_contents}
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
}
|
||||
}
|
||||
module.exports = App
|
|
@ -1,5 +0,0 @@
|
|||
'use strict'
|
||||
const React = require('react')
|
||||
const App = require('./App')
|
||||
|
||||
React.render(<App />, document.getElementById('root'))
|
|
@ -1,33 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
var path = require('path')
|
||||
var webpack = require('webpack')
|
||||
|
||||
module.exports = {
|
||||
devtool: 'eval',
|
||||
entry: [
|
||||
'webpack-dev-server/client?http://localhost:3000',
|
||||
'webpack/hot/only-dev-server',
|
||||
'./src/index'
|
||||
],
|
||||
output: {
|
||||
path: path.join(__dirname, 'dist'),
|
||||
filename: 'bundle.js',
|
||||
publicPath: '/static/'
|
||||
},
|
||||
plugins: [
|
||||
new webpack.HotModuleReplacementPlugin()
|
||||
],
|
||||
module: {
|
||||
loaders: [{
|
||||
test: /\.js$/,
|
||||
loaders: ['react-hot', 'babel'],
|
||||
include: path.join(__dirname, 'src')
|
||||
}, { test: /\.json$/, loader: 'json-loader' }]
|
||||
},
|
||||
node: {
|
||||
fs: 'empty',
|
||||
net: 'empty',
|
||||
tls: 'empty'
|
||||
}
|
||||
}
|
|
@ -1,14 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
const ipfs = require('../../src')('localhost', 5001)
|
||||
|
||||
ipfs.files.ls('/folder1', function (err, res) {
|
||||
if (err) {
|
||||
return console.log('got an error', err)
|
||||
}
|
||||
if (res.readable) {
|
||||
res.pipe(process.stdout)
|
||||
} else {
|
||||
console.log(res)
|
||||
}
|
||||
})
|
|
@ -1,20 +0,0 @@
|
|||
# JS IPFS API - Example Browser - Name
|
||||
|
||||
## Setup
|
||||
|
||||
Install [go-ipfs](https://ipfs.io/docs/install/) and start the daemon.
|
||||
|
||||
Configure CORS as suggested by the README https://github.com/ipfs/js-ipfs-api#cors
|
||||
|
||||
```bash
|
||||
> ipfs daemon
|
||||
```
|
||||
|
||||
then in this folder run
|
||||
|
||||
```bash
|
||||
> npm install
|
||||
> npm start
|
||||
```
|
||||
|
||||
and open your browser at `http://localhost:8888`.
|
|
@ -1,64 +0,0 @@
|
|||
<!doctype html>
|
||||
<html>
|
||||
<head>
|
||||
<meta charset="utf-8" />
|
||||
<title>JS IPFS API name example</title>
|
||||
<style>
|
||||
.hidden {
|
||||
opacity: 0;
|
||||
}
|
||||
|
||||
form {
|
||||
padding-bottom: 1em;
|
||||
}
|
||||
</style>
|
||||
</head>
|
||||
|
||||
<body>
|
||||
<h1>js-ipfs-api</h1>
|
||||
<h2><code>name.publish()</code> and <code>name.resolve()</code></h2>
|
||||
<p id="status" style="color: white; padding: .5em; background: blue">
|
||||
initializing...
|
||||
</p>
|
||||
|
||||
<form id="publish-text">
|
||||
<h3>Add a new file to IPFS and publish it.</h3>
|
||||
<textarea name="text" placeholder="hello, world" required></textarea>
|
||||
<button type="submit" disabled>Publish</button>
|
||||
</form>
|
||||
|
||||
<form id="publish-path">
|
||||
<h3>Publish an existing file or directory from IPFS.</h3>
|
||||
<input name="path" type="text" placeholder="IPFS path" required/>
|
||||
<button type="submit" disabled>Publish</button>
|
||||
</form>
|
||||
|
||||
<div class="results--publish hidden">
|
||||
<p>
|
||||
Published at <code id="publish-result"></code>
|
||||
</p>
|
||||
<p>
|
||||
<a id="publish-gateway-link">Open with HTTP gateway</a>
|
||||
</p>
|
||||
</div>
|
||||
|
||||
<hr />
|
||||
|
||||
<form id="resolve-name">
|
||||
<h3>Resolve an IPNS name</h3>
|
||||
<input name="name" type="text" placeholder="IPNS name" required />
|
||||
<button type="submit" disabled>Resolve</button>
|
||||
</form>
|
||||
<div class="results--resolve hidden">
|
||||
<p>
|
||||
Resolves to <code id="resolve-result"></code>
|
||||
</p>
|
||||
<p>
|
||||
<a id="resolve-gateway-link">Open with HTTP gateway</a>
|
||||
</p>
|
||||
</div>
|
||||
|
||||
<script src="https://unpkg.com/ipfs-api/dist/index.js"></script>
|
||||
<script src="bundle.js"></script>
|
||||
</body>
|
||||
</html>
|
|
@ -1,129 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
const ipfs = window.IpfsApi('/ip4/127.0.0.1/tcp/5001')
|
||||
|
||||
const DOM = {
|
||||
status: document.getElementById('status'),
|
||||
buttons: document.getElementsByTagName('button'),
|
||||
publishNew: document.forms[0],
|
||||
publishPath: document.forms[1],
|
||||
resolveName: document.forms[2],
|
||||
publishResultsDiv: document.querySelector('.results--publish'),
|
||||
resolveResultsDiv: document.querySelector('.results--resolve'),
|
||||
publishResult: document.getElementById('publish-result'),
|
||||
resolveResult: document.getElementById('resolve-result'),
|
||||
publishGatewayLink: document.getElementById('publish-gateway-link'),
|
||||
resolveGatewayLink: document.getElementById('resolve-gateway-link')
|
||||
}
|
||||
|
||||
const COLORS = {
|
||||
active: 'blue',
|
||||
success: 'green',
|
||||
error: 'red'
|
||||
}
|
||||
|
||||
const IPFS_DOMAIN = 'https://ipfs.io'
|
||||
|
||||
const showStatus = (text, bg) => {
|
||||
DOM.status.innerText = text
|
||||
DOM.status.style.background = bg
|
||||
}
|
||||
|
||||
const enableForms = () => {
|
||||
for (let btn of DOM.buttons) {
|
||||
btn.disabled = false
|
||||
}
|
||||
}
|
||||
|
||||
const init = () => {
|
||||
ipfs.id()
|
||||
.then(res => {
|
||||
showStatus(`daemon active\nid: ${res.ID}`, COLORS.success)
|
||||
enableForms()
|
||||
})
|
||||
.catch(err => {
|
||||
showStatus('daemon inactive', COLORS.error)
|
||||
console.error(err)
|
||||
})
|
||||
}
|
||||
|
||||
// Adds a new file to IPFS and publish it
|
||||
const addAndPublish = (e) => {
|
||||
e.preventDefault()
|
||||
|
||||
let input = e.target.elements['text']
|
||||
let buffer = Buffer.from(input.value)
|
||||
|
||||
showStatus('adding to IPFS...', COLORS.active)
|
||||
|
||||
ipfs.add(buffer)
|
||||
.then(res => {
|
||||
showStatus(`success!`, COLORS.success)
|
||||
|
||||
publish(res[0].path)
|
||||
|
||||
input.value = ''
|
||||
})
|
||||
.catch(err => {
|
||||
showStatus('failed to add the data', COLORS.error)
|
||||
console.error(err)
|
||||
})
|
||||
}
|
||||
|
||||
// Publishes an IPFS file or directory under your node's identity
|
||||
const publish = (path) => {
|
||||
showStatus(`publishing...`, COLORS.active)
|
||||
DOM.publishResultsDiv.classList.add('hidden')
|
||||
|
||||
ipfs.name.publish(path)
|
||||
.then(res => {
|
||||
const name = res.Name
|
||||
showStatus('success!', COLORS.success)
|
||||
DOM.publishResultsDiv.classList.remove('hidden')
|
||||
DOM.publishResult.innerText = `/ipns/${name}`
|
||||
DOM.publishGatewayLink.href = `${IPFS_DOMAIN}/ipns/${name}`
|
||||
})
|
||||
.catch(err => {
|
||||
showStatus(`error publishing ${path}`, COLORS.error)
|
||||
console.error(err)
|
||||
})
|
||||
}
|
||||
|
||||
// Resolves an IPNS name
|
||||
const resolve = (name) => {
|
||||
showStatus(`resolving...`, COLORS.active)
|
||||
DOM.resolveResultsDiv.classList.add('hidden')
|
||||
|
||||
ipfs.name.resolve(name)
|
||||
.then(res => {
|
||||
const path = res.Path
|
||||
|
||||
showStatus('success!', COLORS.success)
|
||||
DOM.resolveResultsDiv.classList.remove('hidden')
|
||||
DOM.resolveResult.innerText = path
|
||||
DOM.resolveGatewayLink.href = `${IPFS_DOMAIN}${path}`
|
||||
})
|
||||
.catch(err => {
|
||||
showStatus(`error resolving ${name}`, COLORS.error)
|
||||
console.error(err)
|
||||
})
|
||||
}
|
||||
|
||||
// Event listeners
|
||||
DOM.publishNew.onsubmit = addAndPublish
|
||||
|
||||
DOM.publishPath.onsubmit = (e) => {
|
||||
e.preventDefault()
|
||||
let input = e.target.elements['path']
|
||||
publish(input.value)
|
||||
input.value = ''
|
||||
}
|
||||
|
||||
DOM.resolveName.onsubmit = (e) => {
|
||||
e.preventDefault()
|
||||
let input = e.target.elements['name']
|
||||
resolve(input.value)
|
||||
input.value = ''
|
||||
}
|
||||
|
||||
init()
|
|
@ -1,15 +0,0 @@
|
|||
{
|
||||
"name": "js-ipfs-api-example-name-publish-resolve",
|
||||
"version": "1.0.0",
|
||||
"description": "",
|
||||
"main": "index.js",
|
||||
"scripts": {
|
||||
"start": "browserify index.js > bundle.js && http-server -a 127.0.0.1 -p 8888"
|
||||
},
|
||||
"author": "Tara Vancil <tbvanc@gmail.com>",
|
||||
"license": "MIT",
|
||||
"devDependencies": {
|
||||
"browserify": "^14.4.0",
|
||||
"http-server": "^0.10.0"
|
||||
}
|
||||
}
|
|
@ -1,28 +0,0 @@
|
|||
name, bundled (KBs), minified (KBs)
|
||||
IPFS, 1412.20, 573.44
|
||||
add, 591.17, 198.23
|
||||
bitswap, 590.14, 197.96
|
||||
block, 630.50, 216.31
|
||||
bootstrap, 590.97, 198.22
|
||||
cat, 630.78, 216.41
|
||||
commands, 589.22, 197.59
|
||||
config, 592.21, 198.93
|
||||
dht, 593.86, 199.24
|
||||
diag, 590.31, 198.00
|
||||
files, 669.07, 235.88
|
||||
get, 661.57, 233.16
|
||||
id, 589.65, 197.78
|
||||
key, 589.93, 197.86
|
||||
log, 590.74, 198.20
|
||||
ls, 589.35, 197.63
|
||||
mount, 589.53, 197.69
|
||||
name, 589.97, 197.88
|
||||
object, 833.17, 307.73
|
||||
pin, 590.86, 198.22
|
||||
ping, 589.94, 197.73
|
||||
pubsub, 595.31, 199.76
|
||||
refs, 589.74, 197.77
|
||||
repo, 589.91, 197.85
|
||||
swarm, 1239.42, 498.59
|
||||
update, 589.79, 197.79
|
||||
version, 589.55, 197.71
|
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../src')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/add')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/bitswap')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/block')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/bootstrap')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/cat')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/commands')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/config')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/dht')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/diag')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/files')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/get')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/id')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/key')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/log')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/ls')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/mount')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/name')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/object')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/pin')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/ping')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/pubsub')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/refs')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/repo')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/swarm')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/update')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,3 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
require('../../../../src/version')('/ip4/127.0.0.1/tcp/5001')
|
|
@ -1,17 +0,0 @@
|
|||
{
|
||||
"name": "sub-module",
|
||||
"version": "1.0.0",
|
||||
"description": "",
|
||||
"scripts": {
|
||||
"test": "echo \"Error: no test specified\" && exit 1"
|
||||
},
|
||||
"author": "Nuno Nogueira",
|
||||
"license": "MIT",
|
||||
"devDependencies": {
|
||||
"babel-core": "^6.25.0",
|
||||
"babel-loader": "^7.1.0",
|
||||
"babel-preset-env": "^1.5.2",
|
||||
"babili": "^0.1.4",
|
||||
"webpack": "^3.0.0"
|
||||
}
|
||||
}
|
|
@ -1,26 +0,0 @@
|
|||
#!/bin/sh
|
||||
set -e
|
||||
|
||||
modules=($(ls modules/))
|
||||
|
||||
echo "name, bundled (KBs), minified (KBs)"
|
||||
|
||||
# Full IPFS module
|
||||
webpack --display none --config webpack.config.js complete-module.js complete-bundle.js
|
||||
babili complete-bundle.js -o complete-bundle-minified.js
|
||||
|
||||
ipfsBundleSize=($(wc -c < complete-bundle.js | awk '{b=$1/1024; printf "%.2f\n", b}' | sed 's/,/./g'))
|
||||
ipfsMinSize=($(wc -c < complete-bundle-minified.js | awk '{b=$1/1024; printf "%.2f\n", b}' | sed 's/,/./g'))
|
||||
|
||||
echo IPFS, $ipfsBundleSize, $ipfsMinSize
|
||||
|
||||
for module in "${modules[@]}"
|
||||
do
|
||||
moduledir="modules/$module"
|
||||
webpack --display none --config webpack.config.js $moduledir/$module.js $moduledir/bundle.js
|
||||
babili $moduledir/bundle.js -o $moduledir/bundle-minified.js
|
||||
|
||||
bundlesize=($(wc -c < $moduledir/bundle.js | awk '{b=$1/1024; printf "%.2f\n", b}' | sed 's/,/./g'))
|
||||
minsize=($(wc -c < $moduledir/bundle-minified.js | awk '{b=$1/1024; printf "%.2f\n", b}' | sed 's/,/./g'))
|
||||
echo $module, $bundlesize, $minsize
|
||||
done
|
|
@ -1,15 +0,0 @@
|
|||
'use strict'
|
||||
|
||||
module.exports = {
|
||||
module: {
|
||||
loaders: [{
|
||||
test: /\.js$/,
|
||||
loaders: ['babel-loader']
|
||||
}]
|
||||
},
|
||||
node: {
|
||||
fs: 'empty',
|
||||
net: 'empty',
|
||||
tls: 'empty'
|
||||
}
|
||||
}
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in New Issue